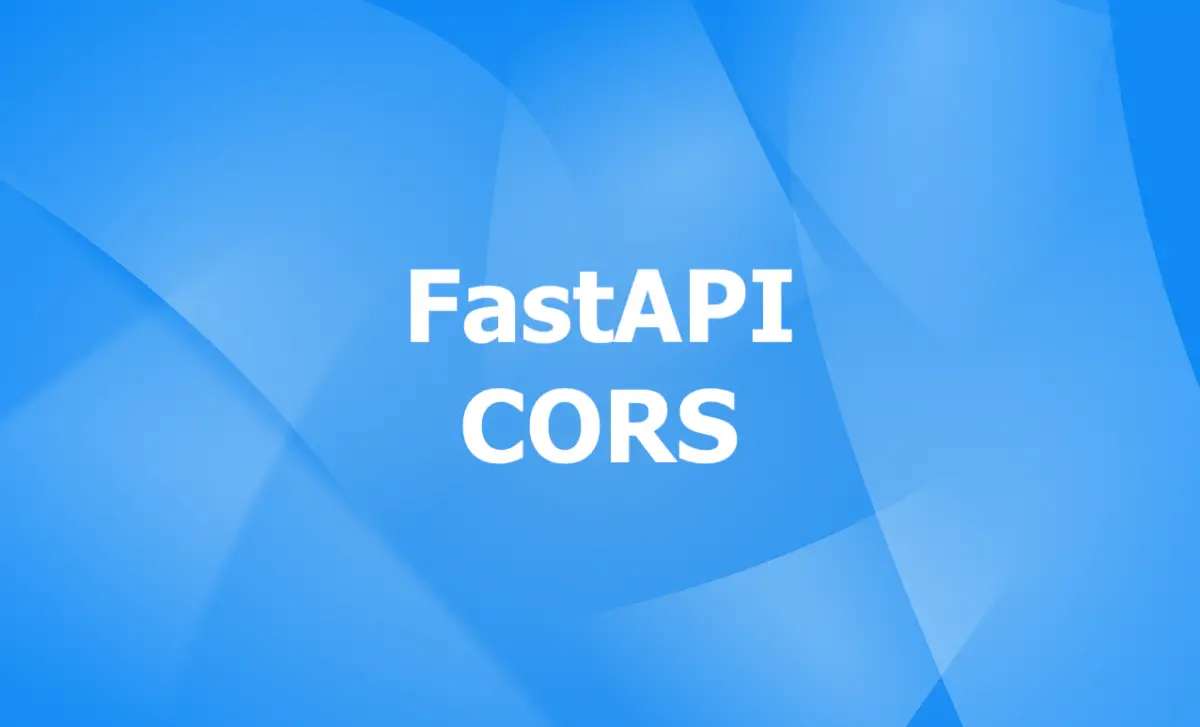
Introduction
By default, a FastAPI application does not allow requests from different origins than its own domain.
Allowing requests from different origins in FastAPI is a common scenario when you have a frontend application that communicates with a backend API, and they are hosted on different domains or ports. This is known as CORS (Cross-Origin Resource Sharing), and it requires some configuration to work properly.
This practical article will show you how to enable CORS in FastAPI. Let’s roll up our sleeves and go ahead!
Showcase: Our public RESTful API, api.slingacademy.com, accepts incoming requests from any domain including localhost. You can try this API endpoint (sample users) or this one (dummy blog posts).
Using CORSMiddleware
The best way to enable CORS is to use the CORSMiddleware module. This module allows you to specify a list of allowed origins, methods, headers, and credentials that your API will accept from cross-origin requests. You can also use wildcards (*) to allow all origins, methods, or headers, but this is not recommended for security reasons.
In general, the steps to setup CORS look as follows:
- Import CORSMiddleware from fastapi.middleware.cors
- Create a list of allowed origins (as strings)
- Add CORSMiddleware as a middleware to your FastAPI application using app.add_middleware()
- Pass the list of allowed origins and any other parameters you want to configure (such as allow_credentials, allow_methods, or allow_headers) to the middleware.
Let’s see the following examples for more clarity.
Allowing Requests from a List of Domains
In this example, we restrict FastAPI to only accept incoming requests from certain origins, with a limited number of methods and headers.
The code:
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
# Allow these origins to access the API
origins = [
"http://localhost",
"https://localhost:3000",
"https://tools.slingacademy.com",
"https://www.slingacademy.com",
]
# Allow these methods to be used
methods = ["GET", "POST", "PUT", "DELETE"]
# Only these headers are allowed
headers = ["Content-Type", "Authorization"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=methods,
allow_headers=headers,
)
@app.get("/")
async def main():
return {"message": "Welcome to Sling Academy"}
Allowing Requests from Any Domains
In some cases, especially when developing and testing software, you might want to remove any restrictions from your API. The code below demonstrates how to do so:
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
app.add_middleware(
CORSMiddleware,
allow_origins=["*"],
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
@app.get("/")
async def main():
return {"message": "Welcome to Sling Academy"}
Conclusion
You’ve learned how to handle CORS in FastAPI and examined a few examples of applying that knowledge in practice.
If you are a frontend developer, a mobile developer, or a data scientist, you can try some of our mock API endpoints made with FastAPI for practicing and prototyping:
- Sample Products – Mock REST API for Practice
- Sample Photos – Free Fake REST API for Practice
- Sample Users – Free Fake API for Practicing & Prototyping
- Marketing Campaigns Sample Data (CSV, JSON, XLSX, XML)
Happy coding & have a nice day!