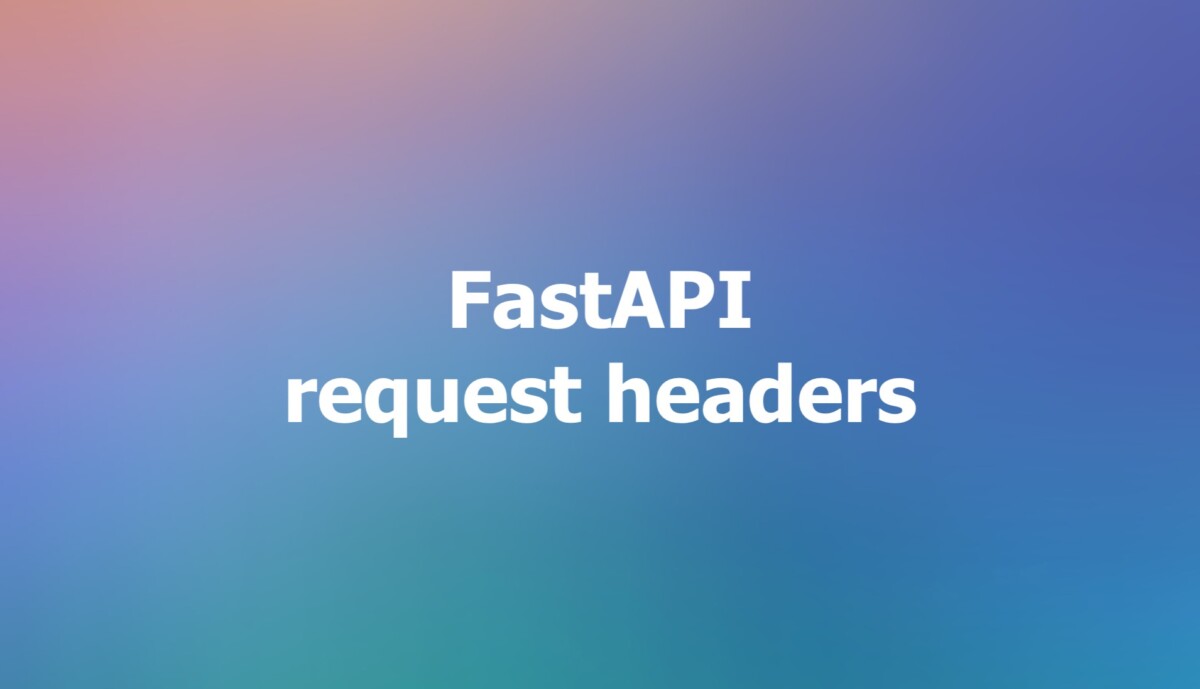
This concise, practical article will walk you through a couple of different ways to extract headers from an incoming request in FastAPI.
What are HTTP headers?
Headers are part of HTTP messages that pass additional information and metadata about the request or response. They consist of case-insensitive name-value pairs separated by a colon. For instance, a request message can use headers to indicate its preferred media formats, while a response can use headers to indicate the media format of the returned body. Headers can be grouped into different categories according to their contexts and how they are handled by proxies and caches.
Some common headers are:
- User-Agent: identifies the client software that is making the request.
- Referer: indicates the URL of the previous web page from which a link was followed.
- Authorization: contains the credentials to authenticate a user-agent with a server.
- Content-Type: indicates the MIME type of the body of the request or response.
- Content-Length: indicates the size of the body in bytes.
Extracting request headers in FastAPI
Using the Request object directly
The easiest way to get headers from incoming requests is to use the Request object directly. You can import it from fastapi and declare it as a parameter in your path operation function. FastAPI will pass the request object to that parameter. Then you can access the headers attribute of the request object, which is a dictionary of header names and values.
The general syntax is:
some_header = request.headers.get("header name")
Real-world example:
import fastapi
from fastapi import Request
app = fastapi.FastAPI()
@app.get("/")
def home_route(request: Request):
user_agent = request.headers.get("user-agent")
authorization = request.headers.get("authorization")
referer = request.headers.get("referer")
print(f"User-Agent: {user_agent}")
print(f"Authorization: {authorization}")
print(f"Referer: {referer}")
return {
"message": "Welcome to Sling Academy"
}
If a header doesn’t exist, the result will be None.
Using Header parameters
Another way is to use Header parameters in your path operation function, which will automatically convert the header name from underscore to hyphen and validate the type.
Example:
from fastapi import FastAPI, Header
app = FastAPI()
@app.get("/")
async def home_route(
user_agent: str = Header(None),
authorization: str = Header(None),
referer: str = Header(None)):
print("user_agent: ", user_agent)
print("authorization: ", authorization)
print("referer: ", referer)
return {
"message": "Welcome to Sling Academy",
}
Both this approach and the previous one are concise and convenient. Choose from them the one you like to implement in your project.