There might be cases where you want to deploy a complex web app that consists of multiple child web apps. Each child web app is developed independently by a team and goes with a subdirectory path like this:
slingacademy.com/blogs/
slingacademy.com/app/
slingacademy.com/shop/
slingacademy.com/forums/
To access a child web app, you will type the root domain + the subdirectory path. In Next.js, you can set the subdirectory path with the basePath option in the next.config.js file (the default value is an empty string):
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
// add this line
basePath: '/subfolder'
}
module.exports = nextConfig
The basePath will be automatically added to every link in your app. It works the same in both development and production. If you don’t clear on what I mean, let’s see the complete example below.
Table of Contents
Example
App Preview
The tiny project we’re going to build uses /sling-academy as the base path. On localhost, the home page is http://localhost:3000/sling-academy.
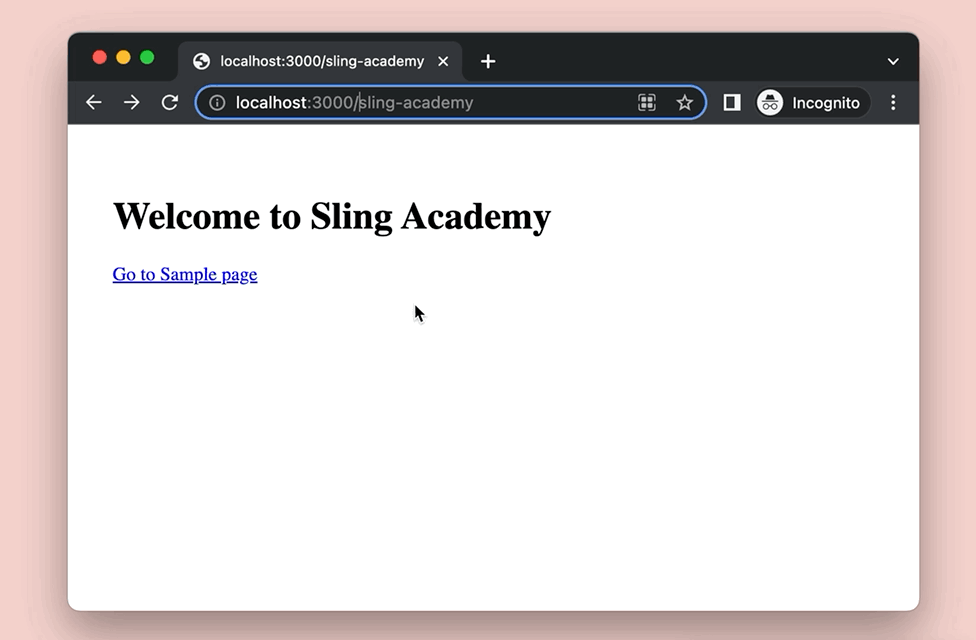
The Steps
1. Create a brand new Next.js app:
npx create-next-app example
2. Modify the next.config.js file as follows:
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
// add this line
basePath: '/sling-academy'
}
module.exports = nextConfig
3. Replace the default code in pages/index.js with the following:
// pages/index.js
import Link from 'next/link';
export default function Home(props) {
return (
<div style={{ padding: 30 }}>
<h1>Welcome to Sling Academy</h1>
<Link
href='/sample-page'
style={{ color: 'blue', textDecoration: 'underline' }}
>
Go to Sample page
</Link>
</div>
);
}
4. In your pages folder, create a new file called sample-page.js, then add the code below into it:
// pages/sample-page.js
export default function Sample(){
return <div style={{padding: 30}}>
<h1>Sample Page</h1>
</div>
}
5. Boot your app up by running this:
npm run dev
Open a web browser, then go to the following address to check your work:
http://localhost:3000/sling-academy
My screenshot:
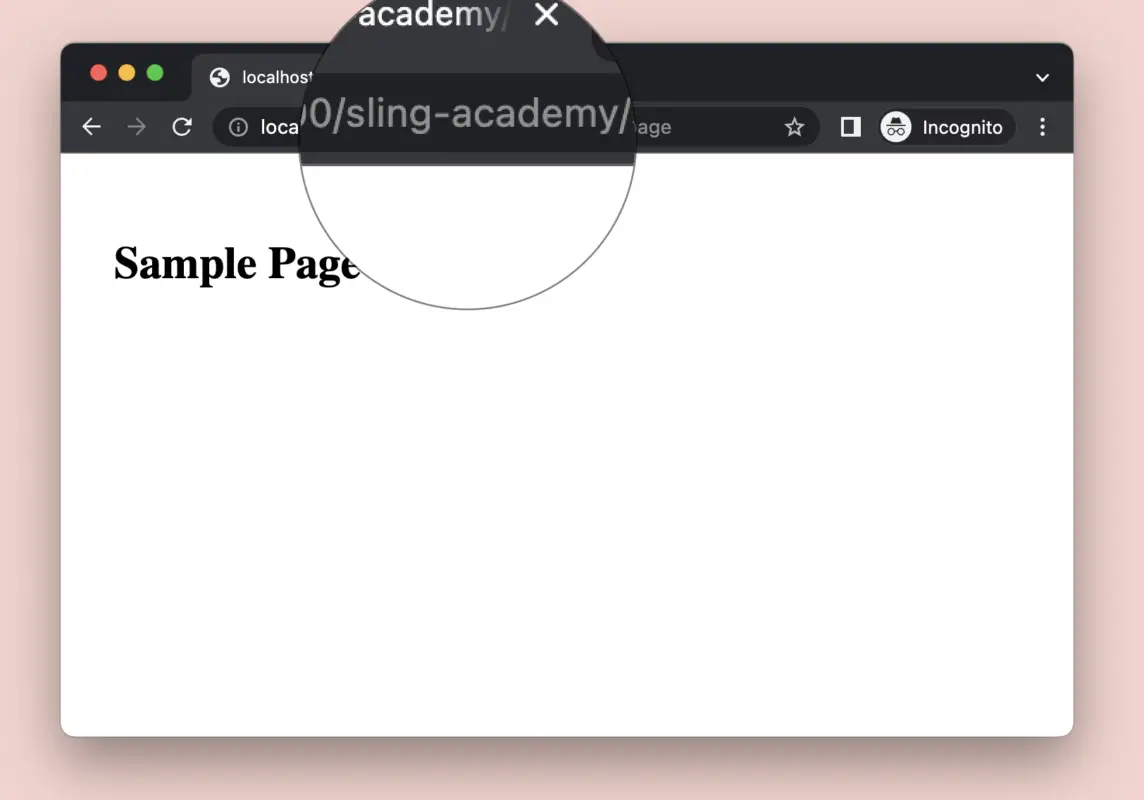
Good luck!