Next.js is a React framework for building server-side rendered (SSR) or static web applications with pre-renders pages at build-time (SSG). It enables developers to build fast, optimized, and scalable web applications with minimal effort.
The 404 error means the requested page or file doesn’t exist on the server. This type of error can happen due to a variety of reasons, ranging from incorrect or broken links to incorrect server configuration.
Why You Should Create a Custom 404 Page
Next.js provides a premade 404 Not Found page, but it’s too plain and looks like a dead-end place with no way to navigate to other pages on your web app (except using the browser’s back/forward buttons or the address bar). When a user reaches here, he or she will very likely leave your web app. That’s a terrible waste and is a strong reason that propels us to add a custom 404 page.
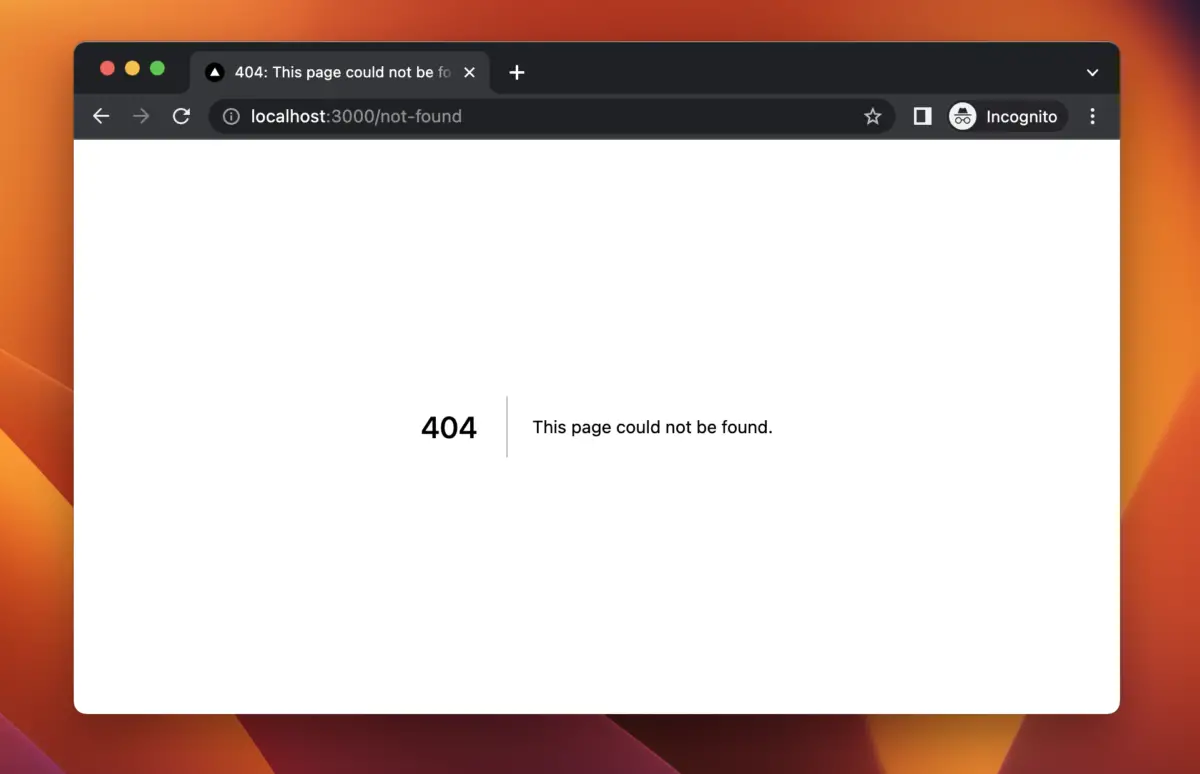
A custom 404 page should be creative, informative, and helpful. It should have links and maybe a search box to help users find what they are looking for. In this article, you will learn how to set up a custom 404 page in Next.js and style it. Without any boring talking, let’s get started!
Custom 404 Page and App Router (new)
If you desire to use modern Next.js (version 13 or beyond) with the /app
directory, this section is the way to go. In case you want to work with the traditional /pages
directory, skip this section and go to the next one.
The First Step
In this example, we’ll use the latest version of Next.js and write code in TypeScript. We’ll also use Tailwind CSS to style things.
You can initialize a new project by executing this command:
npx create-next-app@latest example
You’ll be asked to use TypeScript and Tailwind CSS. Just select Yes
.
Next.js provides some default styles in the app/global.css
file. They are not often useful and can make our lives more difficult. Therefore, you should remove them and keep your app/global.css
file clean as follows:
/* app/global.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
From here, we are good to go. No third-party libraries or extra setups are required.
Setting Up Your Custom 404 Page
To create a custom 404 not found page with App Router, just create a new file named not-found.tsx
to the /app
directory and add some starter code to it:
export default function NotFound() {
return (
<>
<h1>404 - Page Not Found</h1>
</>
);
}
Next.js will automatically send a 404
HTTP status, so you don’t have to worry about it.
At this point, when you try to access a non-existent route, you will see an unattractive page as follows:
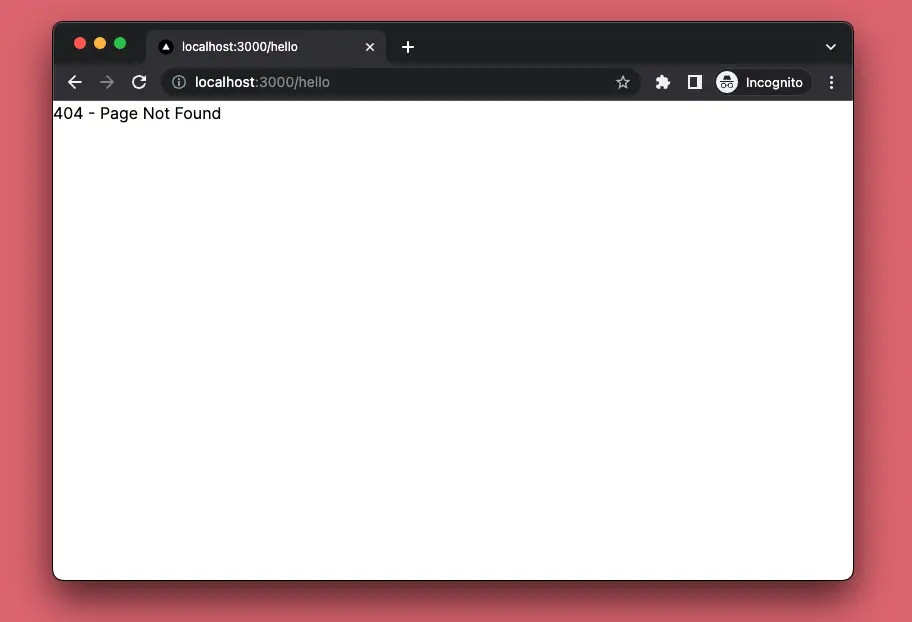
It tells us that our custom 404 page works. However, it’s still not so useful and uninformative. We have to do something to make it better.
Add Useful Things to the Custom 404 Page
You can add a search box or a list of popular pages to the 404 page to help users find what they want. You can also add a link to the homepage or contact page to make it easier for users to navigate to their desired destinations.
Update your app/not-found.tsx
file as follows:
// app/not-found.tsx
import Link from 'next/link';
export default function NotFound() {
return (
<>
<div>
<h1>404 - Page Not Found</h1>
<p>Sorry, there is nothing to see here</p>
<p>
Use the search box or the links below to explore our amazing
application
</p>
<input type='search' placeholder='Just a dummy search box...' />
<div>
<Link href='/'>Homepage</Link>
<Link href='/latest'>Latest Products</Link>
<Link href='/contact'>Contact Us</Link>
</div>
</div>
</>
);
}
At the moment, since we haven’t styled the page yet, it still looks as chaotic as a typical developer’s room (I am an untidy guy):
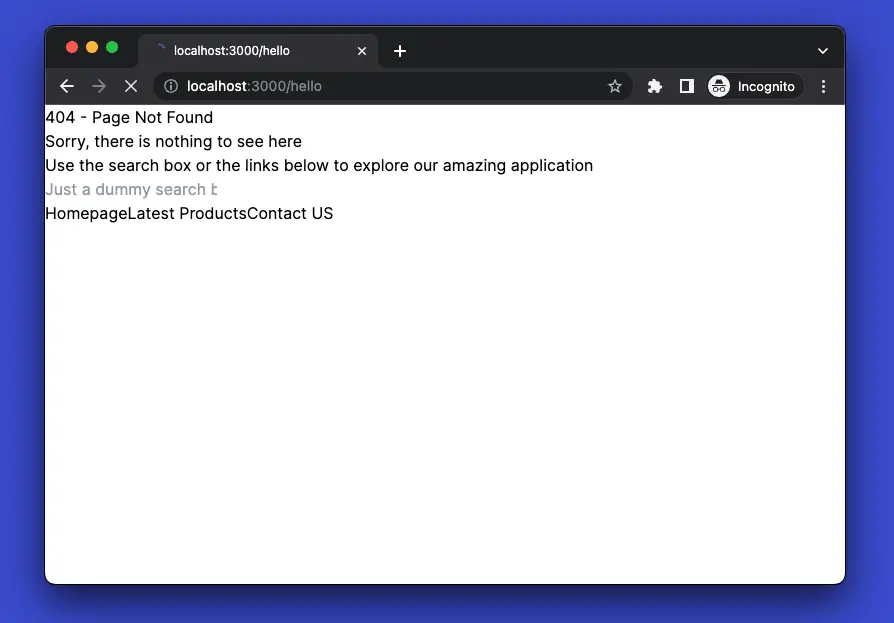
We’ll use Tailwind CSS utility classes to transform it into a professional thing. Update your app/not-found.tsx
file like this:
// app/not-found.tsx
import Link from 'next/link';
export default function NotFound() {
return (
<>
<div className='w-4/5 mx-auto mt-20 flex flex-col justify-center items-center space-y-4'>
<h1 className='text-4xl font-semibold'>404 - Page Not Found</h1>
<h4 className='py-10 text-lg text-red-500 font-semibold capitalize'>
The Nothingness and Emptiness is here...
</h4>
<p>
Use the search box or the links below to explore our amazing
application
</p>
<input
className='w-4/5 px-6 py-3 border border-gray-400 rounded-full'
type='search'
placeholder='Just a dummy search box...'
/>
<div className='space-x-4'>
<Link
className='underline text-blue-600 hover:text-red-500 duration-300'
href='/'
>
Homepage
</Link>
<Link
className='underline text-blue-600 hover:text-red-500 duration-300'
href='/latest'
>
Latest Products
</Link>
<Link
className='underline text-blue-600 hover:text-red-500 duration-300'
href='/contact'
>
Contact Us
</Link>
</div>
</div>
</>
);
}
And here’s the final result:
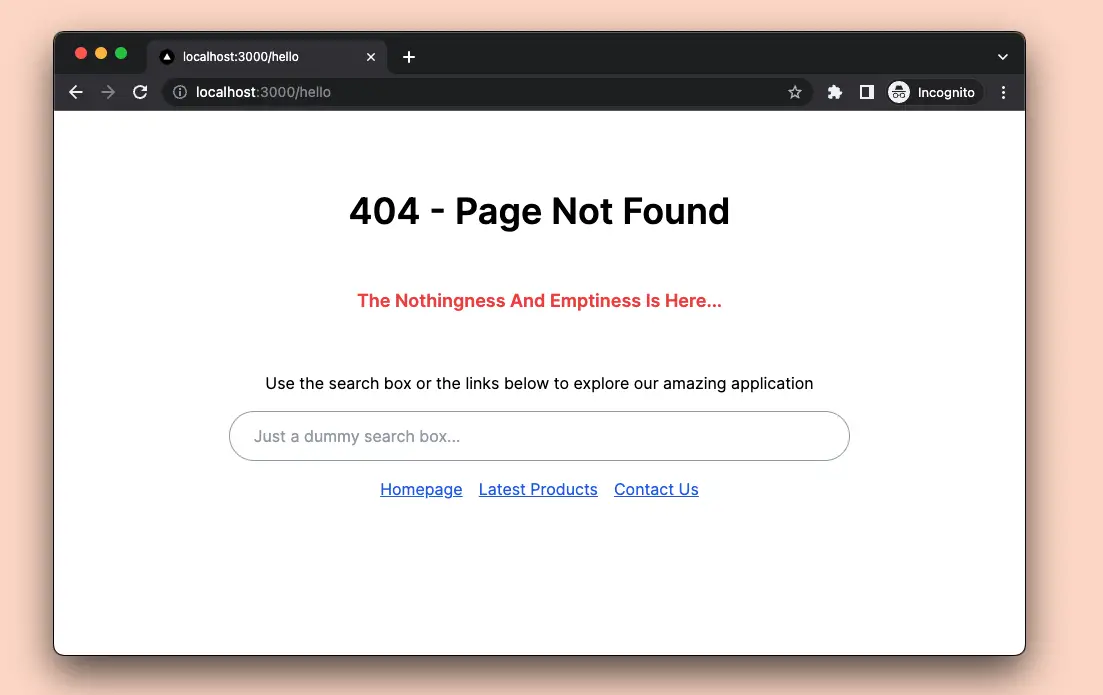
Do you think this Not Found page looks pretty cool and helpful?
Custom 404 Page and Pages Router (legacy)
Setting Up Your Custom 404 Page
To set up a custom 404 page in Next.js, you must create a 404.js
file in the pages
directory. This file will be used as the default page when a user accesses a page that doesn’t exist.
Add the following code to your 404.js
:
// pages/404.js
const NotFoundPage = () => {
return <></>
}
export default NotFoundPage
Now try to enter a wrong URL (for example, http://localhost:3000/not-found
) and you will see a blank page like this:
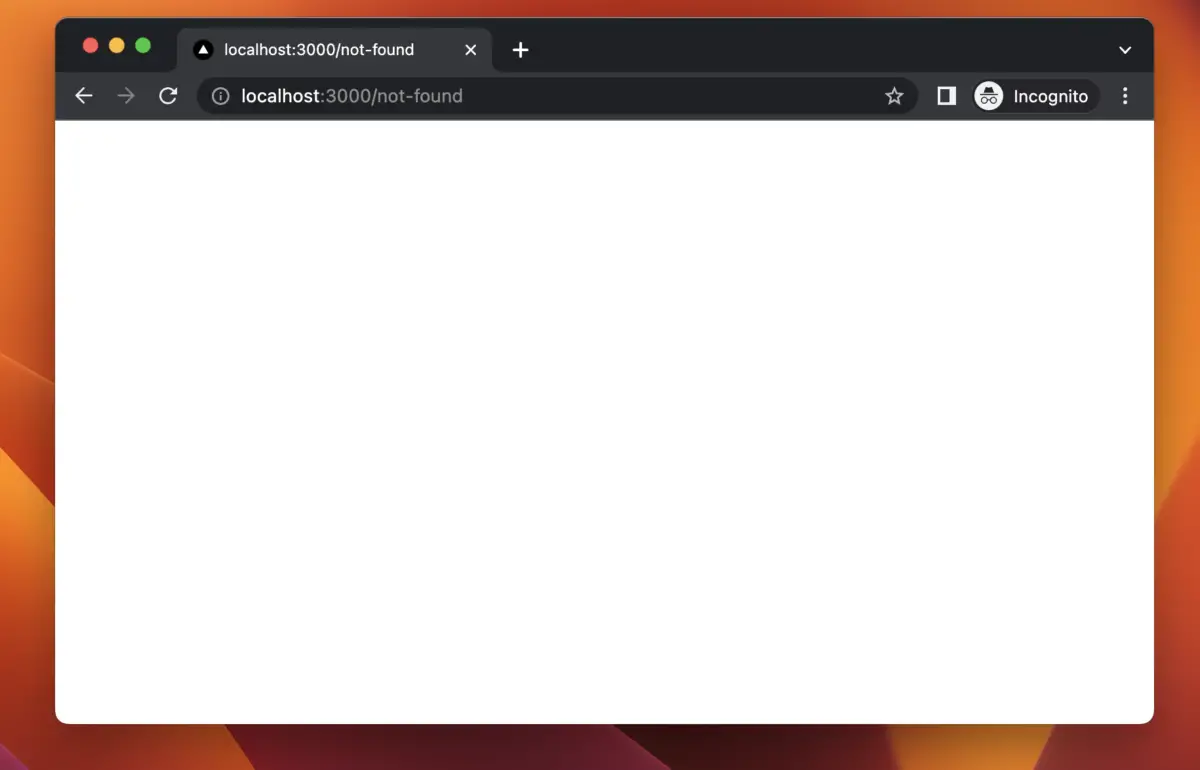
This proves that our 404 page was used instead of the default page. The next step is to make this custom 404 page more useful.
Add Things to Your Custom 404 Page
You can add a search bar or a list of popular pages to the 404 page to help users find what they want. You can also add a link to the homepage or contact page to make it easier for users to navigate to the desired page.
Edit your 404.js
file like this:
// pages/404.js
import Link from 'next/link';
const NotFoundPage = () => {
return (
<>
<div>
<h1>404 - Page Not Found</h1>
<p>Sorry, there is nothing to see here</p>
<p>
Use the search box or the links below to explore our amazing
application
</p>
<input
type='search'
placeholder='Just a dummy search box...'
/>
<div>
<Link href='/'>
Homepage
</Link>
<Link href='/latest'>
Latest Products
</Link>
<Link href='/contact'>
Contact US
</Link>
</div>
</div>
</>
);
};
export default NotFoundPage;
The code above adds a title, some description, a demo search box (just UI), a link to the home page, and some other links that lead to other pages. However, your 404 page still looks like a mess:
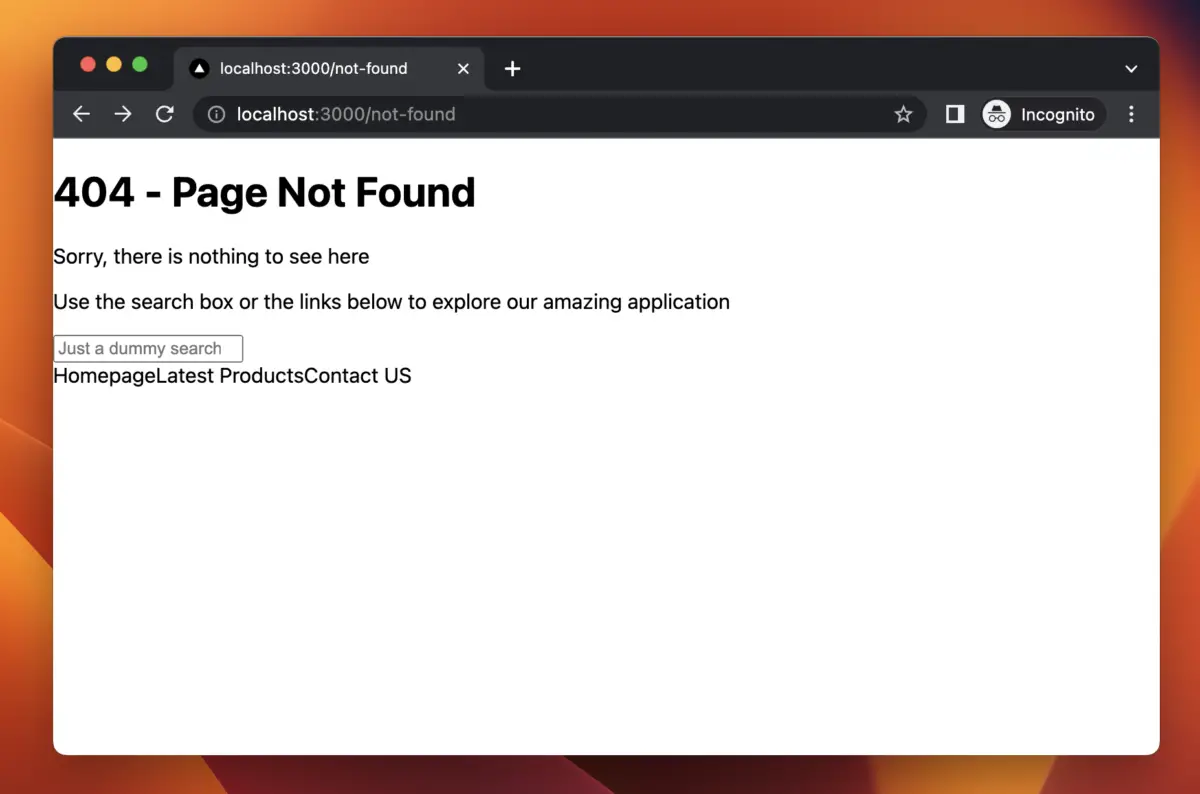
What we have to do next is to polish it with CSS.
Styling Your Custom 404 Page
Once you have created and added error handling to your custom 404 page, it’s time to style it. In the styles
folder, add a new file named 404.module.css
, then add the following to it:
/* styles/404.module.css */
.container {
width: 80vw;
margin: 40px auto;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.searchBox {
width: 80%;
padding: 10px 15px;
border: 1px solid #ccc;
border-radius: 20px;
}
.links {
margin-top: 30px;
}
.link {
margin: 10px;
color: blue;
text-decoration: underline;
}
.link:hover {
color: red;
}
To ensure that the default styles don’t affect our 404 page, you should remove everything in your styles/global.css
file.
Update your 404.js
file like this for the CSS to work:
// pages/404.js
import Link from 'next/link';
import styles from '../styles/404.module.css';
const NotFoundPage = () => {
return (
<>
<div className={styles.container}>
<h1>404 - Page Not Found</h1>
<p>Sorry, there is nothing to see here</p>
<p>
Use the search box or the links below to explore our amazing
application
</p>
<input
type='search'
className={styles.searchBox}
placeholder='Just a dummy search box...'
/>
<div className={styles.links}>
<Link href='/' className={styles.link}>
Homepage
</Link>
<Link href='/latest' className={styles.link}>
Latest Products
</Link>
<Link href='/contact' className={styles.link}>
Contact US
</Link>
</div>
</div>
</>
);
};
export default NotFoundPage;
And here is the final result:
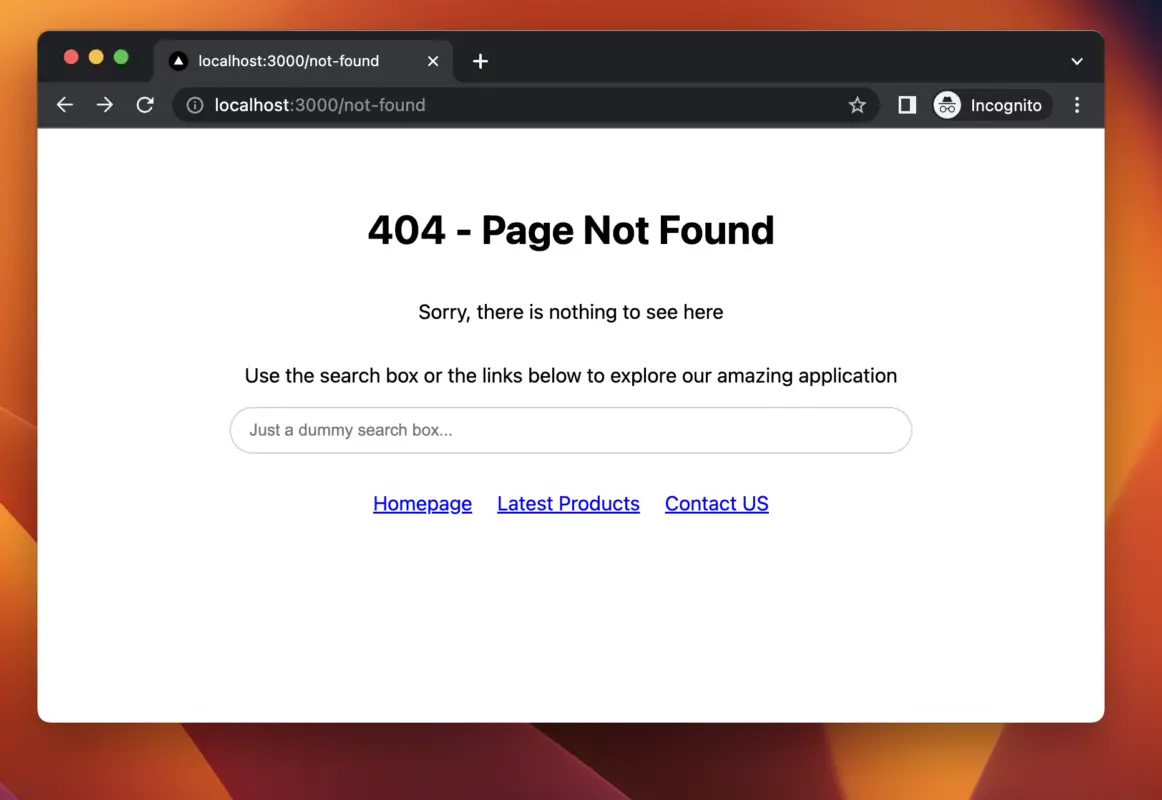
Final Words
Creating a custom 404 page in Next.js is a simple and straightforward process. By following the steps outlined in this article, you can create a custom 404 page that is tailored to your brand and provides a consistent look and feel.
The most important point is to make your 404 page informative and helpful so that we can keep the user stay on our web app longer, and it’s good for most kinds of online businesses.
Good luck and happy coding!