This quick article shows you 2 different approaches to merging 2 or more objects in TypeScript. Without any further ado, let’s get our hands dirty by writing some code.
Using the spread operator
There are some important things you need to keep in mind:
- By using the spread syntax (
...
), the type of the merged object will be inferred automatically. TypeScript will yell at you if you are intent on adding/removing properties to/from the final object (it’s fine if you just want to update the values of the properties). - The order of the objects is important. If objects have duplicate keys, only the values corresponding to those keys of the last object will be retained
Example:
const objectOne = {
name: 'Product One',
price: 100,
description: 'This is product one',
};
const objectTwo = {
weight: 100,
color: 'red',
};
const objectThree = {
name: 'Product Three',
descriptioN: 'Some dummy description',
color: 'blue',
};
// merging objectOne and objectTwo
const a = { ...objectOne, ...objectTwo };
console.log(a);
// merging three objects
// the last object will override the previous objects
const b = { ...objectOne, ...objectTwo, ...objectThree };
console.log(b);
Output:
// console.log(a);
{
name: 'Product One',
price: 100,
description: 'This is product one',
weight: 100,
color: 'red'
}
// console.log(b);
{
name: 'Product Three',
price: 100,
description: 'This is product one',
weight: 100,
color: 'blue',
descriptioN: 'Some dummy description'
}
If you hover your mouse over one of the results, you’ll see it type:
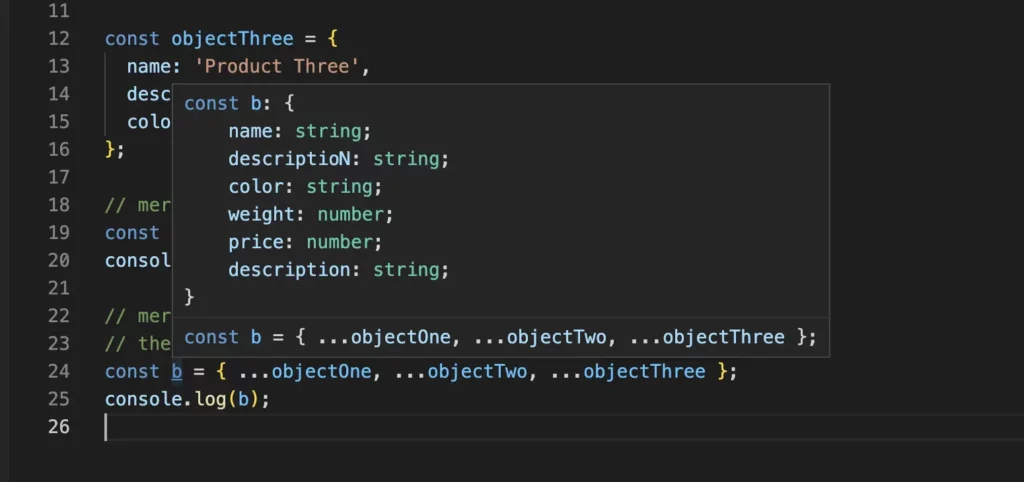
Using the Object.assign() method
This approach works almost the same as the previous one.
Example:
const objectOne = {
name: 'Product One',
price: 100,
description: 'This is product one',
};
const objectTwo = {
weight: 100,
color: 'red',
};
const objectThree = {
name: 'Product Three',
descriptioN: 'Some text',
color: 'blue',
};
// Merge objectOne and objectTwo by using Object.assign()
const resultOne = Object.assign({}, objectOne, objectTwo);
console.log(resultOne);
// Merge objectOne, objectTwo, and objectThree by using Object.assign()
const resultTwo = Object.assign({}, objectOne, objectTwo, objectThree);
console.log(resultTwo);
Output:
// console.log(resultOne);
{
name: 'Product One',
price: 100,
description: 'This is product one',
weight: 100,
color: 'red'
}
// console.log(resultTwo);
{
name: 'Product Three',
price: 100,
description: 'This is product one',
weight: 100,
color: 'blue',
descriptioN: 'Some text'
}
TypeScript is smart and is capable of inferring the types of the results, as you can see in the screenshot below:
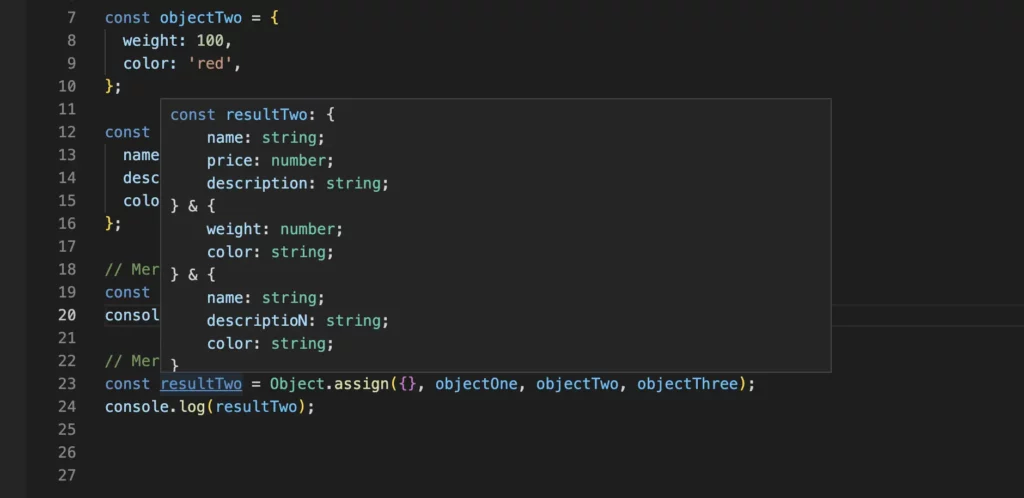
There is a small difference here. The types of the combined objects are Intersection types that are defined using an ampersand &
.