Next.js is a fast-growing React framework that can help us build high-performance hybrid static and server-side rendering web applications. Many projects have been transitioning from traditional React SPAs to Next.js, including giants like Github, IGN, Hulu, etc.
In this article, we’ll explore how to programmatically navigate in a Next.js application by using the useRouter
hook that comes along with the Next.js core. This will be helpful in some scenarios, like changing routes when the user clicks a button, checks a checkbox, chooses an option from a dropdown select menu, after waiting for a countdown timer, etc.
The useRouter hook
If you’re working with server components in the app
directory (that comes with Next.js 13 and newer), you must add the use client
directive at the top of your component to be able to use hooks, as shown below:
"use client";
import { useRouter } from 'next/navigation';
export default function Home() {
const router = useRouter();
console.log(router);
return <></>;
}
If you’re still using the pages
directory as before, the use client
directive is not necessary.
The router
object returned by the useRouter
hook gives us some useful methods for programmatically navigating:
push() method
The push()
method adds a new URL to the history stack:
router.push("/some-destination");
replace() method
This method is similar to the push()
method but will NOT add a new URL into the history stack. That means you cannot go back to the previous route by hitting the back button of your browser.
router.replace("/some-target");
back() and forward() method
You can call the back()
or the forward()
method to go to the previous or the next route, respectively. These methods don’t require any arguments (because their names already tell where to go).
The Complete Example
This example demonstrates how to navigate in Next.js by clicking a button or checking a checkbox. We will use the latest version of Next.js and its app
directory.
Preview
Our sample app has 2 only pages: Home
and Feedback
. There are no links, but the user can press one of the two buttons or check the checkbox to move from the Home
page to the Feedback
page. Here’s how it works in action:
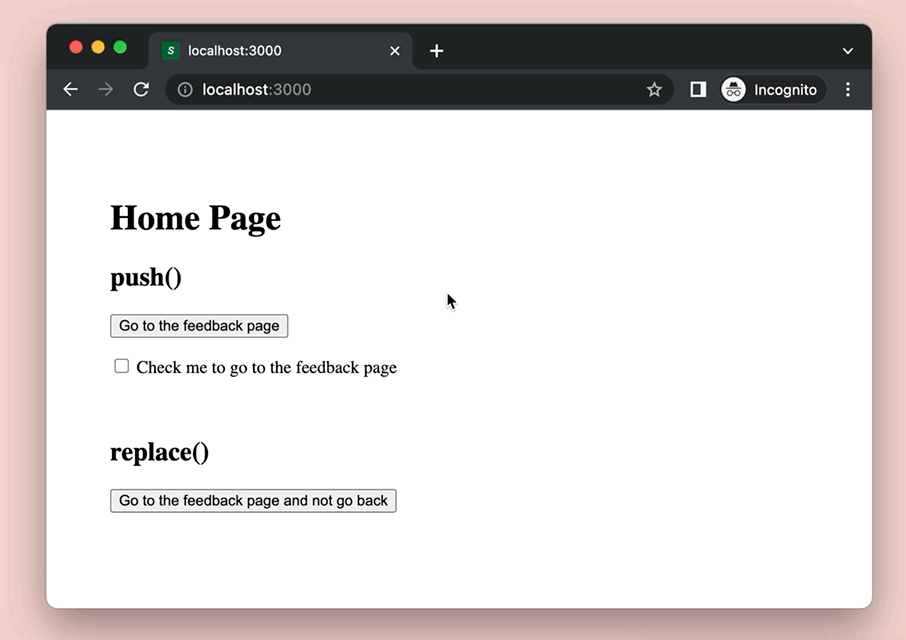
The Steps
1. To make sure we have the same starting point, please create a new project:
npx create-next-app example
Please select TypeScript when Next.js asks you.
When the project initialization is complete, create a new folder named feedback
in the app
directory of your project. After that, add a new file calledfeedback.tsx
inside the feedback
folder.
Here’s the file structure in the app
directory:
.
├── feedback
│ └── page.tsx
├── layout.tsx
└── page.tsx
2.. Add the following code to the file:
// app/feedback/page.tsx
const FeedBack = () => {
return (
<div style={{ padding: 50 }}>
<h1>Feedback Page</h1>
</div>
);
};
export default FeedBack;
3. And here’s the code in the app/page.tsx
:
import { useRouter } from 'next/router';
export default function Home() {
const router = useRouter();
return (
<div style={{ padding: 50 }}>
<h1>Home Page</h1>
<h2>push()</h2>
<p>
<button onClick={() => router.push('/feedback')}>
Go to the feedback page
</button>
</p>
<p>
<input
type="checkbox"
onChange={() => router.push('/feedback')}
></input> Check me to go to the feedback page
</p>
<br />
<h2>replace()</h2>
<div>
<button onClick={() => router.replace('/feedback')}>
Go to the feedback page and not go back
</button>
</div>
</div>
);
}
4. Replace the unwanted code (if any) in your app/layout.tsx file with the code below:
// app/layout.tsx (this is the root layout)
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang='en'>
<body suppressHydrationWarning={true}>{children}</body>
</html>
);
}
5. Get your app up and running:
npm run dev
Then go to http://localhost:3000
to see the result.