Routing refers to how an API’s endpoints (URIs) respond to incoming requests. In FastAPI, a route path, in combination with a request method, define the endpoint at which requests can be made. You can define a dynamic route path that can contain one or multiple path parameters (variables). Each parameter will be wrapped in a pair of curly braces { }.
Let’s examine the most minimal example below for more clarity:
from fastapi import FastAPI
app = FastAPI()
@app.get('/users/{user_id}/{user_name}')
async def get_users(user_id: int, user_name: str):
return {"user_data": {
"id": user_id,
"message": "Hello, " + user_name,
}}
The value of the path params user_id and user_name will be passed to the handler function as the arguments user_id and user_name.
If you run the code above and go to http://localhost:8000/users/123/ABCDEF with your web browser, you will see this:
{"user_data":{"id":123,"message":"Hello, ABCDEF"}}
Screenshot:
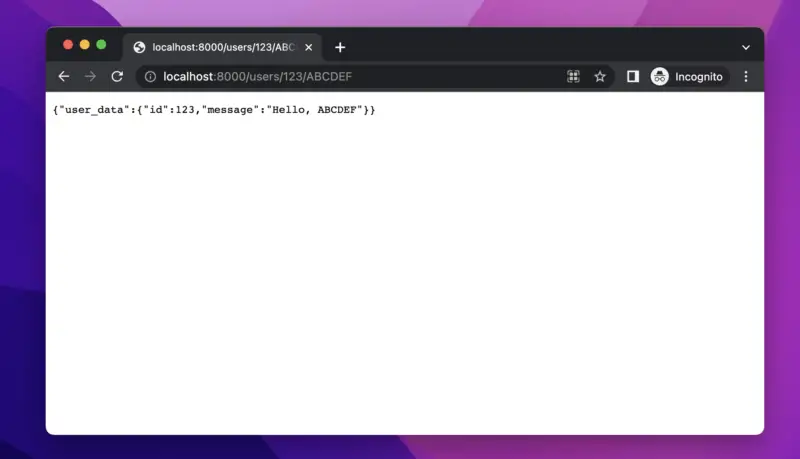
If you look closer at the code, you would notice that the types of the parameters were declared in the function, where user_id is int and user_name is str. This helps validate the values passed to the URL. If you enter this URL http://localhost:8000/users/foo/bar into the address bar, you will get an error:
{"detail":
[{
"loc":["path","user_id"],
"msg":"value is not a valid integer",
"type":"type_error.integer"
}]
}
The detailed information about the API was also automatically generated at http://localhost:8000/docs:
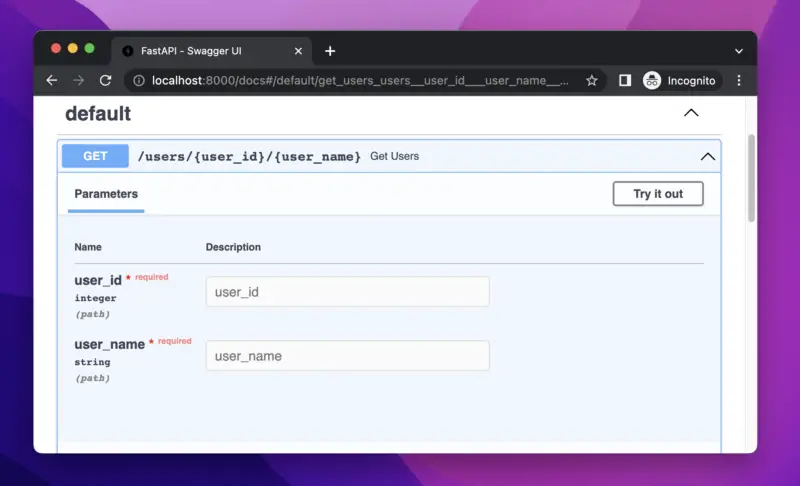
This will make it easier for developers using your API (for web frontend or mobile apps) to get the necessary insights.