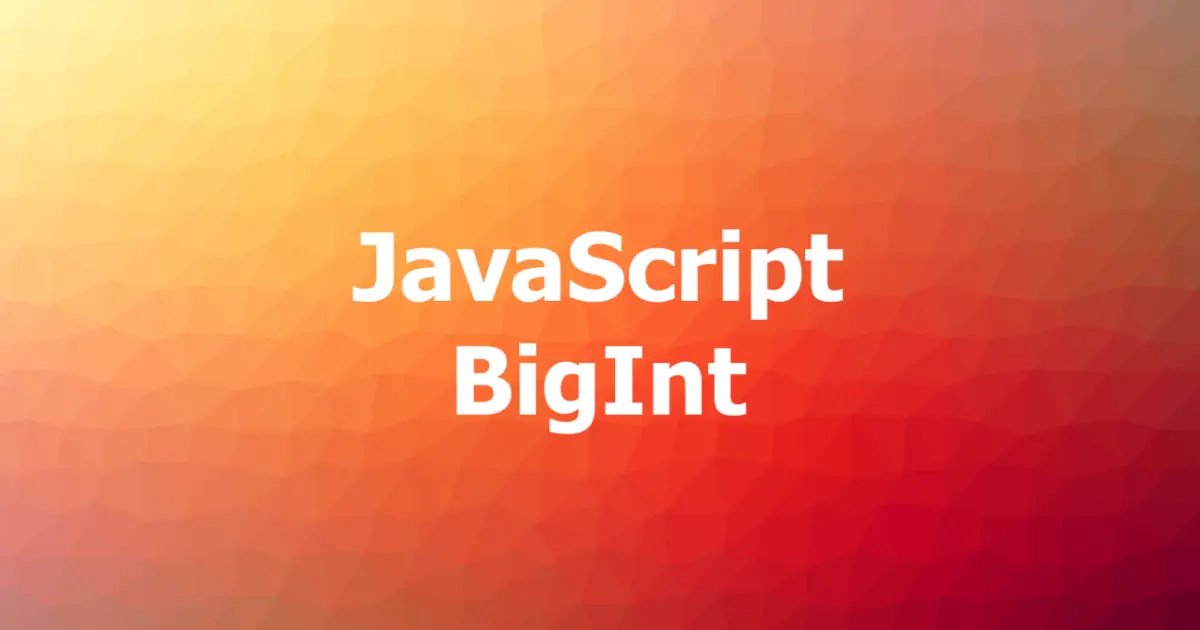
Overview
BigInt is a new data type in JavaScript that allows you to work with very large integers (greater than 2^53 – 1 or lower than -2^53 + 1 ) without losing precision. It has been supported by most web browsers since 2020 (today is 2023). BigInt values are useful for applications that need to handle large numbers, such as cryptography or scientific computing.
Let’s find more details about BigInt in this article and get our hands dirty by writing some code.
Creating BigInt values
BigInt values are created by appending n to the end of an integer literal or by calling the BigInt() function with an integer value or string value.
Example:
// using an integer literal
const x = 1234567890123456789012345678901234567890n;
// using BigInt() function
const y = BigInt(1234567890123456789012345678901234567890);
// using a string value with the BigInt() function
const z = BigInt('1234567890123456789012345678901234567890');
console.log("Type of x: " + typeof(x));
console.log("Type of y: " + typeof(y));
console.log("Type of z: " + typeof(z));
Output:
Type of x: bigint
Type of y: bigint
Type of z: bigint
Using BigInt values with arithmetic and bitwise operators
BigInt values can be used with most of the arithmetic and bitwise operators, except for the unary plus operator (+) and the unsigned right shift operator (>>>).
Example:
// exponentiation
let a = 2n ** 100n;
console.log('a =', a);
// addition
let b = a + 1n;
console.log('b =', b);
// subtraction
let c = b - a;
console.log('c =', c);
// multiplication
let d = c * 2n;
console.log('d =', d);
// division
let e = d / 3n;
console.log('e =', e);
// modulo
let f = e % 2n;
console.log('f =', f);
// bitwise AND
let g = f & 1n;
console.log('g =', g);
// bitwise OR
let h = g | 0n;
console.log('h =', h);
// bitwise XOR
let i = h ^ 1n;
console.log('i =', i);
// bitwise NOT
let j = ~i;
console.log('j =', j);
// bitwise left shift
let k = j << 2n;
console.log('k =', k);
// bitwise right shift
let l = k >> 1n;
console.log('l =', l);
Output:
a = 1267650600228229401496703205376n
b = 1267650600228229401496703205377n
c = 1n
d = 2n
e = 0n
f = 0n
g = 0n
h = 0n
i = 1n
j = -2n
k = -8n
l = -4n
BigInt values and primitive Number values
Mixing BigInt values and Number values
BigInt values cannot be mixed with primitive Number values in operations. They must be coerced into the same type first. That being said, you should be careful when converting BigInt values to Number values, as the precision of a BigInt value may be lost due to the conversion.
Don’t do this because it will cause a TypeError:
let a = 123n + 7;
Error:
TypeError: Cannot mix BigInt and other types, use explicit conversions
The code below works, but it isn’t recommended because the precision of the BigInt value can be lost:
let c = Number(999n) + 11;
The best way to use BigInt values with Number values is to turn Number values into BigInt values, as shown below:
let d = 999n + BigInt(11);
Comparing BigInt values with Number values
BigInt values can also be compared with Number values using the relational operators (<, >, <=, >=), but not with the strict equality operator (===). The loose equality operator (==) can be used, but it is not recommended as it may cause confusion.
Example:
let q = 92n < 93;
// true
let r = 82n > 481;
// true
let s = 42n <= 42;
// true
let t = 42n >= 43;
// false
let u = 42n === 42;
// false
let v = 42n == 42;
// true, but not recommended
BigInt values and hexadecimal, octal, or binary notation
BigInt values can also be written in hexadecimal, octal, or binary notation by using the 0x, 0o, or 0b prefixes, respectively.
Example:
// hexadecimal for 255
let w = 0xffn;
console.log(w);
// octal for 255
let x = 0o377n;
console.log(x);
// binary for 255
let y = 0b11111111n;
console.log(y);
Output:
255n
255n
255n
See also:
- JavaScript: Convert a byte array to a hex string and vice versa
- How to Generate Random Colors in JavaScript (4 Approaches)
The tutorial ends here. If you have any questions related to BigInt in modern JavaScript, just leave a comment. We’re more than happy to help. Happy coding & have a nice day!