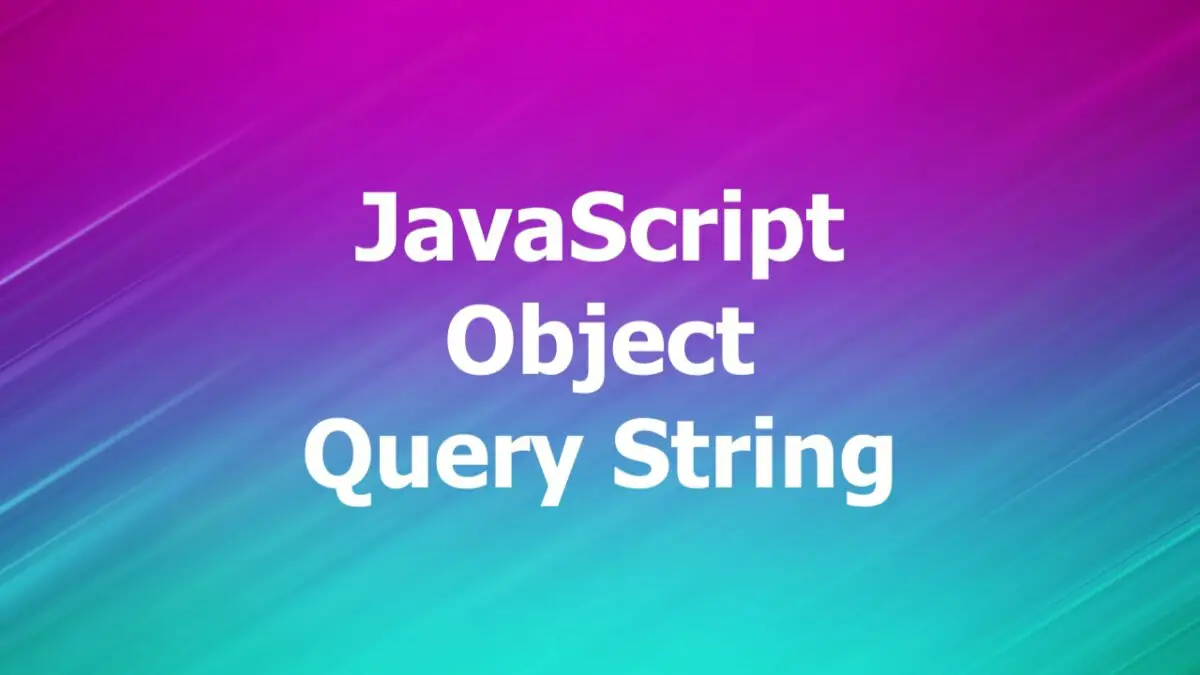
Overview
What is a query string?
In the world of JavaScript and web development, a query string is a set of characters tacked onto the end of a URL. The query string begins after the question mark (?) and can include one or more parameters. Each parameter is represented by a unique key-value pair or a set of two linked data items. The key is a constant defining a data set (e.g., country). The value is a variable belonging to that set. Let’s have a look at the URL below:
https://api.slingacademy.com/v1/sample-data/users?offset=0&limit=20&search=John
The query string of this URL is ?offset=0&limit=20&search=John.
Extracting a query string from a given URL
In order to extract the query string from a URL stored in a string variable, you can use the String.indexOf() and String.substring() methods to get the substring that starts from the first question mark as shown below:
const url =
'https://api.slingacademy.com/v1/sample-data/users?offset=0&limit=20&search=John';
const queryString = url.substring(url.indexOf('?'));
console.log(queryString);
// ?offset=0&limit=20&search=John
Convert a JavaScript object to a query string
You may need to convert an object to a query string if you want to send data to a web application or a back-end database using the URL.
To convert a JavaScript object to a query string, you can use the URLSearchParams interface, which provides utility methods to work with the query string of a URL.
Example:
const user = {
name: 'Sling Academy',
age: 999,
address: 'Under the sea',
};
const queryString = "?" + new URLSearchParams(user).toString();
console.log(queryString);
Output:
?name=Sling+Academy&age=999&address=Under+the+sea
Turn a query string into a JavaScript object
To parse a query string to a JavaScript object, you can use the same URLSearchParams interface but pass the query string to the constructor and then use the get() method to access the parameters.
const queryString = '?name=Sling+Academy&age=999&address=Under+the+sea';
const params = new URLSearchParams(queryString);
const userName = params.get('name');
const age = params.get('age');
const address = params.get('address');
console.log('Name:', userName);
console.log('Age:', age);
console.log('Address:', address);
Output:
Name: Sling Academy
Age: 999
Address: Under the sea
Conclusion
You’ve learned how to transform a JavaScript object into a query string and vice versa. This knowledge is very useful and can help you a lot when working with web projects. If you have any questions, please comment. Happy coding and have a nice day!