With Javascript, you can easily detect if the user clicks somewhere outside a given HTML element. For example, the code snippet below shows you how to catch a click outside an element with the id of my-box:
window.onclick = function (event) {
var myBox = document.getElementById('my-box');
if (event.target.contains(myBox) && event.target !== myBox) {
console.log('You clicked outside the box!');
} else {
console.log('You clicked inside the box!');
}
}
Below is the complete example with Javascript as well as HTML and CSS.
Example preview
We have a simple webpage that displays a red box. When you click inside the box, the console will print “You clicked inside the box!”. Otherwise, it will show “You clicked outside the box!”.
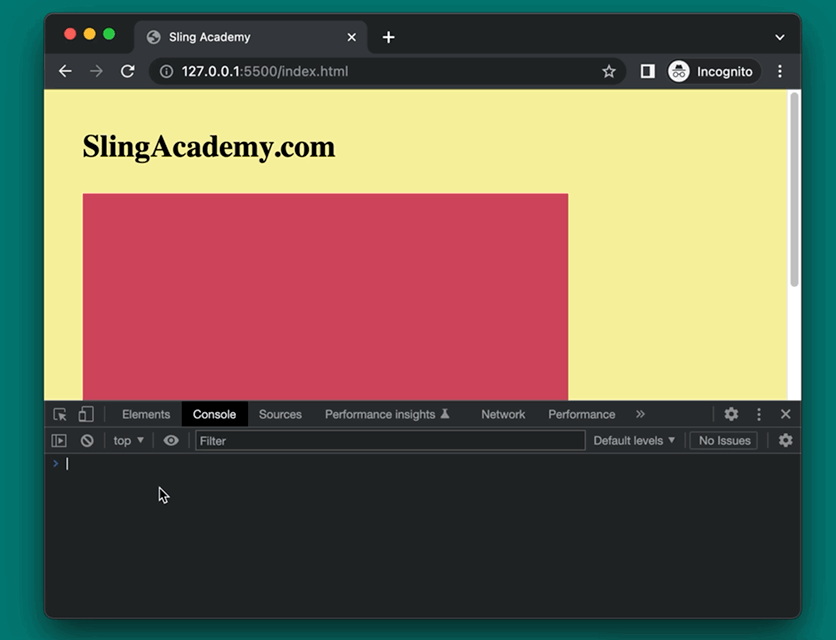
The code:
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<style>
* {
margin: 0;
padding: 0;
}
body {
padding: 40px;
background: rgb(247, 240, 155);
}
#my-box {
margin-top: 30px;
background: rgb(206, 69, 92);
width: 500px;
height: 350px;
cursor: pointer;
}
</style>
<title>KindaCode.com</title>
</head>
<body>
<h1>KindaCode.com</h1>
<div id="my-box"></div>
<script>
window.onclick = function (event) {
var myBox = document.getElementById('my-box');
if (event.target.contains(myBox) && event.target !== myBox) {
console.log('You clicked outside the box!');
} else {
console.log('You clicked inside the box!');
}
}
</script>
</body>
</html>
If you have any questions about this the code snippet above, please leave a comment. We’re more than happy to help.