This straightforward, practical article shows you how to determine the position (X and Y coordinates) of an element by using vanilla JavaScript.
What is the position of an element?
When you want to get the position of an element in JavaScript, you need to know where the element is located on the web page. The web page is like a big canvas that has a coordinate system with X and Y axes. The X-axis goes from left to right, and the Y-axis goes from top to bottom. The origin point (0, 0) is at the top left corner of the web page.
An element is a piece of HTML code that represents something on the web page, such as text, an image, a button, etc. Each element also has a position that tells where it is located on the web page. The position is usually given by two numbers: the Y coordinate and the Y coordinate. The X coordinate tells how far the element is from the left edge of the web page and the Y coordinate tells how far the element is from the top edge of the web page.
Determine the Position of an Element
Position in relation to viewport
You can use the element.getBoundingClientRect() function to measure the position of an element in JavaScript. This function returns an object with eight properties: top, right, bottom, left, x, y, width, and height. These properties tell you the position and size of the element relative to the viewport:
- left: The distance from the left edge of the element to the left edge of the viewport.
- top: The distance from the top edge of the element to the top edge of the viewport.
- right: The distance from the right edge of the element to the left edge of the viewport.
- bottom: The distance from the bottom edge of the element to the top edge of the viewport.
- width: The width of the element, including its padding and border width.
- height: The height of the element, including its padding and border width.
- x: The same as left.
- y: The same as top.
Quick example:
var element = document.getElementById("example-element");
var rect = element.getBoundingClientRect();
// the position related to the viewport
const x = rect.x;
const y = rect.y;
The viewport is part of the web page that you can see on your browser window. For example, if you have a web page that is 1000 pixels wide and 2000 pixels high, but your browser window is only 800 pixels wide and 600 pixels high, then you can only see part of the web page at a time. That part is called the viewport. The viewport also has a coordinate system with x and y axes, but its origin point (0, 0) is at the top left corner of the viewport, not the web page. So, when you use the element.getBoundingClientRect() function, you get the position and size of the element relative to the viewport, not the web page.
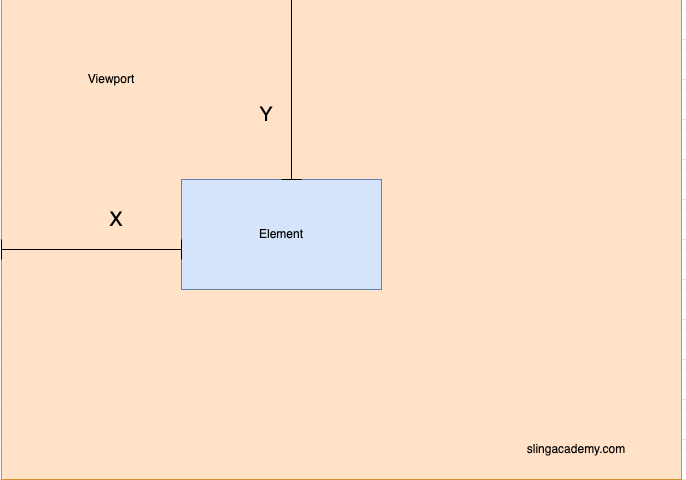
Position in relation to the page (with respect to page scroll)
To calculate the position of the element related to the web page, you need to add window.scrollX and window.scrollY as follows:
var element = document.getElementById("example-element");
var rect = element.getBoundingClientRect();
// the position related to the viewport
let x = rect.x;
let y = rect.y;
// get the postion related to the webpage
x = x + window.scrollX;
y = y + window.scrollY;
For more clarity, see the complete example below.
Complete example
Preview
The sample webpage we’re going to make contains a button and an element whose background color is light blue. When the button is clicked or when the window gets resized, the position of that element relative to the web page will be updated and displayed on the screen.
Here’s how it works:
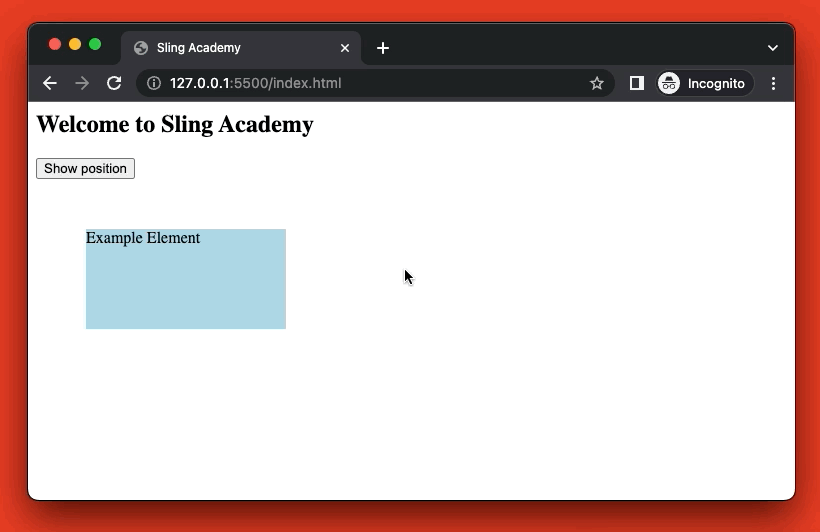
The code
The full source code that produces the demo above:
<html>
<head>
<title>Sling Academy</title>
<style>
/* We will get the position of this element */
#example-element {
width: 200px;
height: 100px;
background-color: lightblue;
margin: 50px;
}
/* The result will be displed within this element */
#output {
font-size: 30px;
color: blue;
}
</style>
</head>
<body>
<h2>Welcome to Sling Academy</h2>
<button id="show-position">Show position</button>
<div id="example-element">Example Element</div>
<div id="output"></div>
<script>
// Put your JS code here
// Get the elements by their ids
const button = document.getElementById("show-position");
const element = document.getElementById("example-element");
const output = document.getElementById("output");
// Define a function to display the position of the element
const displayPosition = () => {
// Use getBoundingClientRect() to get the position of the element
const rect = element.getBoundingClientRect();
const x = rect.left + window.scrollX;
const y = rect.top + window.scrollY;
// Create a string with the position information
const position = `X: ${x} <br>Y: ${y}`;
// Display the position in the output element
output.innerHTML = position;
}
// Add an event listener to the button to call displayPosition on click
button.addEventListener("click", displayPosition);
// Add an event listener to the window to call displayPosition on resize
window.addEventListener("resize", displayPosition);
</script>
</body>
</html>
Save this code in a file named index.html then open it with your favorite web browser. Enjoy your work!