Knowing that dark or light mode is being applied is useful for websites (and web apps) to programmatically adjust their UI based on the user’s preference or the device’s theme. This practical article will walk you through a complete example that demonstrates how to detect dark or light mode in vanilla JavaScript.
Table of Contents
What is the point?
We can do the job by using the matchMedia() method and the prefers-color-scheme media feature, like so:
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
console.log('User prefers dark mode');
} else {
console.log('User prefers light mode');
}
We can also use an event listener that will be triggered whenever the user changes their color scheme preference:
const detectColorScheme = () => {
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
console.log('User prefers dark mode');
} else {
console.log('User prefers light mode');
}
}
window.matchMedia('(prefers-color-scheme: dark)').addEventListener('change', detectColorScheme);
Now we will use all of these to build a complete little project.
Complete Example
Preview
The sample web page we’re going to make will have the following feature:
- Automatically use a dark background color and light text color when the device is in dark mode
- Automatically use a light background color and dark text color when the device is in light mode
Here’s the demo:
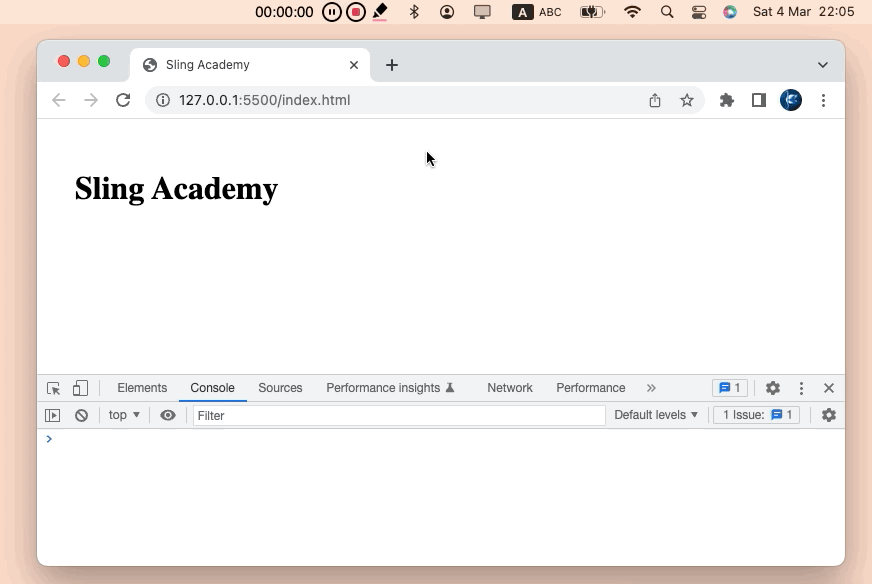
The Code
Create an HTML file, then add the following code to it:
<!DOCTYPE html>
<html>
<head>
<title>Sling Academy</title>
<script>
const detectColorScheme = () => {
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
console.log('User prefers dark mode');
document.body.style.backgroundColor = 'black';
document.body.style.color = 'white';
} else {
console.log('User prefers light mode');
document.body.style.backgroundColor = 'white';
document.body.style.color = 'black';
}
}
window.matchMedia('(prefers-color-scheme: dark)').addEventListener('change', detectColorScheme);
</script>
</head>
<body>
<h1 style="padding: 30px">Sling Academy</h1>
</body>
</html>
Save the file and open it with a web browser. Switch between dark mode and light mode on your computer and enjoy your results.
Conclusion
So we’re done with it, a small web page that adapts colors when the user switches the theme between light and dark. You can use this technique to enhance the user experience of your products. If you have any questions, please leave comments. Happy coding & have a nice day!