
In most websites and apps, there is an “I agree to terms and conditions” checkbox (the phrase in the quotes may differ slightly) at the registration screen. This is a method of protecting their business by requiring that users acknowledge the rules they must abide by when using their services. It is also a way of getting users to consent to their privacy policy or terms agreement, which is a legally binding contract.
Example Preview
In the example below, we are going to make a sample webpage (with vanilla JavaScript, HTML, and a little CSS) whose main parts are a checkbox and a button. When the checkbox is unchecked, the button is disabled and cannot be clicked. When the checkbox is checked, the button will be enabled, and the user can interact with it.
Here’s the demo:
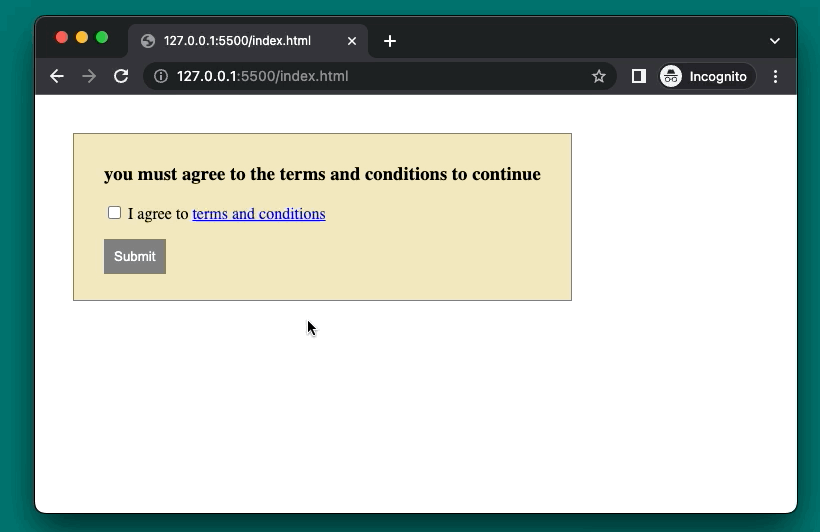
The Code
The complete code with explanations:
<html>
<head>
<style>
/* Style the container */
.container {
display: inline-block;
background: rgb(243, 233, 191);
border: 1px solid gray;
padding: 10px 30px;
margin: 30px;
}
/* Style the button */
#submit {
background-color: green;
color: white;
padding: 10px;
border: none;
cursor: pointer;
}
#submit:hover {
background-color: orange;
}
/* Style the button when disabled */
#submit:disabled {
background-color: gray;
cursor: not-allowed;
}
</style>
</head>
<body>
<div class="container">
<h3>you must agree to the terms and conditions to continue</h3>
<!-- Create a checkbox element -->
<div>
<input type="checkbox" id="agree">
<label for="agree">I agree to <a href="#">terms and conditions</a></label>
</div>
<!-- Create a button element -->
<p>
<button id="submit" disabled>Submit</button>
</p>
</div>
<!-- Include the JavaScript code -->
<script>
// Get the checkbox element
const checkbox = document.querySelector("#agree");
// Get the button element
const button = document.querySelector("#submit");
// Define a function to check the checkbox status
const checkStatus = () => {
// If the checkbox is checked, enable the button
if (checkbox.checked) {
button.disabled = false;
}
// Otherwise, disable the button
else {
button.disabled = true;
}
}
// Add an event listener to the checkbox to call the function on change
checkbox.addEventListener("change", checkStatus);
// Do something when the button is clicked
button.addEventListener("click", () => {
alert("Welcome to SlingAcademy.com");
});
</script>
</body>
</html>
You can save the code above into an HTML file and then open it with your favorite browser. Enjoy!