You can dynamically change the lang attribute of your HTML document just by using pure Javascript like this:
document.documentElement.setAttribute("lang", 'your language code');
For more clarity, take a look at the example below.
Table of Contents
Example
Preview
We are going to build a simple web app that contains 3 buttons. Each button is associated with a language code (en: English, es: Espanol, fr: French).
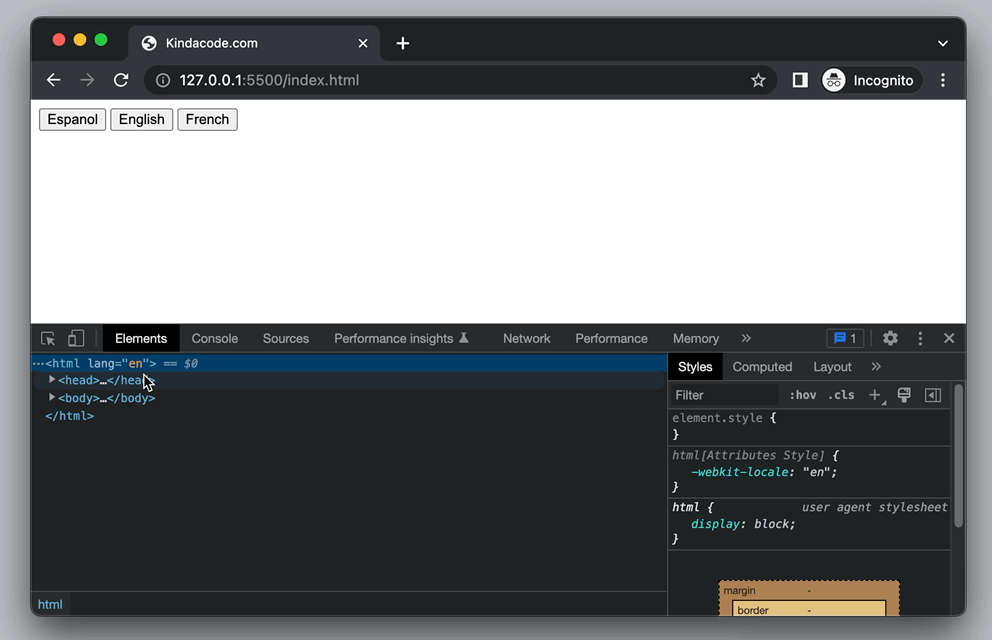
The Complete Code
<html lang="en">
<head>
<title>Kindacode.com</title>
<script>
// this function will be called when a button is clicked
const changeLang = (languageCode) => {
document.documentElement.setAttribute("lang", languageCode);
};
</script>
</head>
<body>
<div>
<button onclick="changeLang('es')">Espanol</button>
<button onclick="changeLang('en')">English</button>
<button onclick="changeLang('fr')">French</button>
</div>
</body>
</html>
That’s it. Happy coding!