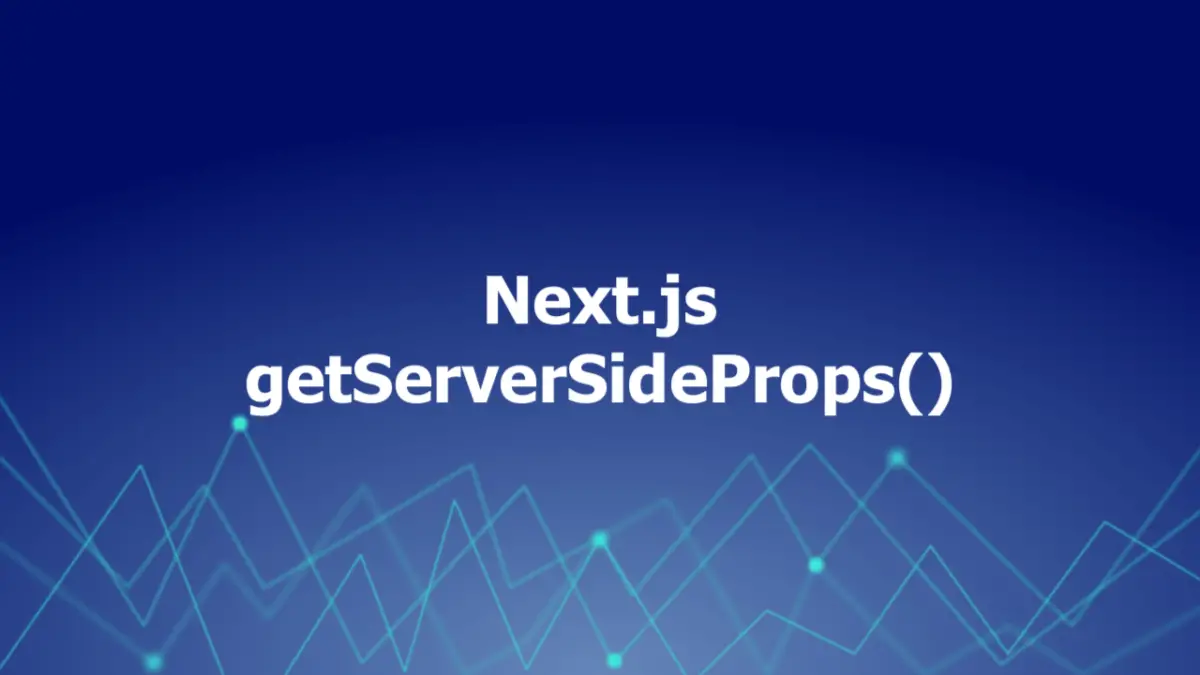
Overview
getServerSideProps() is a Next.js asynchronous function that can be exported from a page component (in your pages folder) to fetch data at the requested time on the server side before rendering the page. It is useful for dynamic pages that require data that cannot be determined at build time.
General Syntax
In the vast majority of cases, the usage of the getServerSideProps() function will look like this:
export async function getServerSideProps(context) {
// Fetch data from an external API or database
const data = await fetch('your API endpoint')
const jsonData = await data.json()
// Pass data to the page via props
return {
props: {
data: jsonData
}
}
}
Return Value & Parameters
getServerSideProps() should return an object with one of these properties: props, notFound, or redirect. The props property is an object that will be passed to the page component as props. The notFound property is a boolean that allows the page to return a 404 status and page. The redirect property is an object that allows the page to redirect to another URL with a specific status code.
getServerSideProps() receives a context parameter that contains useful information about the request:
- context.params: If this page uses a dynamic route, the params property contains the route parameters.
- context.req: The HTTP IncomingMessage object, with an additional cookies prop, which is an object with string keys mapping to string values of cookies.
- context.res: The HTTP response object.
- context.query: An object representing the query string, including dynamic route parameters.
- context.preview: preview is true if the page is in the Preview Mode and false otherwise.
- context.previewData: The preview data set by setPreviewData().
- context.resolvedUrl: A normalized version of the request URL that strips the _next/data prefix for client transitions and includes original query values.
- context.locale contains the active locale (if enabled).
- context.locales contains all supported locales (if enabled).
- context.defaultLocale contains the configured default locale (if enabled).
Important Notes
Some key points you should know before using the getServerSideProps() function:
- getServerSideProps() only runs on the server side and never on the browser. It runs every time you request the page, either directly or through client-side transitions.
- You can import modules in top-level scope for use in getServerSideProps(). Imports used will not be bundled for the client side. This means you can write server-side code directly in getServerSideProps(), including fetching data from your database.
- You should use getServerSideProps() only if you need to render a page whose data must be fetched at request time. This could be due to the nature of the data or properties of the request (such as authorization headers or geolocation). Pages using getServerSideProps() will be server-side rendered at request time and only be cached if cache-control headers are configured.
- You should avoid calling API routes from getServerSideProps(), as it will cause an extra request to be made due to both functions running on the server. Instead, directly import the logic used inside your API route into getServerSideProps().
See also: Read and Display Data from a Local JSON File using Next.js.
Complete Example
App Preview
This example will render a page with some information about a user on each request by using the data returned by the getServerSideProps() function. We will fetch data from the following REST API endpoint:
https://api.slingacademy.com/v1/sample-data/users/[id]
The user id is in the range from 1 to 1000.
The final result will look like this (if you look closely you will see that every time we change the id the content of the page changes too):
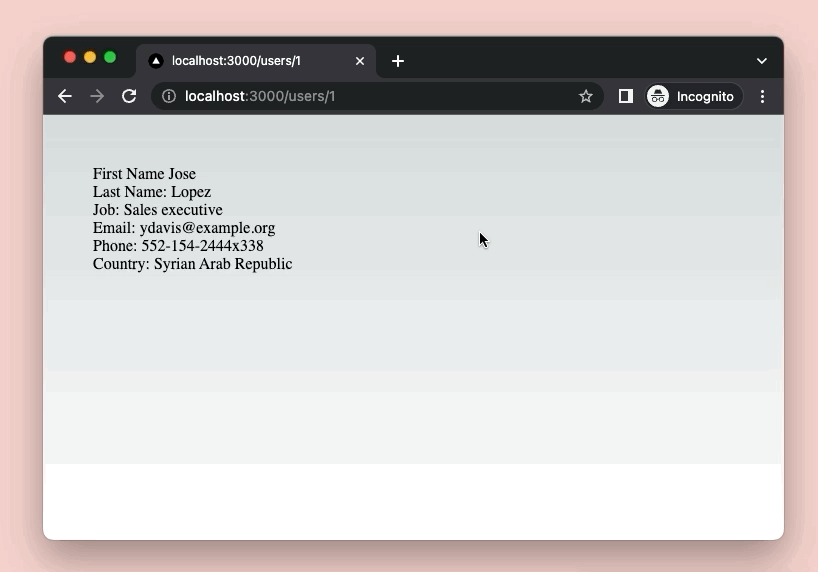
You can find more details about this API endpoint on this page: Sample Users – Free Fake API for Practicing & Prototyping.
Writing Code
In the pages folder of your Next.js project, create a new folder named users. Inside this folder, create a new file called [id].js, then add the following code to it:
// pages/users/[id].js
export default function User({ user }) {
// Render user data
return (
<div style={{ width: '100%', height: '100vh', padding: 50 }}>
<p>First Name {user.first_name}</p>
<p>Last Name: {user.last_name}</p>
<p>Job: {user.job}</p>
<p>Email: {user.email}</p>
<p>Phone: {user.phone}</p>
<p>Country: {user.country}</p>
</div>
);
}
export async function getServerSideProps(context) {
const { params } = context;
const { id } = params;
const URL = `https://api.slingacademy.com/v1/sample-data/users/${id}`;
// Fetch data from external API
const res = await fetch(URL);
const data = await res.json();
const user = data.user;
// Pass data to the page via props
return { props: { user } };
}
Run your project:
npm run dev
Open a new tab in your web browser, then go to the following URL:
http://localhost:3000/users/1
Replace the number 1 at the end of the URL with another integer from 2 to 1000 and see what happens!
Some other public mock REST APIs for practice:
- Sample Products – Mock REST API for Practice
- Sample Photos – Free Fake REST API for Practice
- Sample Blog Posts – Public REST API for Practice
Happy coding & have a nice day!