In Next.js, you can get the query string parameters of a URL by using the useRouter hook like this:
import { useRouter } from "next/router";
/*...*/
const router = useRouter();
const queryParams = router.query;
console.log(queryParams);
/*...*/
For more completeness and clarity, see the example below.
Example
Let’s say you have a products page with the following code:
// pages/product.js
import { useRouter } from "next/router";
import { useEffect } from "react";
const Products = () => {
const router = useRouter();
// Check whether router.query is empty {} or not
if (Object.keys(router.query).length !== 0) {
const queryParams = router.query;
console.log(queryParams);
console.log("Search", queryParams.search);
console.log("Page", queryParams.page);
console.log("Limit", queryParams.limit);
}
return (
<div style={styles.center}>
<h1>Products</h1>
</div>
);
};
export default Products;
const styles = {
center: {
width: "100%",
height: "100vh",
display: "flex",
justifyContent: "center",
alignItems: "center",
},
};
Now when you go to:
http://localhost:3000/products?search=abc&page=1&limit=10&sort=id&order=desc
Check your console, and you will see:
{search: "abc", page: "1", limit: "10", sort: "id", order: "desc"}
Search abc
Page 1
Limit 10
This result is the same as the screenshot below:
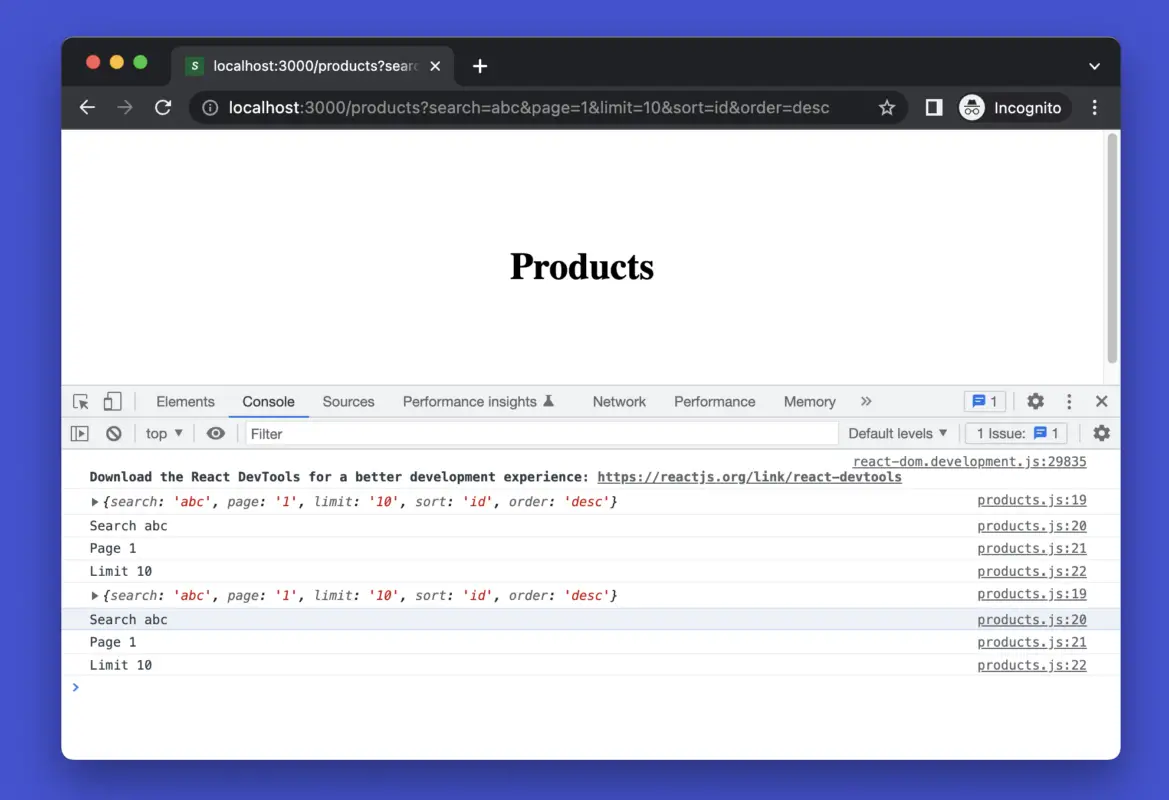
That’s it. Happy coding!