In Next.js 13.3 and other newer versions of the framework, you can extract segment data from a dynamic route by using the useParams()
hook. It returns an object whose properties name is the segment’s name, and the properties value is what the segment is filled in with. Note that to use hooks in a component in the app
directory, you have to add the use client
directive to the top of the corresponding file. For more clarity, see the complete example below.
Complete Example
Let’s say the app
folder of a Next.js project has a structure as follows:
.
├── layout.tsx
└── users
└── [uid]
└── page.tsx
Then we can access a URL with a specific uid
like so:
/users/123
We can get uid
like this:
const params = useParams();
const uid = params.uid;
Demo:
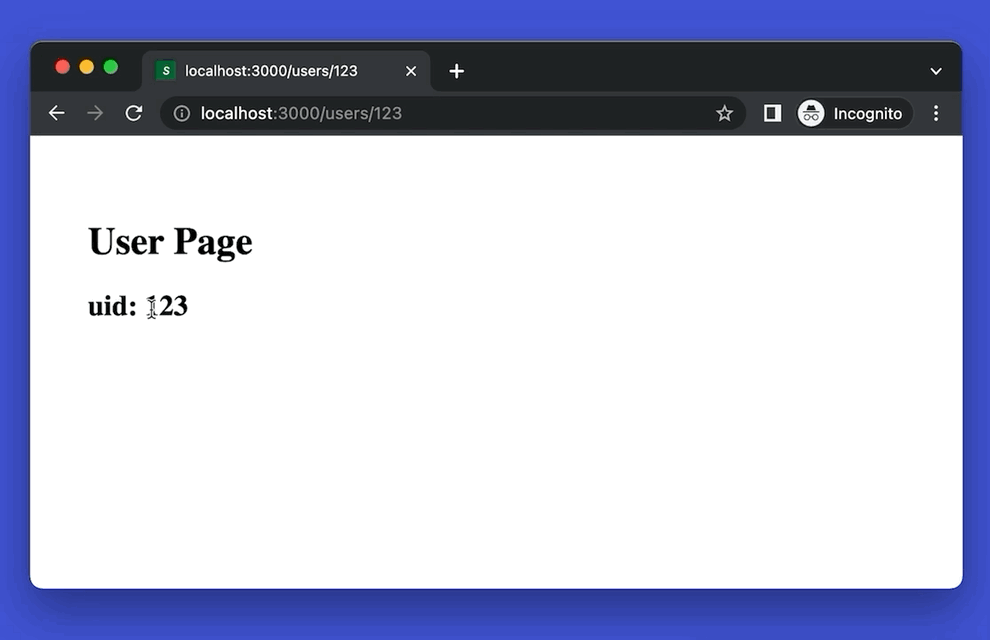
The full code in app/users/[uid]/page.tsx
:
// app/users/[uid]/page.tsx
// This directive is required to use hooks
'use client';
import { useParams } from 'next/navigation';
export default function User() {
const params = useParams();
const uid = params.uid;
return (
<div style={{ padding: 40 }}>
<h1 style={{fontSize: 40, fontWeight: 'bold'}}>User Page</h1>
<h2 style={{fontSize: 30, fontWeight: 'bold'}}>uid: {uid}</h2>
</div>
);
}
You can do the same with nested dynamic routes.
And here is the root layout (which is required if you’re using the app
directory):
// app/layout.tsx
export const metadata = {
title: 'Sling Academy',
description: 'This is a meta description.',
}
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
All the code snippets above were written in TypeScript. However, since our example is very simple, it makes no difference to JavaScript.