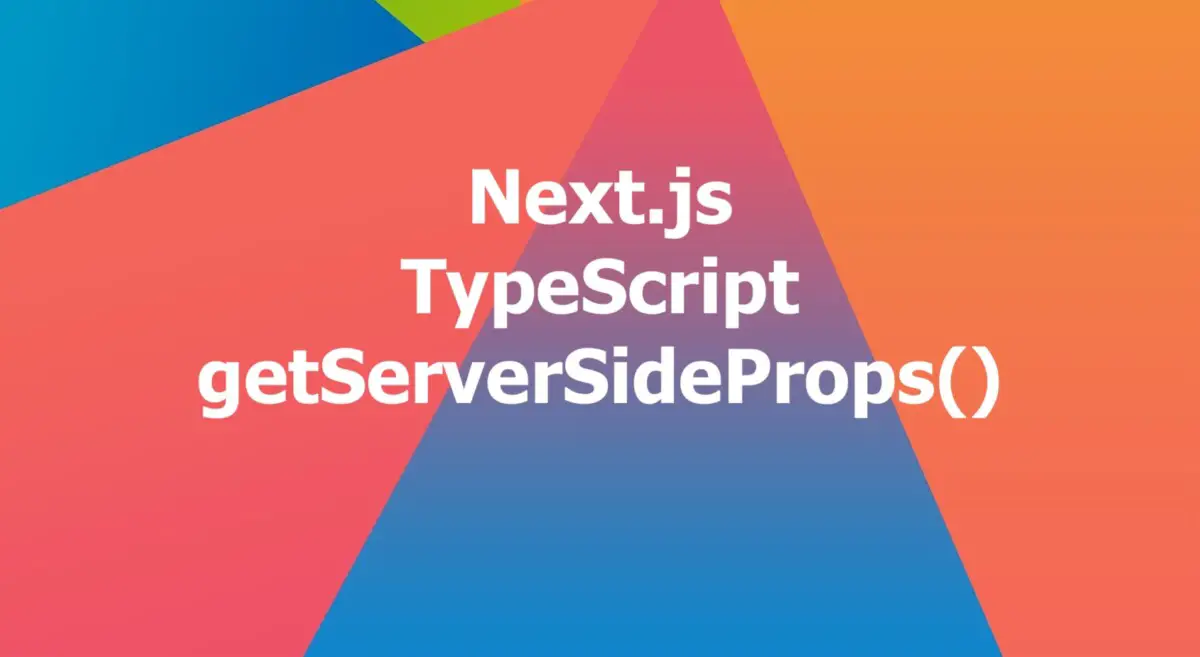
Table of Contents
Overview
In Next.js, the getServerSideProps() function is a way to fetch data on the server side and pass it as props to your page component. It is useful for dynamic data that changes often and needs to be updated on each request.
To use the getServerSideProps() function with TypeScript, you need to import the GetServerSideProps type from next and annotate your function with it. This will help you avoid type errors and get autocomplete suggestions for the context parameter and the return value. In general, the syntax will look as follows:
import { GetServerSideProps } from 'next';
// Define a type for our props
interface Data {/*.../*}
export const getServerSideProps: GetServerSideProps<{data: Data}> = async (context) => {
// fetching data here
// Return the data as props
return {
props: {
data
},
};
};
You need to make sure that the props object you return is serializable, meaning it can be converted to JSON. In other words, you cannot return functions, class instances, symbols, or undefined values as props. If you do so, you will get an error like “Reason: undefined cannot be serialized as JSON. Please use null or omit this value.”
Problems may come during data fetching, such as network errors, API errors, or validation errors. Using use try/catch blocks to handle errors is a good idea. You can also use the notFound or redirect properties in the return value to show a 404 page or redirect to an alternative page if an error happens.
These words might be boring and confusing. See the full example below for more clarity.
Complete Example
App Preview
What we are going to make is a sample Next.js page that fetches a dummy product with a specified id and then displays the information of that product (name, price, description, category) on the page.
Here’s how it works:
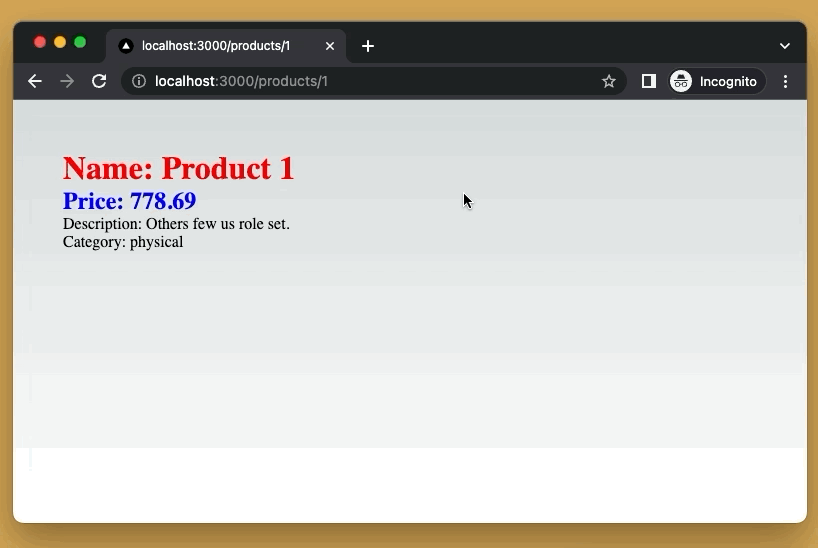
The Code
Here’s the API endpoint we will use in our code:
https://api.slingacademy.com/v1/sample-data/photos/[product id]
To make sure we have the same starting point, you should initialize a brand new Next.js project that uses TypeScript.
In your pages directory of your project, add a new folder named products. Inside this products folder, create a new file called [id].tsx. From now on, we will only care about this one. Put the following code into it:
// pages/products/[id].tsx
import { GetServerSideProps } from 'next';
// Define a type for our product data
type Product = {
id: number;
name: string;
description: string;
price: number;
category: 'physical' | 'digital';
};
// Define a type for our props
interface PropductProps {
product: Product;
}
// Export the page component
export default function productsPage({ product }: PropductProps) {
// Render the product
return (
<div style={{ height: '100vh', padding: 50 }}>
<h1 style={{ color: 'red' }}>Name: {product.name}</h1>
<h2 style={{ color: 'blue' }}>Price: {product.price}</h2>
<p>Description: {product.description}</p>
<p>Category: {product.category}</p>
</div>
);
}
// Export the getServerSideProps function with GetServerSideProps type
export const getServerSideProps: GetServerSideProps<{
product: Product;
}> = async (context) => {
// get product id from the url
const productId = parseInt(context.params?.id as string) || 1;
// Define the API url with the product id
const API_URL = `https://api.slingacademy.com/v1/sample-data/products/${productId}`;
// Fetch data
const res = await fetch(API_URL);
// Parse the data
const data = await res.json();
const product = data.product;
// If the product is not found, return notFound - 404 page
if (product === null) {
return {
notFound: true,
};
}
// Return the product as props
return {
props: {
product,
},
};
};
Get your Next.js app up and running:
npm run dev
Then go to http://localhost:3000/products/1 to view the result. Change 1 at the end of the URL with another number from 2 to 1000 and see what happens.
You can find more details about the API in this article: Sample Products – Mock REST API for Practice. Below are some other free mock APIs for learning and testing:
- Sample Users – Free Fake API for Practicing & Prototyping
- Sample Photos – Free Fake REST API for Practice
- Sample Blog Posts – Public REST API for Practice
The tutorial ends here. Happy coding & have a nice day!