This article shows you how to enable ES6 import/export
in Node.js and use both require
and import
in the same file.
Enabling ES import/export
You can use ES6 import/export
in Node.js by simply adding "type": "module"
to your package.json
file, like this:
{
"type": "module"
}
You can also save a file with the .mjs
extension to be able to use import/export
, for example:
// abc.mjs
const abc = () => {
console.log('hello')
}
export default abc;
// index.js
import abc from './abc.mjs';
abc()
Using both “require” and “import” in the same file
What if you want to use both require
and import
in the same file? Is it possible? The answer is “Yes” but you have to do some extra things. Otherwise, you will get the following error:
ReferenceError: require is not defined in ES module scope, you can use import instead
To use require
in the ES module scope, you have to define it. Just two lines of code to get the job done:
// These lines make "require" available
import { createRequire } from "module";
const require = createRequire(import.meta.url);
A Complete Example
A good example that you cannot use import
directly is with a JSON file. In this case, you can use require
or read the content from the JSON file using the fs
module. In this example, we will choose the first option because it is short and easy.
The sample project we are going to build is really plain. Its job is to send data from a JSON file to the user after they make a GET request to http://localhost:3000
(with a web browser or Postman or whatever HTTP tool you like).
1. Go to the folder you want your project to live in then install ExpressJS by running:
npm i express
Create a subfolder named src and add two empty files to it: data.json
and index.js
. Now the project structure looks like this:
.
├── node_modules
├── package-lock.json
├── package.json
└── src
├── data.json
└── index.js
2. Add "type": "module"
to your package.json
to enable ES6 import/export
:
{
"name": "sling.academy",
"version": "1.0.0",
"description": "",
"type": "module",
"main": "index.js",
"scripts": {
"dev": "nodemon src/index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.18.1"
}
}
3. Put the code below to your index.js
:
// index.js
import express from "express";
// Define "require"
import { createRequire } from "module";
const require = createRequire(import.meta.url);
const data = require("./data.json");
const app = express();
app.use(express.json());
app.get("/", (req, res) => {
res.status(200).send(data);
});
app.listen(3000, (error) => {
if (error) {
throw new Error(error);
}
console.log("Backend is running");
});
4. Gives your data.json
some data:
{
"name": "John Doe",
"age": 101,
"occupation": "astronaut"
}
5. Run the project and go to http://localhost:3000
by using your browser or Postman.
The result:
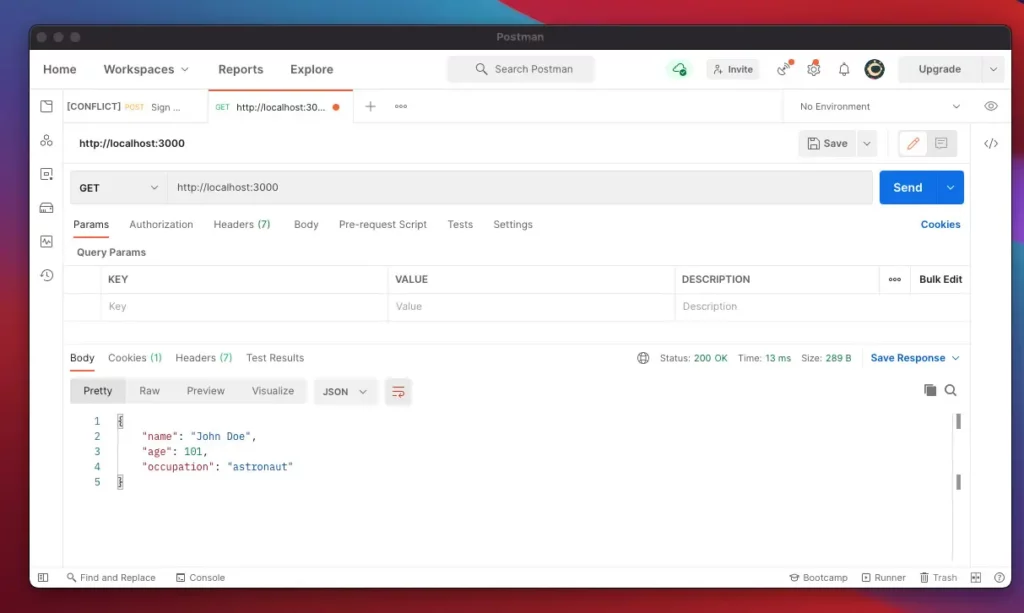
Conclusion
Cases where it is necessary to use both require
and import
in a single file, are quite rare, and it is generally not recommended and considered not a good practice. However, sometimes it is the easiest way for us to solve a problem. There are always trade-offs and the decision is up to you.