Introduction
The numpy.random.Generator.binomial()
method is a powerful tool in NumPy, an essential library for scientific computing in Python. This method allows you to generate random binomial distributed numbers, which is crucial in many fields of science and engineering, particularly in statistics and data analysis. In this guide, we will explore how to use this method through four increasing complexity examples.
What is Binomial Distribution?
Before diving into examples, let’s understand what a binomial distribution is. In probability theory and statistics, a binomial distribution with parameters n
and p
is the discrete probability distribution of the number of successes in a sequence of n
independent experiments, each asking a yes/no question, and each with its success probability p
. Here, n
indicates the number of trials, and p
is the probability of success on each trial.
Getting Started with Generator.binomial()
To utilize the numpy.random.Generator.binomial()
method, you first need to create a generator object using numpy.random.default_rng()
. This generator will be your source of randomness for generating binomial distribution numbers.
A basic example of using the binomial method is as follows:
import numpy as np
rng = np.random.default_rng()
samples = rng.binomial(n=10, p=0.5, size=1000)
print(samples[:10])
This piece of code generates 1000 samples where each sample is the result of 10 coin flips, assuming a fair coin (p=0.5). The first ten samples might look like this:
[5, 6, 7, 4, 5, 7, 6, 5, 3, 5]
Here, each number represents the number of heads observed in 10 flips.
Visualizing a Binomial Distribution
A binomial distribution can be visualized using histograms. Let’s plot the distribution of the samples generated in our first example using Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
rng = np.random.default_rng()
# Generate the samples
samples = rng.binomial(n=10, p=0.5, size=1000)
# Plot the histogram
plt.hist(samples, bins=range(0, 11), edgecolor='black')
plt.xlabel('Number of Heads')
plt.ylabel('Frequency')
plt.title('Histogram of Binomial Distribution')
plt.show()
Output (vary, due to the randomness):
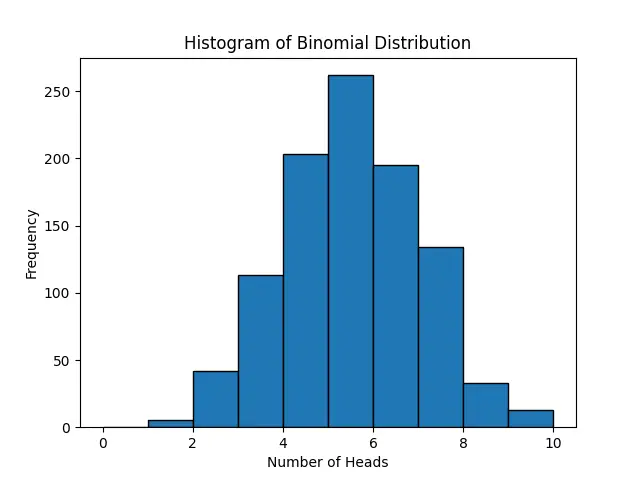
The histogram gives a visual representation of how the results of the binomial trials are distributed, reinforcing the properties of a binomial distribution.
Using binomial() for Probability Estimates
Next, let’s use the numpy.random.Generator.binomial()
method to estimate the probability of observing a certain number of successes. For instance, what’s the probability of getting exactly 5 heads in 10 coin flips? You can use the np.mean()
function to calculate this probability as follows:
import numpy as np
rng = np.random.default_rng()
samples = rng.binomial(n=10, p=0.5, size=100000)
probability = np.mean(samples == 5)
print(f'Probability of getting exactly 5 heads in 10 flips: {probability:.4f}')
Output (vary);
Probability of getting exactly 5 heads in 10 flips: 0.2463
In this case, since the number of simulations is large (100,000), the estimated probability is quite close to the theoretical probability.
Simulating a Real-World Scenario
Finally, let’s apply the numpy.random.Generator.binomial()
to a real-world scenario. Imagine you’re analyzing the success rate of a new drug in a clinical trial. If the drug is given to 1000 patients and each has a 0.1 chance of successful treatment, you can simulate this scenario as follows:
import numpy as np
rng = np.random.default_rng()
patients = 1000
success_rate = 0.1
# Simulate the scenario
results = rng.binomial(n=1, p=success_rate, size=patients)
successes = np.sum(results)
print(f'Successful treatments: {successes}')
Output (vary):
Successful treatments: 98
In this simulated experiment, results
is an array where each element represents the outcome for each patient (1 for success, 0 for failure). The total number of successful treatments are then summed up to get the final count.
Conclusion
This tutorial walked you through the process of generating random numbers following a binomial distribution using numpy.random.Generator.binomial()
. By understanding and applying these concepts, you can simulate a wide range of real-world scenarios and perform statistical analyses with ease. The flexibility and power of NumPy make it an indispensable tool for anyone delving into data science, statistics, or related fields.