Overview
NumPy is a fundamental package for scientific computing with Python. Among its array of features, the NumPy library allows users to generate random numbers, which is crucial for various computational tasks, such as simulations, testing, and probabilistic algorithms. In this article, we’ll delve into the random.Generator.uniform()
method, which is part of the new NumPy random API introduced in version 1.17. This method generates samples from a uniform distribution. Our journey will cover a comprehensive guide on using this method, spanned across four detailed examples.
Syntax & Parameters
Syntax:
generator.uniform(low=0.0, high=1.0, size=None)
Parameters:
- low: float or array_like of floats, optional. Lower boundary of the output interval. All values generated will be greater than or equal to
low
. The default value is0.0
. - high: float or array_like of floats, optional. Upper boundary of the output interval. All values generated will be less than
high
. The default value is1.0
. - size: int or tuple of ints, optional. Specifies the shape of the returned array of samples. If not provided, a single value is returned.
Prerequisites: To follow along with this tutorial, you should have a basic understanding of Python and have NumPy installed on your system. If it’s not installed yet, you can do so by running pip install numpy
in your terminal or command prompt.
Example 1: Basic Usage of random.Generator.uniform()
Let’s start with the most straightforward example of generating a single random float number between 0 and 1.
import numpy as np
rng = np.random.default_rng()
number = rng.uniform()
print(f'Generated Number: {number}')
Output:
Generated Number: 0.5299440027475123
This example shows how to initializeredissect and utilize the random number generator and subsequently call the uniform()
method without any arguments except for the self parameter, which is passed automatically. The generated number falls within the default interval [0, 1).
Example 2: Specifying Interval
Next, we extend our exploration by specifying the interval over which our uniform distributed numbers should be generated. We’ll generate five random numbers within the range of -10 to 10.
import numpy as np
rng = np.random.default_rng()
numbers = rng.uniform(-10, 10, 5)
print(f'Generated numbers: {numbers}')
Output:
Generated numbers: [ 0.6152581 -2.74917881 3.43250728 -9.14228731 7.62758643]
By providing the lower bound, upper bound, and the number of samples to generate, we can control the range and quantity of the random numbers produced.
Example 3: Generating a Uniformly Distributed Array
Building upon our knowledge, let’s generate a 2D array of uniformly distributed random numbers. Having an array of random numbers can be especially useful in simulations and modeling.
import numpy as np
rng = np.random.default_rng()
array = rng.uniform(0, 5, (3, 4))
print(f'Generated 2D array:\n{array}')
Output:
Generated 2D array:
[[ 1.00780959 3.55934491 0.57509333 3.8873416 ]
[ 2.44539653 0.8349957 1.7535448 4.48609164]
[ 4.61893845 2.4156952 4.18399048 3.53310349]]
This code snippet illustrates how to generate a 2-dimensional array with specified dimensions. Each element in the array is a random float number uniformly distributed over the interval [0, 5).
Example 4: Reproducibility with Seed
In scientific computations and simulations, reproducibility is often critical. The uniform()
method allows for reproducible results by setting the seed value.
import numpy as np
seed = 42
rng = np.random.default_rng(seed)
number = rng.uniform(0, 10)
print(f'Generated number with seed 42: {number}')
Output:
Generated number with seed 42: 3.745401188473625
By initializing the random number generator with a seed, we ensure that the same sequence of numbers can be generated each time the script is run, thus ensuring predictability and reproducibility of our results.
Example 5: Histogram of Uniformly Distributed Samples
import numpy as np
import matplotlib.pyplot as plt
# Create a Generator instance
rng = np.random.default_rng(seed=2024)
# Generate 1000 samples from a uniform distribution
samples = rng.uniform(low=-10, high=10, size=1000)
# Plotting the histogram of the samples
plt.hist(samples, bins=30, density=True, alpha=0.6, color='g')
plt.title('Histogram of Uniformly Distributed Samples')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
Output:
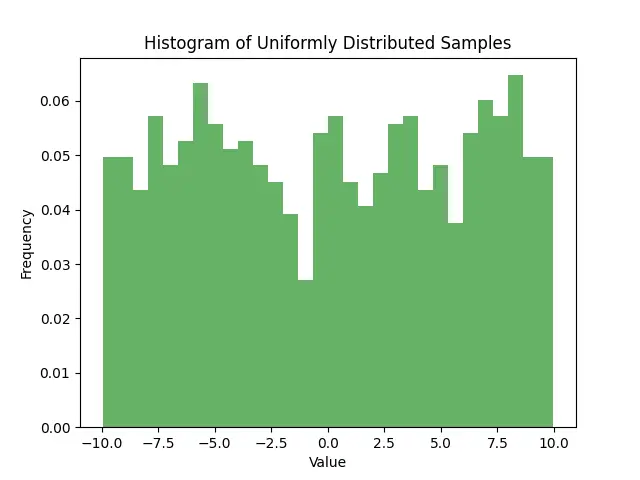
he plot above shows a histogram of 1000 samples generated from a uniform distribution ranging from -10
to 10
. The distribution is approximately uniform, as indicated by the roughly equal height of each bin, which is consistent with the properties of a uniform distribution where each interval within the range has an equal probability of containing a sample.
Conclusion
Throughout four illustrative examples, we journeyed from generating a single random float to producing an array of uniformly distributed numbers, covering intermediate steps and emphasizing important considerations such as intervals and reproducibility. Understanding how to effectively use the random.Generator.uniform()
method lays a solid groundwork for using NumPy’s random number generating capabilities in scientific computing and data science projects. Effectively wielding this tool can enable better simulations, model testing, and much more in Python.