Introduction
NumPy, a foundational package for numerical computing in Python, offers extensive support for arrays, mathematical operations, and a rich library of statistical functions. Among these, the random.Generator.lognormal()
method is a powerful tool for generating log-normal distributed random numbers. This tutorial will walk you through the basics to more advanced examples of using the lognormal()
method, illustrating with practical examples.
The Fundamentals
The numpy.random.Generator.lognormal()
method draws samples from a log-normal distribution. The log-normal distribution is the probability distribution of a random variable whose logarithm is normally distributed.
Syntax:
generator.lognormal(mean=0.0, sigma=1.0, size=None)
Parameters:
- mean: float or array_like of floats, optional. The mean value of the underlying normal distribution. The default value is
0.0
. - sigma: float or array_like of floats, optional. The standard deviation of the underlying normal distribution. Must be non-negative. The default value is
1.0
. - size: int or tuple of ints, optional. Specifies the output shape. If not provided, a single value is returned. If an integer is given, the result is a 1-D array of that length. A tuple can be used to specify a multi-dimensional array.
Returns:
- out: ndarray or scalar. Drawn samples from the parameterized log-normal distribution.
Example 1: Basic Usage of lognormal()
Let’s start with the simplest example. The following code generates a single random number from a log-normal distribution:
import numpy as np
rng = np.random.default_rng()
number = rng.lognormal()
print(number)
Output (vary):
0.7540904184261428
This example uses default parameters for the lognormal()
method, producing a random number where the underlying normal distribution has a mean of 0 and a standard deviation of 1.
Example 2: Generating Multiple Numbers
Increasing complexity, let’s generate multiple random numbers from a log-normal distribution:
import numpy as np
rng = np.random.default_rng()
size = 5
numbers = rng.lognormal(mean=0, sigma=1, size=size)
print(numbers)
Output (vary):
[1.25444759 1.34336275 1.63953346 0.18955023 0.31220918]
In this case, we generate an array of five random numbers using the same distribution parameters. This can be useful for simulations or modeling.
Example 3: Custom Parameters
Diving deeper, you can customize the distribution parameters to fit your needs. Let’s adjust the mean (mean
) and standard deviation (sigma
) of the underlying normal distribution:
import numpy as np
rng = np.random.default_rng()
mean = 0.5
sigma = 0.2
numbers_custom = rng.lognormal(mean, sigma, 1000)
print(numbers_custom.mean(), numbers_custom.std())
Output (vary):
1.6822432437076735 0.34812197748092
This demonstrates how changing the parameters affects the generated distribution, providing a useful way to simulate different conditions in statistical modeling.
Example 4: Visual Representation
Finally, to fully grasp the impact of our parameters, let’s graph the generated log-normal distribution against a normal distribution:
import matplotlib.pyplot as plt
import numpy as np
rng = np.random.default_rng()
dist = rng.lognormal(0.5, 0.2, 1000)
# Plotting
plt.hist(dist, bins=50, alpha=0.75, label='Log-normal')
plt.legend()
plt.show()
Output (vary):
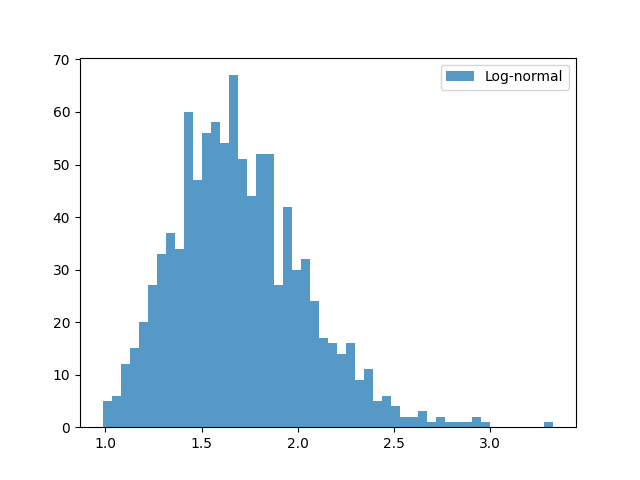
Through this visualization, you can clearly see the skewness and shape of the log-normal distribution, allowing for a more intuitive understanding of the data.
Conclusion
The lognormal()
method in NumPy’s random module is a versatile tool for generating numbers following a log-normal distribution. From generating a single random number to creating complex distributions with custom parameters and visualizing them, this tutorial has covered essential aspects to help you get started. With practice, you’ll find numerous applications of this method in your data analysis and model simulations.