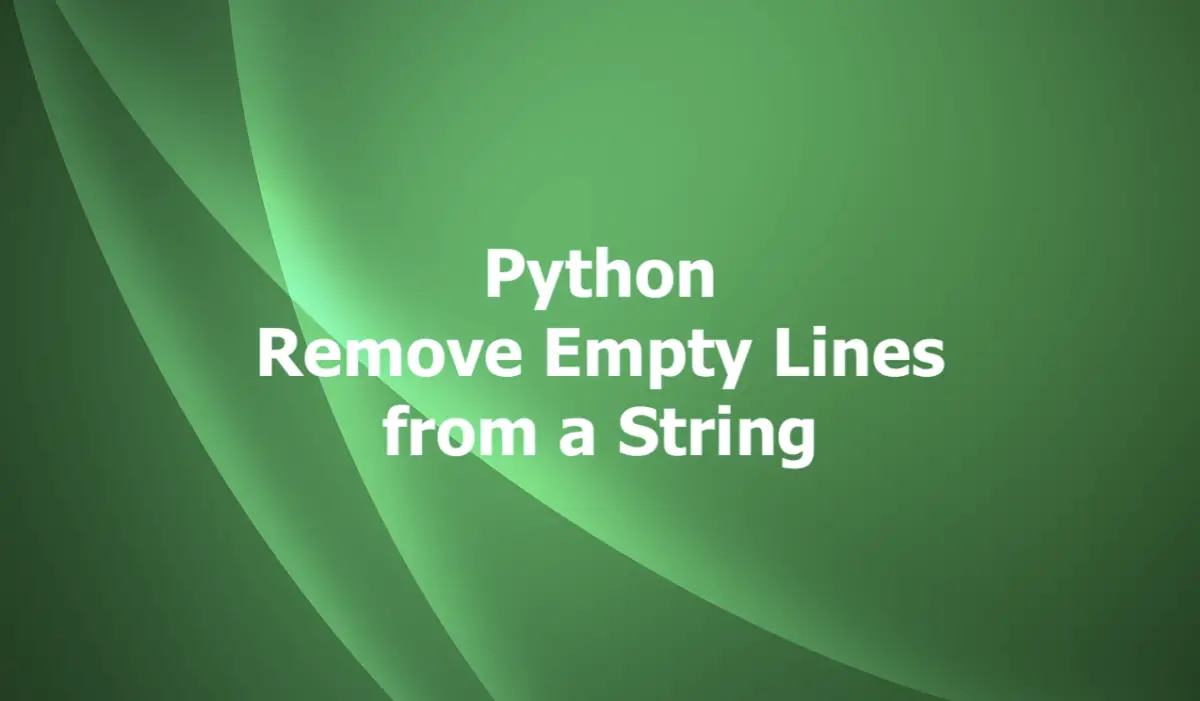
A multiple-line string might contain one or many empty lines. Empty lines refer to lines in a text or string that contain no visible content or characters. They are lines that appear blank or have only whitespace characters, such as spaces, tabs, or line breaks:
This is line 1.
This is line 3.
This is line 5.
This concise, code-focused article will walk you through several examples that demonstrate how one can eliminate empty lines from a given string in Python in order to make the resulting string more compact and meaningful.
Using a List Comprehension
The idea here is to split the input string into lines, iterate over each line using a list comprehension, and select only the non-empty lines. Finally, join these lines back together to form a single string.
input_string = """
Welcome to Sling Academy
This is a multi-line string.
It contains empty lines.
There are some empty lines above this.
And another one below this.
Thank you!
"""
def remove_empty_lines(text):
lines = text.split('\n')
non_empty_lines = [line for line in lines if line.strip() != '']
return '\n'.join(non_empty_lines)
print(remove_empty_lines(input_string))
Output:
Welcome to Sling Academy
This is a multi-line string.
It contains empty lines.
There are some empty lines above this.
And another one below this.
Thank you!
The remove_mepty_lines
function in the code snippet above can also be rewritten like this (it’s a little bit longer but easier to debug):
def remove_empty_lines(text):
lines = text.split('\n')
non_empty_lines = []
for line in lines:
if line.strip() != '':
non_empty_lines.append(line)
return '\n'.join(non_empty_lines)
Using the filter() Function
In this approach, the filter()
function is used along with a lambda function to filter out the empty lines. The lambda function checks if the line, after stripping the leading and trailing whitespace, is not an empty string.
Example:
input_string = """
He thrusts his fists
against the posts
and still insists he sees the ghosts
I am a fish
"""
def remove_empty_lines(text):
lines = text.split('\n')
non_empty_lines = filter(lambda line: line.strip() != '', lines)
return '\n'.join(non_empty_lines)
print(remove_empty_lines(input_string))
Output:
He thrusts his fists
against the posts
and still insists he sees the ghosts
I am a fish
Using Regular Expressions
This approach employs regular expressions (re
module) to remove empty lines. It replaces multiple consecutive newline characters with a single newline character.
Example:
import re
input_string = """
One Two Three Four
Five Six Seven Eight
Nine Ten Eleven Twelve
"""
def remove_empty_lines(text):
return re.sub(r'\n+', '\n', text).strip('\n')
print(remove_empty_lines(input_string))
Output:
One Two Three Four
Five Six Seven Eight
Nine Ten Eleven Twelve
That’s it. Happy coding & have a nice day!