A web server is a program that listens for requests from clients (such as web browsers) and sends back responses (such as web pages, JSON data, or files). aiohttp
is a library that provides asynchronous HTTP client and server functionality for Python. It allows you to write concurrent code using coroutines and the async/await
syntax.
In this example-based, step-by-step tutorial, I will explain how to create a simple web server with aiohttp
and Python. Without any further ado, let’s get started!
The Steps to Implement a Web Server
Step 1: Install aiohttp
:
pip install aiohttp
Then import web
from the module:
from aiohttp import web
Step 2: Create an application object with the web.Application
class:
app = aiohttp.web.Application()
Step 3: Define some routes and handlers
A route is a mapping between a URL path and a handler function that will process the request and return a response. A handler is a coroutine function that takes a request object as an argument and returns a response object.
In this example, we’ll define 2 routes
/
: The root path. The corresponding handler function will return a simple HTML page./api
: The API path. Its handler function will return some JSON data.
To register a route to the web server, we use the web.add_routes()
method. Let’s see the code below for more clarity:
# Define a handler for the root path
async def root_handler(request):
return web.Response(
text="<h1>Welcome to Sling Academy!</h1>", content_type="text/html"
)
# Add the root route and its handler to the app
app.add_routes([web.get("/", root_handler)])
# Define a handler for the /api path
async def api_handler(request):
return web.json_response(
{"status": 200, "message": "Welcome to the Sling Academy API!"}
)
# Add the /api route and its handler to the app
app.add_routes([web.get("/api", api_handler)])
Step 4: Run the application using the aiohttp
web server.
You can use the run_app()
function from the aiohttp
module to start the web server and run the application on a given host and port. For example, you can run the application on localhost
and port 8080
like this:
aiohttp.web.run_app(app, host="localhost", port=8080)
The Complete Code
Here’s the final source code that combines all the steps we’ve seen in the previous section:
# SlingAcademy.com
# main.py
from aiohttp import web
# Create an instance of the web application
app = web.Application()
# Define a handler for the root path
async def root_handler(request):
return web.Response(
text="<h1>Welcome to Sling Academy!</h1>", content_type="text/html"
)
# Add the root route and its handler to the app
app.add_routes([web.get("/", root_handler)])
# Define a handler for the /api path
async def api_handler(request):
return web.json_response(
{"status": 200, "message": "Welcome to the Sling Academy API!"}
)
# Add the /api route and its handler to the app
app.add_routes([web.get("/api", api_handler)])
# Run the app
web.run_app(app, host="localhost", port=8080)
You execute the code like this:
python main.py
Or:
python3 main.py
Then go to http://localhost:8080
with your favorite web browser. The result looks as follows:

You can also go to http://localhost:8080/api
to check the JSON response:
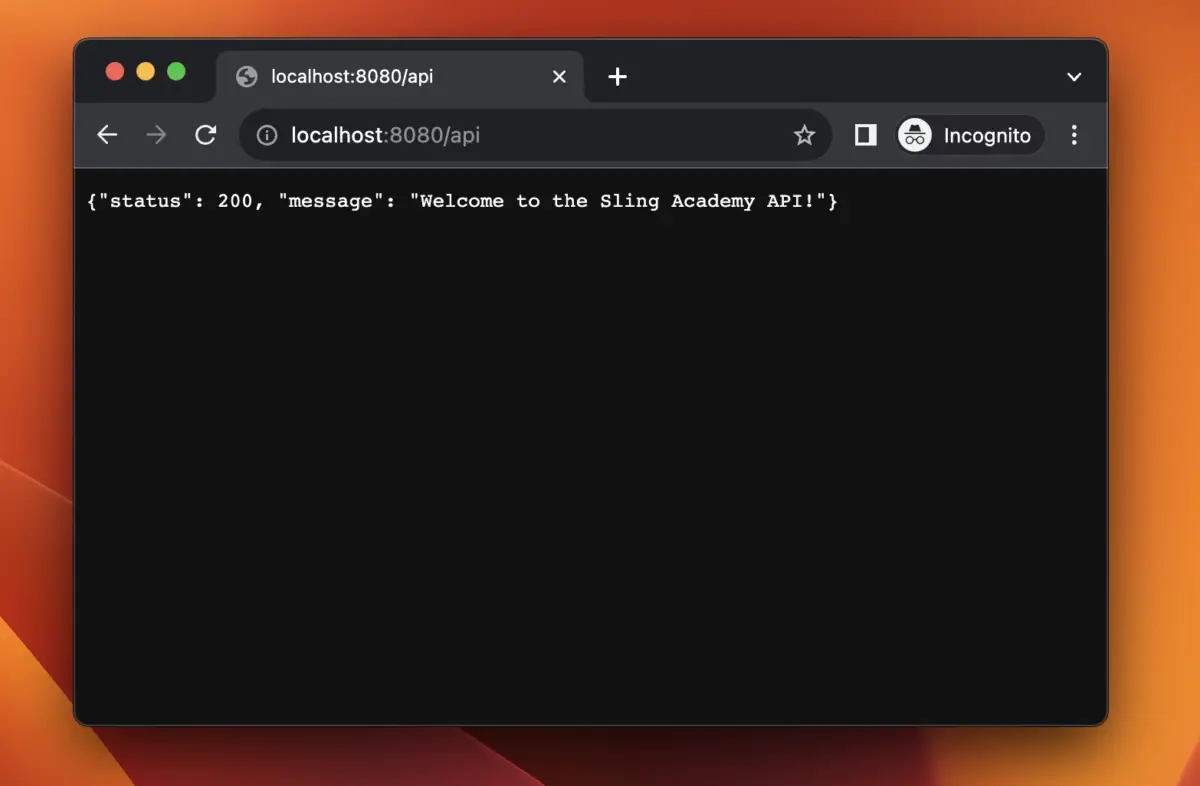
That’s it. Happy coding & have a nice day!