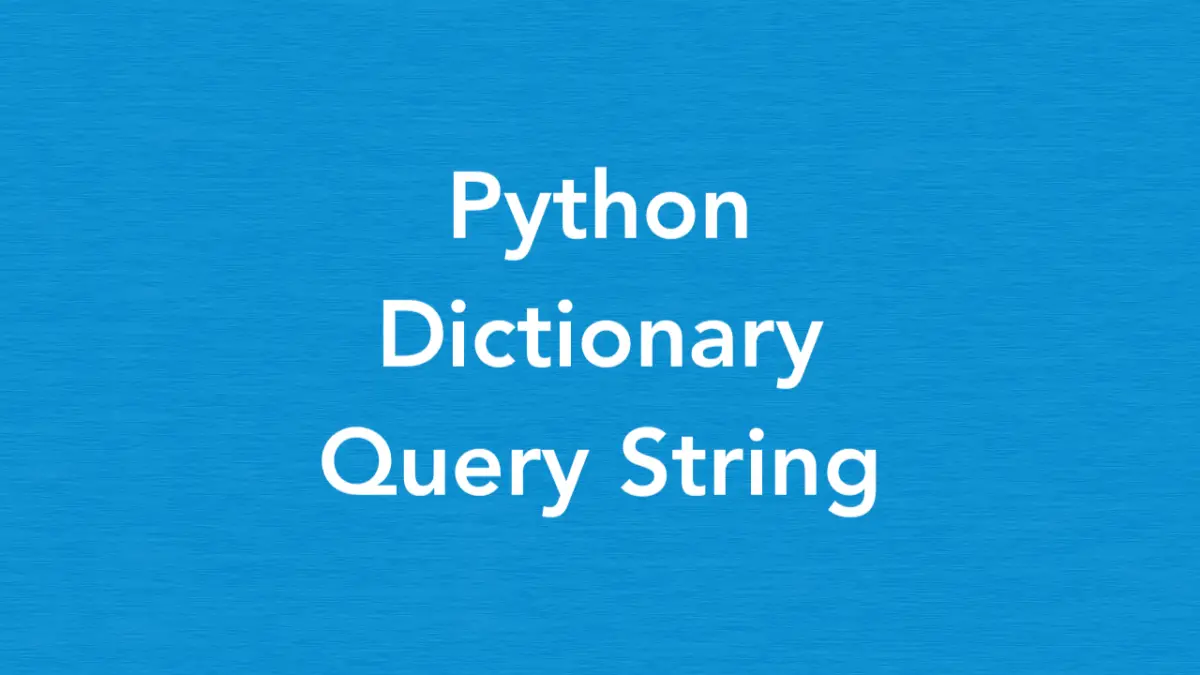
What is a Query String?
A query string is a part of a URL that follows the ?
symbol and contains key-value pairs separated by &
symbols. It is used to pass data to a web server as parameters. The query string consists of parameter names and their corresponding values.
An example of a URL with a query string:
https://www.slingacademy.com/search?q=python&category=tutorials&page=1
In this example, the query string starts with the ?
symbol and contains three key-value pairs: q=python
, category=tutorials
, and page=1
. Each key-value pair is separated by &
. The values in the query string are URL-encoded, which means special characters are replaced with their corresponding percent-encoded values. The web server can parse the query string to extract the parameters and use them for processing the request.
This concise, example-based article will walk you through a couple of different ways to convert a dictionary into a query string in Python. Without any further ado, let’s get started.
2 Ways to Convert a Dictionary to a Query String
Using urllib.parse.urlencode()
You can use the urllib.parse.urlencode()
function from the urllib.parse
module to encode the dictionary into a query string. This approach is simple and straightforward.
Example:
import urllib.parse
params = {"q": "python", "category": "tutorials", "page": 1}
# Encode the query string
query_string = urllib.parse.urlencode(params)
# Append the query string to the base URL
url = "https://www.slingacademy.com.com/search?" + query_string
print(url)
Output:
https://www.slingacademy.com.com/search?q=python&category=tutorials&page=1
Manual String Concatenation
An alternative solution is to iterate over the dictionary items and manually concatenate the key-value pairs into a query string. The step-by-step process is as follows:
- Create an empty list to store the key-value pairs.
- Iterate over the dictionary items using a loop.
- Encode each key-value pair and append it to the list.
- Join the list elements with
&
to form the query string. - Append the query string to the base URL if necessary.
Code example:
params = {"q": "python", "category": "tutorials", "page": 1}
# Create an empty list to store key-value pairs
key_value_pairs = []
# Iterate over the dictionary items
# and append encoded key-value pairs to the list
for key, value in params.items():
key_value_pairs.append(f"{key}={value}")
# Join the list elements with "&" to form the query string
query_string = "&".join(key_value_pairs)
# Append the query string to the base URL
url = "https://www.slingacademy.com/search?" + query_string
print(url)
Output:
https://www.slingacademy.com/search?q=python&category=tutorials&page=1
This approach is more manual work and error-prone compared to using built-in functions.