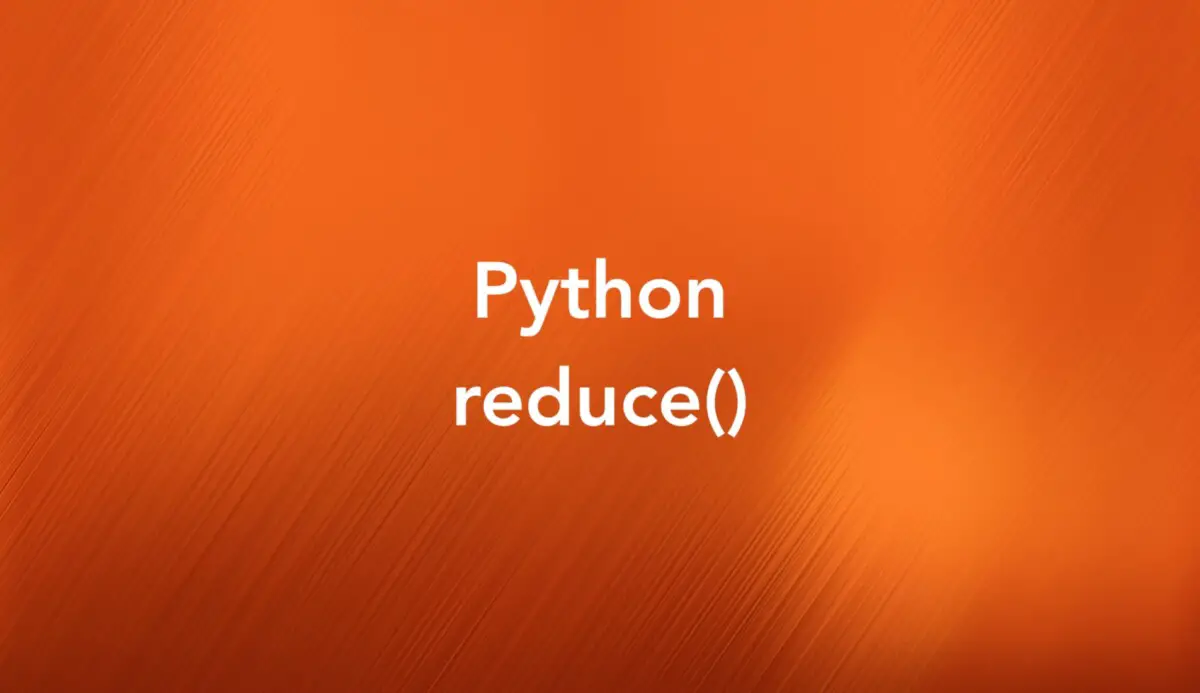
Overview
The reduce()
function in Python is part of the functools
module and is used to perform a specified function on a sequence of elements, reducing it to a single value. It takes two parameters: the function to be applied and the iterable sequence to be reduced.
Syntax:
reduce(function, iterable)
Parameters:
function
: The function to be applied. It should take two arguments and return a single value. The function is applied cumulatively to the items of the iterable from left to right.iterable
: The sequence of elements to be reduced.
In general, the reduce()
function is mostly used when working with lists.
Examples
Some examples of using the reduce()
function in practice, in order from basic to advanced.
Summing a list of numbers
The reduce()
function can help us easily calculate the sum of all numbers in a list:
from functools import reduce
numbers = [2023, 2024, 2025, 2026, 2027]
sum = reduce(lambda x, y: x + y, numbers)
print(sum) # 10125
Flattening a list of lists
You can use the reduce()
function to flatten a list of lists into a single list with only a single line of code:
from functools import reduce
nested_list = [[1, 2], [3, 4], [5, 6]]
flat_list = reduce(lambda x, y: x + y, nested_list)
print(flat_list) # Output: [1, 2, 3, 4, 5, 6]
Finding the longest string
Let’s say we have a list of strings. What we need to do is to find the longest string from there. The reduce()
function can help us get the job done without spending too much effort:
from functools import reduce
strings = ["dog", "slingacademy.com","turtle", "dark hole", "banana"]
longest = reduce(lambda x, y: x if len(x) > len(y) else y, strings)
print(longest) # slingacademy.com
Finding the minimum and maximum values
Here’s the code:
from functools import reduce
numbers = [12, 45, 23, 67, 9]
minimum = reduce(lambda x, y: x if x < y else y, numbers)
print(minimum) # Output: 9
maximum = reduce(lambda x, y: x if x > y else y, numbers)
print(maximum) # Output: 67
That’s it. The tutorial ends here. Happy coding & have a nice day!