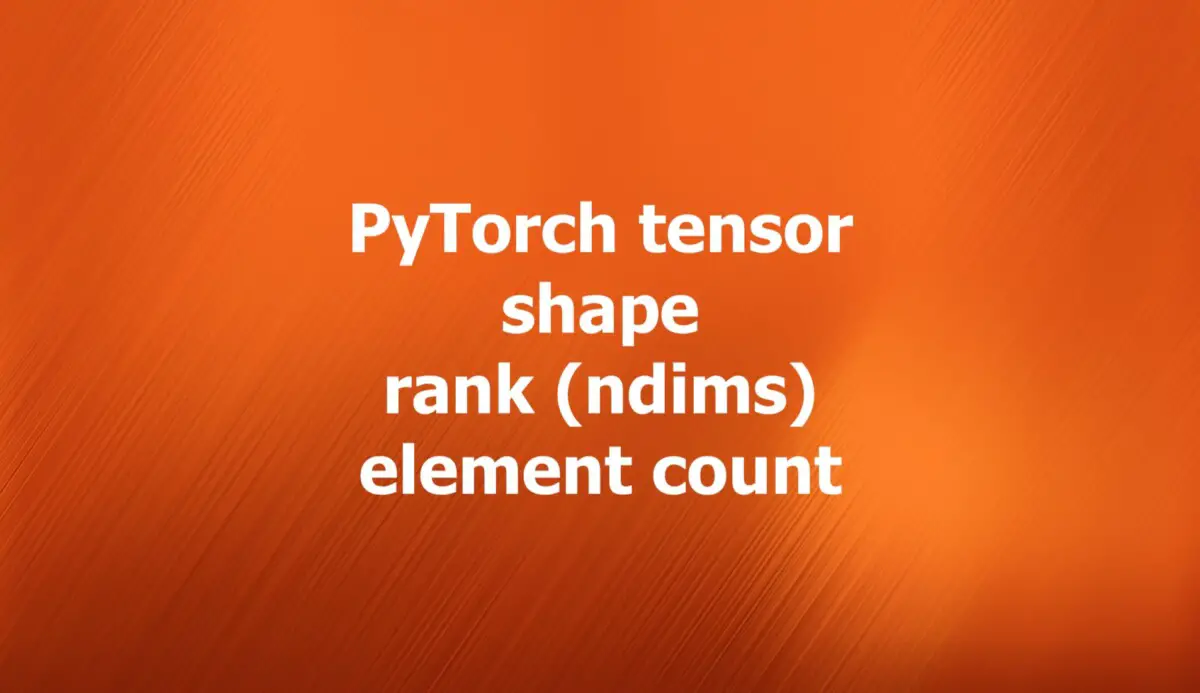
The shape of a PyTorch tensor
The shape of a PyTorch tensor is the number of elements in each dimension. You can use the shape attribute or the size() method to get the shape of a tensor as a torch.Size object, which is a subclass of tuple. You can also pass an optional argument dim to the size() method to know the size of a specific dimension as an int.
Example:
import torch
t = torch.rand(9, 7, 5)
print(f"The shape of the tensor is: {t.shape}")
print(f"The size of the tensor is: {t.size()}")
# print the size of the second dimension
print(f"The size of the second dimension is: {t.size(1)}")
Output:
The shape of the tensor is: torch.Size([9, 7, 5])
The size of the tensor is: torch.Size([9, 7, 5])
The size of the second dimension is: 7
In the context of the example above, the terms “shape” and “size” are the same.
The rank (ndims) of a PyTorch tensor
The rank of a PyTorch tensor is the number of dimensions present within the tensor. It is equal to the number of indices required to uniquely select each element of the tensor. Rank is also known as “order”, “degree”, or “ndims.”
You can use the ndim attribute or the dim() method to get the rank of a given tensor as shown in the example below:
import torch
t = torch.rand(9, 7, 5)
print(t.dim()) # 3
print(t.ndim) # 3
Counting elements in a PyTorch tensor
You can use the numel() method to count all elements in a PyTorch tensor. It returns the total number of elements in the input tensor as an int. The nelement() method is an alias for the numel() method, and will give you the same result.
Example:
import torch
t = torch.rand(9, 7, 5)
print(t.nelement())
print(t.numel())
Output:
315
315