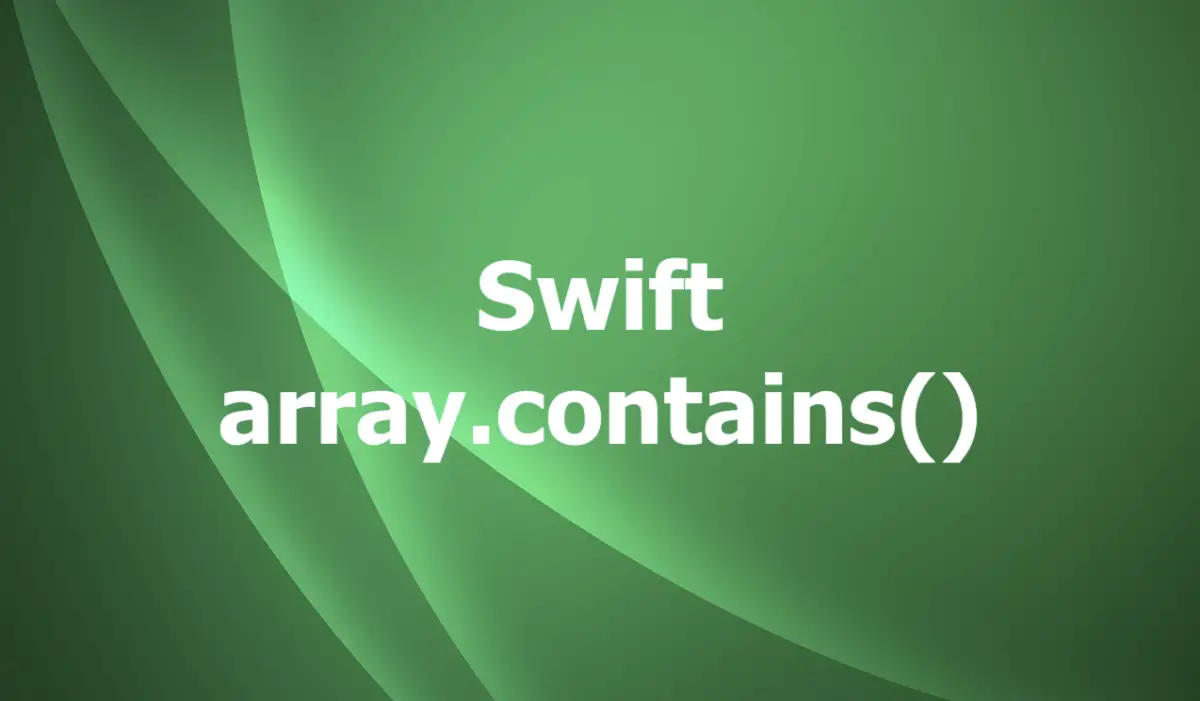
Table of Contents
- Overview
- Examples
- Basic example: Check if an array of strings contains a specific string
- Basic example: Using contains() with a closure
- Intermediate example: Check if an array of custom structs contains a specific struct
- Advanced example: Check if an array of custom classes contains a specific class instance using a custom equality function
- Conclusion
Overview
The array contains() method in Swift is a method that checks whether an array contains a specific element or not. This method has two variants.
Value version: This version takes an element as an argument and returns true if the element is found in the array and false if not. The element must conform to the Equatable protocol:
array.contains(element)
Closure version: This version takes a closure as an argument and returns true if the closure returns true for any element in the array and false otherwise. The closure takes an element as a parameter and returns a Boolean value:
array.contains { element in
// some condition involving element
}
Words might be boring and not-easy-to-understand. Let’s get our hands dirty by writing some code.
Examples
Basic example: Check if an array of strings contains a specific string
The code:
let fruits = ["apple", "banana", "orange", "grape"]
let fruit = "orange"
if fruits.contains(fruit) {
print("The array contains \(fruit).")
} else {
print("The array does not contain \(fruit).")
}
Output:
The array contains orange.
Basic example: Using contains() with a closure
Suppose you have an array of numbers and you want to check if any of them is divisible by 5. You can use the closure version of the contains() method like this:
let numbers = [12, 34, 56, 78, 90]
let divisibleByFive = numbers.contains { number in
number % 5 == 0
}
print(divisibleByFive)
Output:
true
Intermediate example: Check if an array of custom structs contains a specific struct
The code:
struct Person: Equatable {
let name: String
let age: Int
}
let people = [
Person(name: "Mr. Moneybags", age: 200),
Person(name: "Sling Academy", age: 69),
Person(name: "John Doe", age: 33),
]
let person = Person(name: "John Doe", age: 33)
if people.contains(person) {
print("The array contains \(person.name).")
} else {
print("The array does not contain \(person.name).")
}
Output:
The array contains John Doe.
Advanced example: Check if an array of custom classes contains a specific class instance using a custom equality function
The code:
class Animal: Equatable {
let name: String
let legs: Int
init(name: String, legs: Int) {
self.name = name
self.legs = legs
}
// Define a custom equality function for Animal class
static func == (lhs: Animal, rhs: Animal) -> Bool {
return lhs.name == rhs.name && lhs.legs == rhs.legs
}
}
let animals = [
Animal(name: "Dog", legs: 4),
Animal(name: "Cat", legs: 4),
Animal(name: "Bird", legs: 2),
]
let animal = Animal(name: "Cat", legs: 4)
if animals.contains(animal) {
print("The array contains \(animal.name).")
} else {
print("The array does not contain \(animal.name).")
}
Output:
The array contains Cat.
Conclusion
The contains() method is used widely in the world of iOS development. Mastering it can help you save a lot of time because, like it or not, you will need it quite often. If you have any questions, please leave a comment. Happy coding and good luck!