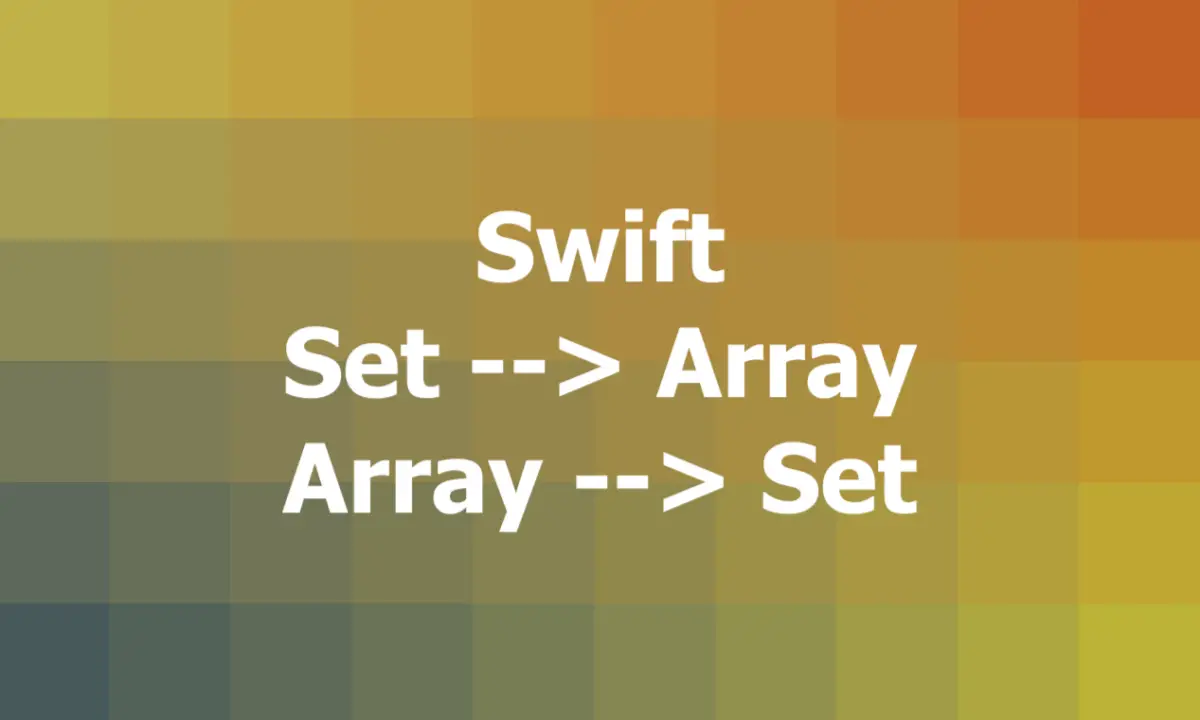
Converting a set to an array
In Swift, there are a couple of different ways to turn a set into an array. Let’s explore them one by one.
Using the Array initializer
To convert a given set to an array, you can use the Array initializer that takes a sequence as an argument. This initializer creates an array containing the elements of the sequence.
Example:
let numbers: Set = [1, 2, 3, 4]
let numbersArray = Array(numbers)
print(type(of: numbersArray))
Output:
Array<Int>
Note that the order of the elements in the array may vary sets are unordered collections. If you want the array elements have a consistent order, see the next approach.
Using the sorted() method
You can call the sorted()
method on the input set to create an array with the elements sorted in ascending order. This method returns an array that is the result of sorting the elements of the set according to a given predicate.
Example:
let words: Set = ["Sling", "Academy", "Swift", "Hello", "Buddy"]
let wordsArray = words.sorted()
print(wordsArray)
Output:
["Academy", "Buddy", "Hello", "Sling", "Swift"]
Converting an array into a set
To convert an array into a set in Swift, you can use the Set
initializer that takes a sequence as an argument. This initializer creates a set containing the elements of the sequence.
Example:
let myArray = ["sling", "academy", "swift", "dog", "dog", "dog"]
let mySet = Set(myArray)
print(mySet)
Output:
["academy", "swift", "dog", "sling"]
There are some important things you should be aware of when converting a Swift array to a set:
- The elements in the array must conform to the
Hashable
protocol. This means that they must have a unique hash value that can be used to compare them for equality. - The elements in the set will be unique. If the array contains any duplicate elements, they will be removed when converting to a set.
- The elements in the set will be unordered. If the order of the elements in the array matters, you may want to use an array instead of a set.
That’s it. The tutorial reaches its end here. Happy coding and have a nice day, my friend.