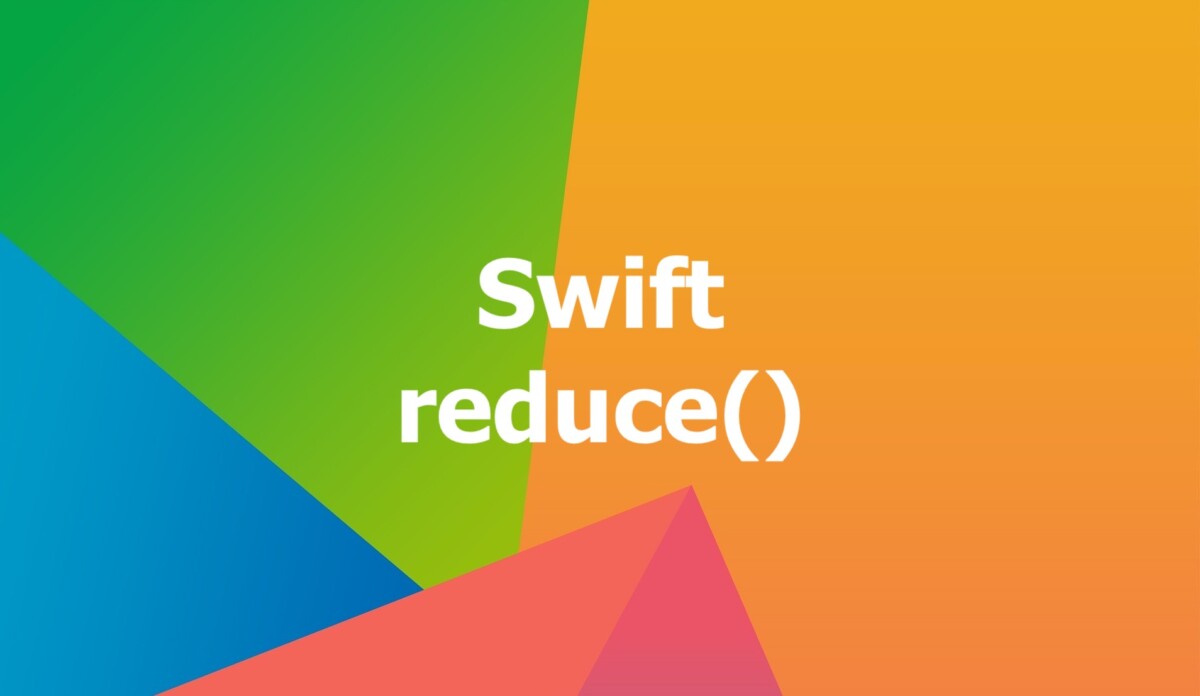
Prologue
Swift is a modern, powerful, and expressive programming language that has become the go-to choice for iOS development. One of the key features of Swift is its support for functional programming paradigms, which help developers write clean, concise, and efficient code.
The reduce() method is a higher-order function that belongs to the Sequence protocol in Swift. It’s a powerful method that allows you to transform a sequence (such as an array) into a single value by iteratively combining each element with an accumulator.
In this comprehensive article, we’ll dive deep into the reduce() method, exploring its syntax, parameters, and real-world use cases with code examples.
Syntax and parameters
The reduce() method is a higher-order function that takes two arguments: an initial value and a closure that combines the accumulator and current element.
The concise syntax:
reduce<Result>(_ initialResult: Result, _ nextPartialResult: (Result, Element) throws -> Result) rethrows -> Result
The reduce() method is generic over both the sequence’s elements and the result type. The Result type represents the accumulator, which is the running total or combined value being built up during the iteration.
The closure, denoted by nextPartialResult, specifies how to combine the accumulator and the current element. It takes two arguments: the current accumulator value and the current element of the sequence. It returns an updated value for the accumulator.
Examples
Let’s look at some practical examples of using the reduce() method in Swift. These examples will illustrate some common use cases and demonstrate the method’s versatility (and its usefulness, of course). I intend to arrange these examples in order from simple to complex, from easy to difficult (although this is subjective).
Summing up the elements of an array
One of the most straightforward applications of reduce() is summing up the elements of an array.
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
let sum = numbers.reduce(0) { accumulator, currentElement in
accumulator + currentElement
}
print(sum)
Output:
45
The choice of the initial value for the accumulator is crucial, as it determines the starting point for the reduction process. If you provide an inappropriate initial value, you might end up with incorrect results or runtime errors. When calculating the sum or product of an array of numbers, make sure to use 0 and 1 as initial values, respectively.
Finding the product of an array of numbers
Another common use case for reduce() is finding the product of an array of numbers. Let’s say we have the following array of integers:
let numbers = [1, 2, 3, 4, 5]
We can use the reduce() method to calculate the product of the elements like this:
let numbers = [1, 2, 3, 4, 5]
let product = numbers.reduce(1) { accumulator, currentElement in
accumulator * currentElement
}
print(product)
Output:
120
Concatenating an array of strings
The reduce() method can also be used to concatenate an array of strings. Suppose that we have the following array of strings:
let words = ["Sling", "Academy", "is", "the", "best", "place", "to", "learn", "Swift"]
We can use the reduce() method to concatenate the elements like this:
let words = ["Sling", "Academy", "is", "the", "best", "place", "to", "learn", "Swift"]
let sentence = words.reduce("") { accumulator, currentElement in
accumulator + " " + currentElement
}
print(sentence)
Output:
Sling Academy is the best place to learn Swift
Using reduce() with nested arrays
Let’s say we have a nested array of integers:
let nestedArray = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
We can use the reduce() method to flatten the nested array and calculate the sum of all the elements like this:
let nestedArray = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
let sum = nestedArray.reduce(0) { accumulator, currentArray in
accumulator + currentArray.reduce(0, +)
}
print(sum)
Output:
45
See also: Working with Multidimensional Arrays in Swift.
Combining arrays of dictionaries
Suppose we have an array of dictionaries, where each dictionary represents a product with a name and price:
let products = [
["name": "Apple", "price": 1.5],
["name": "Banana", "price": 2.0],
["name": "Carrot", "price": 1.0]
]
We can use the reduce() method to calculate the total price of all the products like this:
let products = [
["name": "Apple", "price": 1.5],
["name": "Banana", "price": 2.0],
["name": "Carrot", "price": 1.0],
]
let totalPrice = products.reduce(0.0) { accumulator, currentProduct in
accumulator + (currentProduct["price"] as! Double)
}
print(totalPrice)
Output:
4.5
Epilogue
The reduce() method is a powerful and versatile tool for working with arrays in Swift. By understanding its syntax, parameters, and practical use cases, you can write more efficient and expressive code for your iOS apps.
If you have any questions about the reduce() method, feel free to leave a comment. We’re more than happy to hear from you. Happy coding & have a nice day!