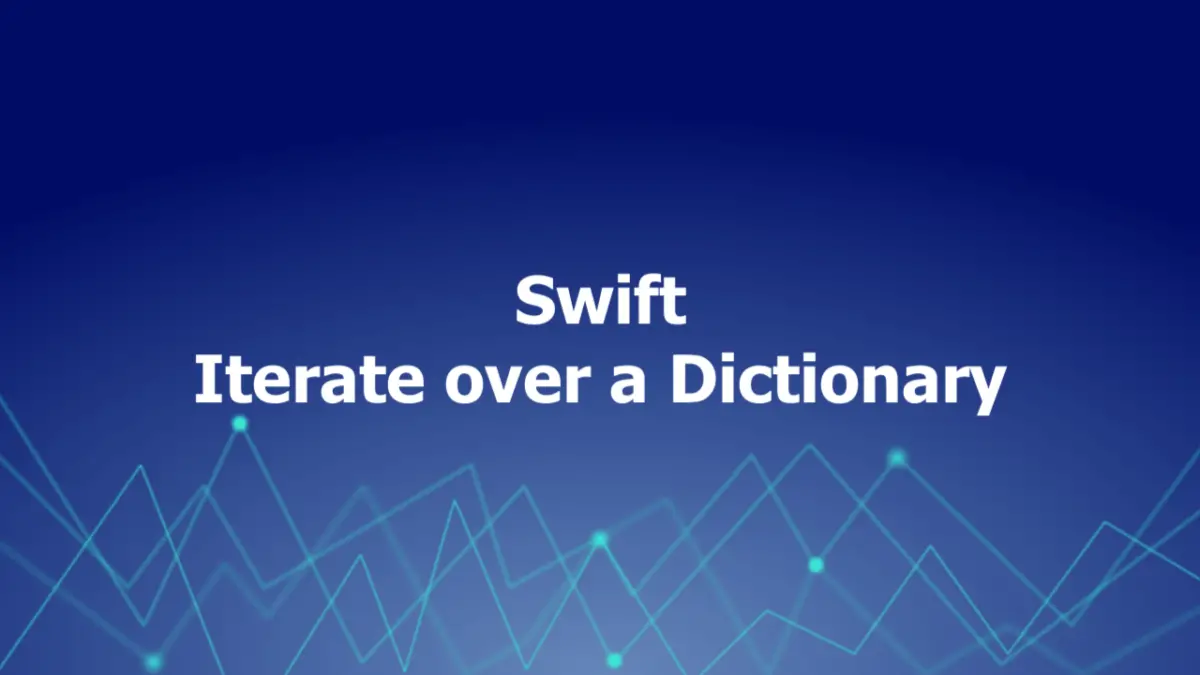
Using a for-in loop
In Swift, you can use a for-in
loop to iterate over the key-value pairs of a dictionary. Each item in the dictionary is returned as a (key, value)
tuple, and you can decompose the tuple’s members as explicitly named constants for use within the body of the loop.
Example:
// a dictionary of employees and their salaries
var employees = [
"Andy": 5000,
"Bobby": 4000,
"Cosy": 3000,
"Dondy": 4000,
"Eva": 5500,
]
for (name, salary) in employees {
print("\(name) has a salary of \(salary)")
}
Output:
Dondy has a salary of 4000
Andy has a salary of 5000
Bobby has a salary of 4000
Cosy has a salary of 3000
Eva has a salary of 5500
Using the forEach() method
The forEach()
method can be called on a dictionary to perform a closure on each key-value pair. The closure takes a (key, value) tuple as its argument and does not return any value.
Example:
// a dictionary of employees and their salaries
var employees = [
"Andy": 5000,
"Bobby": 4000,
"Cosy": 3000,
"Dondy": 4000,
"Eva": 5500,
]
employees.forEach { name, salary in
print("\(name) has a salary of \(salary)")
}
Output:
Dondy has a salary of 4000
Andy has a salary of 5000
Bobby has a salary of 4000
Cosy has a salary of 3000
Eva has a salary of 5500
Using the enumerated() method
You can also use the enumerated()
method on a dictionary to access its index and element as a tuple. The index is an integer that starts from zero and increases by one for each element. The element is another tuple that contains the key and value of the dictionary item.
Example:
// a dictionary of employees and their salaries
var employees = [
"Andy": 5000,
"Bobby": 4000,
"Cosy": 3000,
"Dondy": 4000,
"Eva": 5500,
]
for (index, element) in employees.enumerated() {
print("Item \(index): \(element)")
}
Output:
Item 0: (key: "Andy", value: 5000)
Item 1: (key: "Bobby", value: 4000)
Item 2: (key: "Dondy", value: 4000)
Item 3: (key: "Eva", value: 5500)
Item 4: (key: "Cosy", value: 3000)
Using the keys and values property
If you want to access only the keys or values of a dictionary, just use the keys
or values
property, respectively. These properties return a collection of the keys
or values
that can be iterated over with a for-in
loop.
Example:
// a dictionary of employees and their salaries
var employees = [
"Andy": 5000,
"Bobby": 4000,
"Cosy": 3000,
"Dondy": 4000,
"Eva": 5500,
]
for name in employees.keys {
print(name)
}
Output:
Andy
Eva
Bobby
Cosy
Dondy
Using the map() method
Example:
let dict1 = [
"key1": "value1",
"key2": "value2",
"key3": "value3",
]
_ = dict1.map { (key, value) in
print("\(key) : \(value)")
}
Output:
key2 : value2
key3 : value3
key1 : value1