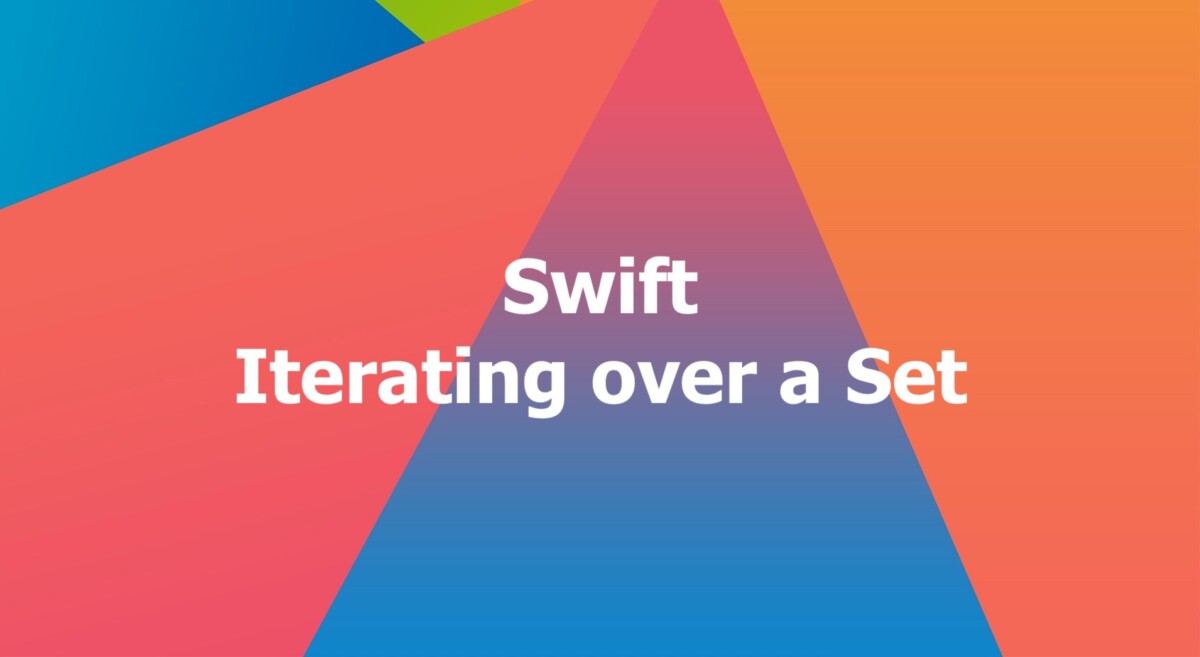
In Swift, there are two main ways to iterate through a set. The first approach is to use a for-in
loop, and the second one is to use the forEach()
method. You can also use some high-order functions that work with collections, such as map()
, reduce()
, or filter()
to perform various operations on a given set.
Using a for-in loop
Example:
let mySet = Set(["Sling", "Academy", "Swift", "iOS"])
for element in mySet {
print(element)
}
Output:
Sling
Academy
Swift
iOS
The order of the elements is not guaranteed because sets are unordered collections.
Using the forEach() method
Example:
let numbers: Set<Int> = [1, 2, 3, 4, 5]
numbers.forEach { element in
print(element)
}
Output:
3
2
1
4
5
You can also write the code in a more concise style like this:
let numbers: Set<Int> = [1, 2, 3, 4, 5]
numbers.forEach{ print($0)}
Once again, the order of the elements in the output is not guaranteed.
Iterating over a sorted set
Suppose you want to print the elements of a set in a consistent order then you can use the sorted()
method to create an array of sorted elements and then use either a for-in
loop or a forEach()
method on that array.
Example:
import Foundation
// create a set of words
var words: Set<String> = ["slingacademy.com", "swift", "ios", "programming"]
// create an array of sorted elements from the set
var sortedwords = words.sorted()
// iterate over the sorted array using a for-in loop
for word in sortedwords {
print(word)
}
// iterate over the sorted array using the forEach() method
sortedwords.forEach { word in
print(word)
}
Using high-order functions with a a set
Example:
// create a set of numbers
var numbers: Set<Int> = [1, 2, 3, 4, 5]
// use map() to create a new set with each element squared
var squaredNumbers = numbers.map { $0 * $0 }
print(squaredNumbers) // [16, 1, 9, 25, 4]
// use reduce() to create a single value by adding all the elements
var sum = numbers.reduce(0, +)
print(sum) // 15
// use filter() to create a new set with only the even elements
var evenNumbers = numbers.filter { $0 % 2 == 0 }
print(evenNumbers) // [2, 4]