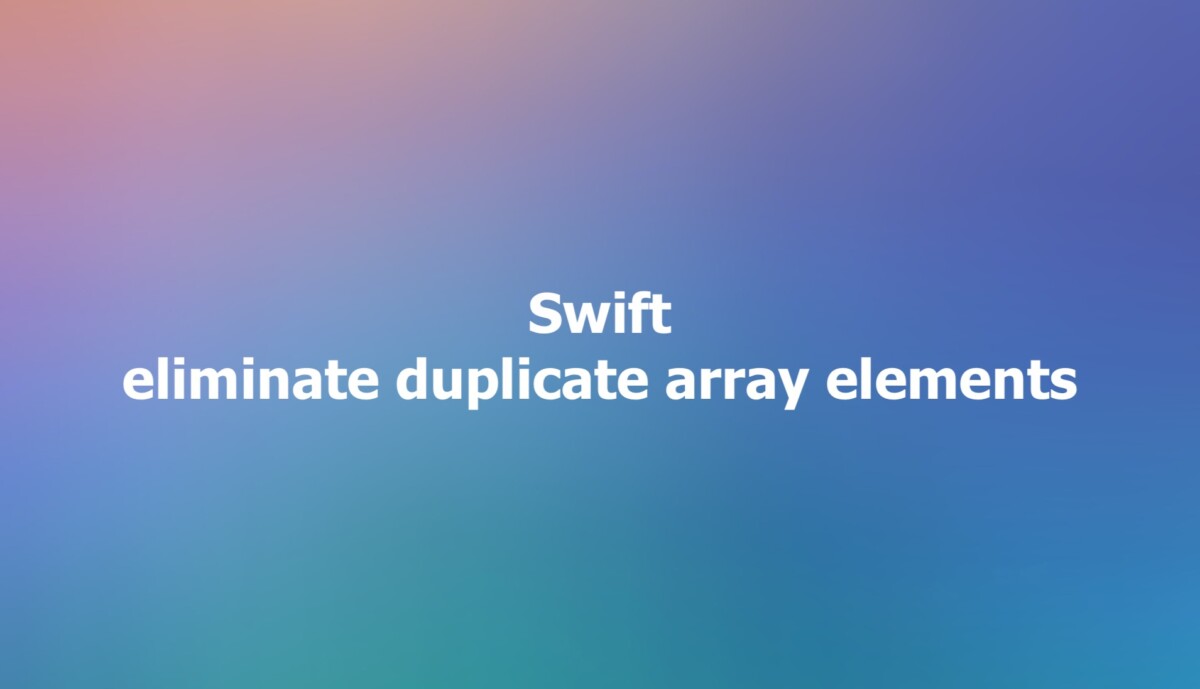
Overview
Swift is a powerful, intuitive, and efficient programming language that allows you to write clean, easy-to-read code. Arrays, a fundamental data structure in Swift, are used to store multiple values of the same type.
Duplicate array elements are items in an array that are identical to one or more other items in the array. Depending on the context of your project, duplicate elements can be a hindrance or even a bug, as they can cause incorrect calculations, inaccurate data representation, or inefficient memory usage.
This practical, example-based article will walk you through a couple of different ways to eliminate duplicate elements from a Swift array. Without any other further ado, let’s get started.
Using Set
A Set
is a collection type in Swift that stores unique elements in no particular order. By converting an array into a Set and then back into an array, you can quickly get rid of duplicates.
Example:
=let numbers = [1, 2, 3, 1, 3, 5, 6, 2, 5]
let uniqueNumbers = Array(Set(numbers))
print(uniqueNumbers)
// Output: [1, 5, 2, 3, 6]
Another example (creating an extension):
// declare an extension
extension Array where Element: Hashable {
func removingDuplicates() -> [Element] {
return Array(Set(self))
}
}
let numbers = [1, 2, 3, 4, 4, 5, 6, 6]
let uniqueNumbers = numbers.removingDuplicates()
print(uniqueNumbers)
// Output: [5, 2, 3, 1, 6, 4]
Keep in mind that converting an array to a Set will not maintain the original order of the elements. If you need to preserve the order, see other approaches in this article.
Using the Array.filter() method
Another technique for removing duplicates is to use the filter()
method, which allows you to create a new array by filtering the elements of the original array that meet specific criteria. In this case, the criteria would be to include only unique elements.
This method maintains the original order of the elements but may be less efficient than using a Set, as it requires iterating over the entire array.
Example:
let numbers = [1, 2, 3, 1, 3, 5, 6, 2, 5]
var uniqueNumbers: [Int] = []
_ = numbers.filter { item in
if uniqueNumbers.contains(item) {
return false
} else {
uniqueNumbers.append(item)
return true
}
}
print(results)
// Output: [1, 2, 3, 5, 6]
Defining a custom function
You can also create a custom function to remove duplicates from an array. This approach allows for more flexibility and customization, depending on your specific requirements.
Here’s an example of a custom function that removes duplicates while preserving the original order:
func removeDuplicates<T: Equatable>(from array: [T]) -> [T] {
var uniqueElements: [T] = []
for item in array {
if !uniqueElements.contains(item) {
uniqueElements.append(item)
}
}
return uniqueElements
}
let numbers = [1, 2, 3, 1, 3, 5, 6, 2, 5]
let uniqueNumbers = removeDuplicates(from: numbers)
print(uniqueNumbers)
// Output: [1, 2, 3, 5, 6]
This custom function uses a generic type to support arrays of any type that conforms to the Equatable protocol.
Conclusion
Removing duplicate elements from an array is a common task in Swift programming. In this tutorial, we’ve explored three methods for eliminating duplicates:
- Using Set: This method is generally the fastest and requires the least amount of code. However, it does not maintain the original order of the elements.
- Filter method: This method preserves the original order of the elements but may be slower than using a Set, as it requires iterating over the entire array.
- Custom function: This method provides the most flexibility and customization but may be less efficient than the other methods. It also requires more code and a deeper understanding of Swift.
Each method has its advantages and disadvantages, so it’s essential to consider your specific requirements when choosing the best approach for your apps.