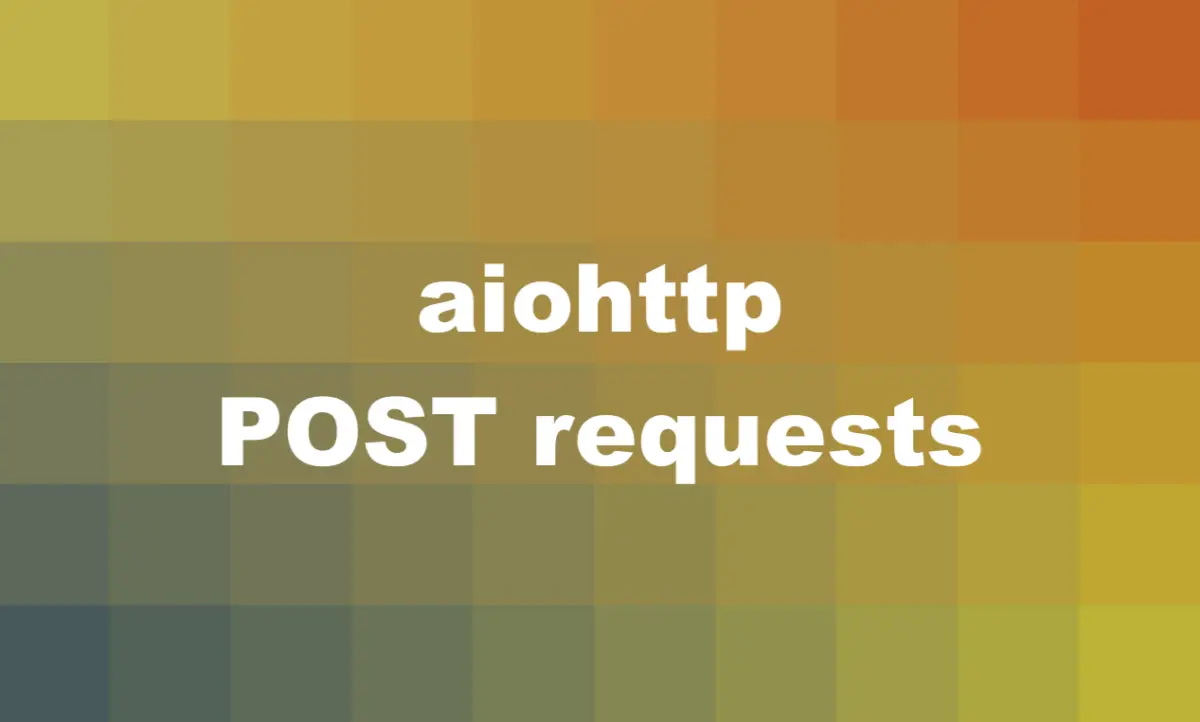
aiohttp
is a modern Python library that allows you to make http requests asynchronously with the async/await
syntax. In this concise and straightforward article, we’ll learn how to use this library to efficiently send POST requests to an API endpoint or a remote server. We’ll have a look at the fundamentals and then walk through a few examples of applying that knowledge in practice.
The Fundamentals
Before getting started, make sure you have aiohttp
installed:
pip install aiohttp
The session.post() method
To send POST requests with aiohttp
, you need to use the aiohttp.ClientSession()
function to create a session
object, and then call the post()
method on that session object. Below is the syntax of the session.post()
method:
session.post(url, *, data=None, json=None, **kwargs)
The parameters are:
url
: The URL of the request. It can be a string or a yarl.URL object. It is the only required parameter.data
: The data to send in the request body. It can be one of the following types:bytes
: A binary data object.str
: A text data object. It will be encoded using UTF-8 by default.dict
: A dictionary of key-value pairs. It will be encoded using theapplication/x-www-form-urlencoded
content type by default.aiohttp.FormData
: A multipart form data object. It can contain files and fields.async generator
: An asynchronous generator that yields binary data chunks.file-like object
: An object that supports theread()
method, such as an open file or aBytesIO
object.
json
: The JSON data to send in the request body. It can be any Python object that is serializable by thejson
module. It will be encoded using theapplication/json
content type by default.**kwargs
: Any other keyword arguments that are supported by theClientSession.request()
method, such asheaders
,params
,auth
,timeout
, etc.
The session.post()
method returns a ClientResponse
object, which represents the response from the server. You can access various attributes and methods of the response object, such as status
, headers
, text()
, json()
, etc.
The session.request() method
An alternative way to make POST requests is to use the session.request()
method with the first parameter is set to "POST"
:
session.request("POST", url, *, data=None, json=None, **kwargs)
Other parameters are the same as the session.post()
method.
Examples
In the following example, we’ll make some POST requests to this API endpoint:
https://api.slingacademy.com/v1/sample-data/products
You can post information about a fictional product to it with a name
(required), price
(required), description
(optional), and quantity
(optional).
Example 1: Sending JSON data
By using the async width
statement, you can create a session object and close it automatically. Below is the code:
# SlingAcademy.com
# This code uses Python 3.11.4
import asyncio
import aiohttp
# Import the pprint module to print the JSON response in a readable format
# This is a built-in module, so it does not need to be installed
from pprint import pprint
async def main(url):
# Create a session using the async with statement
async with aiohttp.ClientSession() as session:
# Make a POST request using the session.post() method
async with session.post(
url,
json={
"name": "Example Product",
"price": 99.99,
"description": "This is an example product.",
"quantity": 3,
},
) as response:
# Print the status code and the response text
print(f"Status: {response.status}")
pprint(await response.json())
# Run the main coroutine
url = "https://api.slingacademy.com/v1/sample-data/products"
asyncio.run(main(url))
Output:
Status: 201
{'message': 'product submitted successfully',
'product': {'created_at': '2023-08-19T19:35:52.666168+00:00',
'description': 'This is an example product.',
'id': 'test-123',
'name': 'Example Product',
'price': 99.99,
'quantity': 3},
'success': True}
If you don’t familiar with the pprint()
function in the code snippet above, see this article: Working with the pprint.pprint() function in Python (with examples).
Example 2: Setting headers
There are two ways to set headers when using the session.post()
method. You can either pass them as a dictionary to the headers
parameter of the session.post()
method, or you can set them as default headers for the session using the headers
parameter of the ClientSession
constructor.
# Set headers for a single request
headers = {'content-type': 'application/json'}
async with session.post(url, data=your_data,headers=headers) as response:
# Do something with the response
# Set headers for all requests in a session
headers = {'user-agent': 'The Wolf of Wallstreet'}
async with aiohttp.ClientSession(headers=headers) as session:
async with session.post(url, data=your_data) as response:
# Do something with the response
That’s it. Happy coding & enjoy your day!