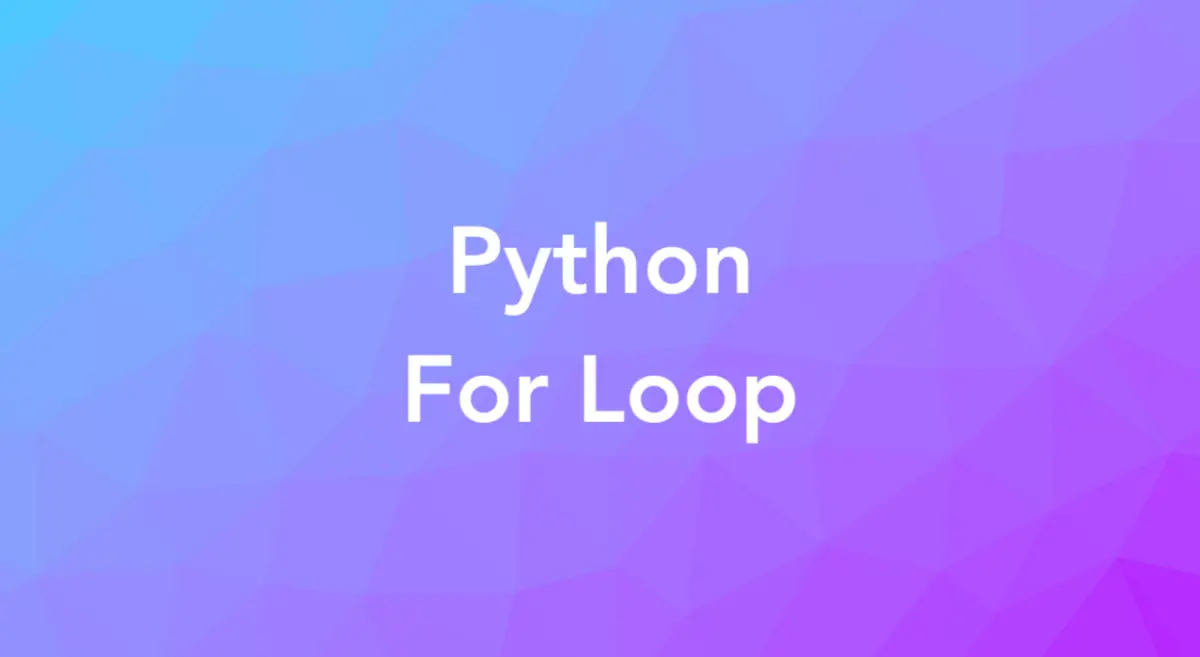
The basic
In Python, a for
loop is a type of loop that is used to iterate over a sequence of items, such as a list, a tuple, a dictionary, a set, or a string. A for
loop executes a block of code for each item in the sequence until the sequence is exhausted or a break
statement is encountered.
Syntax:
for item in sequence:
# do something with item
Explanation:
- The
for
keyword initiates the loop. item
is a variable that represents each item in the sequence for each iteration.sequence
is the sequence of items to iterate over.- The colon (
:
) indicates the start of the code block that will be executed for each iteration. - The indented block of code is the body of the loop that is executed for each item.
Basic example:
years = [2023, 2024, 2025, 2026, 2027]
for year in years:
print(f"Year: {year}")
Output:
Year: 2023
Year: 2024
Year: 2025
Year: 2026
Year: 2027
Another example:
text = "Sling Academy"
for letter in text:
print(letter)
Output:
S
l
i
n
g
A
c
a
d
e
m
y
Using a “for” loop with the range() function
When using a for
loop with the range()
function, you can iterate over a sequence of numbers within a specified range. The range()
function generates a sequence of numbers based on the provided start, stop, and step values.
The syntax of the range()
function is as follows:
range(start, stop, step)
Where:
start
(optional) specifies the starting value of the range (inclusive). If not provided, it defaults to0
.stop
specifies the ending value of the range (exclusive). The generated sequence will contain numbers up to, but not including, thestop
value.step
(optional) specifies the increment between each number in the sequence. If not provided, it defaults to1
.
Example:
for num in range(5):
print(num)
Output:
0
1
2
3
4
This example Iterates over a range of numbers from 1 to 10 (exclusive), incrementing by 2:
for num in range(1, 10, 2):
print(num)
Output:
1
3
5
7
9
In case you want to iterate over a range of numbers in reverse order, just use a negative step like this:
for num in range(10, 0, -1):
print(num)
Output:
10
9
8
7
6
5
4
3
2
1
Using a “for” loop with the “break” statement
You can use the break
statement to stop a for
loop before it has run through all the items.
Example:
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
if x == "banana":
break
Output:
apple
banana
Using a “for” loop with the “continue” statement
The continue
statement stops the current iteration of a for
loop and continues with the next one.
Example:
fruits = ["apple", "banana", "cherry", "mango"]
for x in fruits:
if x == "banana":
continue
print(x)
Output:
apple
cherry
mango
Using a “for” loop with the “else” clause
You can use a for
loop with the else
clause to execute a block of code when the loop is finished.
Example:
for x in range(6):
print(x)
else:
print("Finally finished!")
Output:
0
1
2
3
4
5
Finally finished!