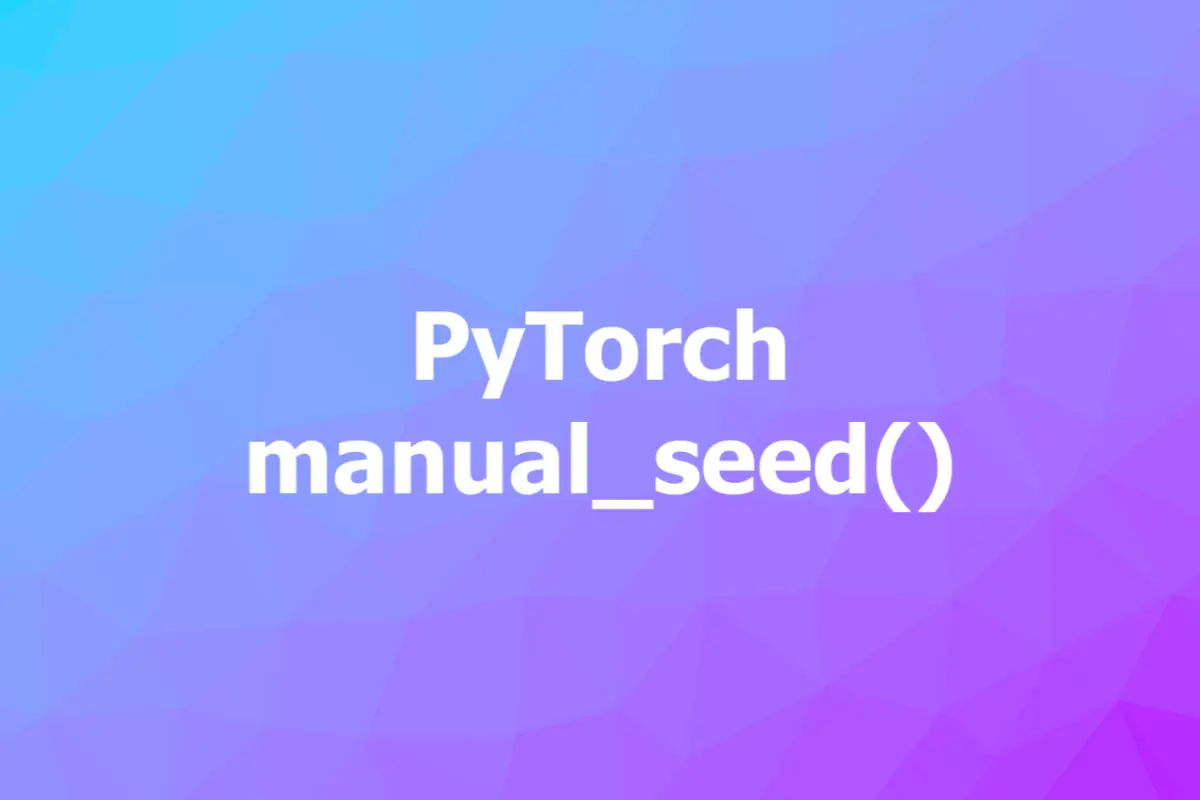
Why use torch.manual_seed()
torch.manual_seed() is a function that helps you control the randomness in PyTorch. A lot of times, you want to use random numbers in your code, such as when you create a tensor with torch.rand() or when you shuffle your data with torch.utils.data.RandomSampler(). Random numbers can make your code more interesting and fun, but they can also make it hard to repeat or compare your results. For example, if you run your code twice with different random numbers, you may get different outputs or errors.
To avoid this problem, you can use torch.manual_seed() to set a seed for the random number generator in PyTorch. A seed is like a secret code that tells PyTorch how to generate random numbers. If you use the same seed every time you run your code, you will get the same random numbers every time. This way, you can make your code more consistent and reliable (and it will be easier to discuss a task with other people).
Syntax
The general syntax of torch.manual_seed() is:
torch.manual_seed(seed)
Where seed is a positive integer or 0 that specifies the seed value for the random number generator in PyTorch. It is recommended to use a large and random value to avoid statistical bias.
In case you want to retrieve the initial seed value of the random generator in PyTorch, just call the torch.random.initial_seed() function:
my_seed = torch.random.initial_seed()
Examples
Setting a seed and creating a random tensor
The code:
import torch
# Set the seed to 1984
torch.manual_seed(1984)
# Create a random tensor of size 2x3
x = torch.rand(2, 3)
# Print the tensor
print(x)
Output:
tensor([[0.2415, 0.7049, 0.4789],
[0.6017, 0.4420, 0.1624]])
The result will be consistent no matter how many times you re-run your code.
Setting random seeds for specific operations
The code:
import torch
import torch.nn.functional as F
torch.manual_seed(99)
x = torch.randn(2, 2)
torch.manual_seed(456)
y1 = F.dropout(x, p=0.5, training=True)
torch.manual_seed(8888)
y2 = F.dropout(x, p=0.5, training=True)
print(y1)
print(y2)
Output:
tensor([[1.2254, -0.0000],
[-0.0000, -0.0000]])
tensor([[ 0.0000, -0.0000],
[-0.0000, -1.3331]])