What is a chi-square distribution?
A chi-square distribution is a statistical distribution that describes the sum of the squares of a set of independent standard normal random variables. It is a special case of the gamma distribution and is widely used in hypothesis testing, particularly in chi-square tests for goodness-of-fit, independence in contingency tables, and in variance analysis.
Key Points:
- Degrees of Freedom: The shape of the chi-square distribution is determined by its degrees of freedom (df), which equals the number of independent standard normal variables being summed. The distribution becomes more symmetric and approaches a normal distribution as the degrees of freedom increase.
- Non-Negativity: Values of a chi-square distribution are always non-negative, since they are based on squared quantities.
- Applications: It is used in chi-square tests, which are statistical tests that compare observed data with data expected based on a specific hypothesis. These tests are useful for determining whether there are significant differences between expected and observed data.
- Skewness: The distribution is skewed to the right, but the skewness decreases as the degrees of freedom increase.
Understanding the random.Generator.chisquare() method
The numpy.random.Generator.chisquare()
method plays a crucial role in generating random samples from a chi-square distribution. A deep dive into this function opens up powerful avenues for data analysis and simulation in scientific computing. In this tutorial, we’re going to explore the versatile use of the chisquare()
method in NumPy, backed with practical examples that span from basic to advanced applications, letting you harness the full potential of generating random chi-square distributed data.
Syntax:
generator.chisquare(df, size=None)
Parameters:
- df: float or array_like of floats. The degrees of freedom of the distribution. The parameter must be greater than 0.
- size: int or tuple of ints, optional. Specifies the shape of the returned array of random samples. If not provided, a single value is returned.
Returns:
- out: ndarray or scalar. Random samples drawn from a chi-square distribution.
Example 1: Basic Usage
In our first example, let’s generate a basic chi-square distributed random sample using NumPy:
import numpy as np
# Create a random generator
rng = np.random.default_rng()
# Generate a chi-square distributed sample
chi_square_sample = rng.chisquare(df=2, size=10)
print(chi_square_sample)
The output is an array of random numbers following a chi-square distribution with 2 degrees of freedom and a size of 10. Note that ‘df’ refers to degrees of freedom, a critical component in the distribution’s shape.
A possible output:
[2.6608669 0.37269299 0.04525142 1.38630627 1.1701559 0.33366615
1.78312699 0.49363823 0.49718952 0.31764432]
Example 2: Visualizing the Distribution
Moving to our second example, we’ll visualize the distribution of a large chi-square generated sample to understand its shape and spread:
import numpy as np
import matplotlib.pyplot as plt
# Create a random generator
rng = np.random.default_rng()
# Generate a large chi-square sample
chi_square_large = rng.chisquare(df=5, size=1000)
# Visualize the sample
plt.hist(chi_square_large, bins=30, density=True)
plt.title('Chi-Square Distribution Visualization')
plt.xlabel('Values')
plt.ylabel('Frequency')
plt.show()
Output (may vary, due to the randomness):
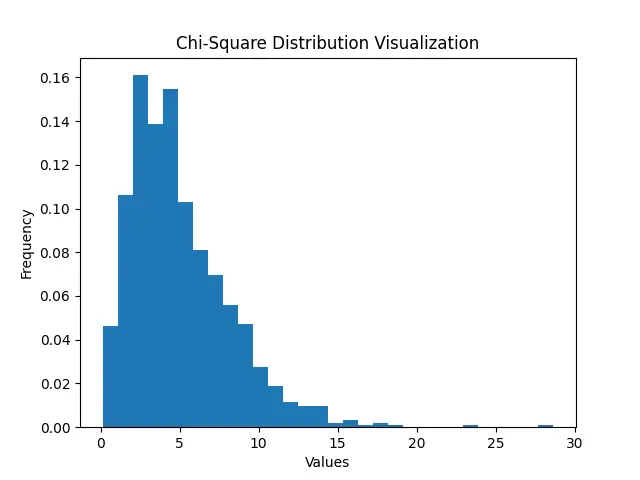
This histogram visually represents the chi-square distribution with 5 degrees of freedom, obtained from a thousand data points. Visualization like this is crucial for understanding the distribution’s characteristics.
Example 3: Reproducibility
To ensure that scientific research is reproducible, it’s essential to generate the same set of random numbers across different execution environments. The next example shows how to set a seed in the random generator:
import numpy as np
# Set a seed for reproducibility
seed = 123
rng = np.random.default_rng(seed)
# Reproducible chi-square sample
reproducible_sample = rng.chisquare(df=3, size=10)
print(reproducible_sample)
Output:
[0.78246232 6.36791338 4.93925129 1.21079075 1.71405454 2.54959597
5.97241235 5.2298229 7.45425566 1.72251141]
Using a seed ensures that every time you run your program, the generated chi-square distributed random numbers are the same, making your results reproducible and verifiable.
Example 4: Sampling for Hypothesis Testing
In this more sophisticated application, we’ll simulate chi-square distribution samples to perform a basic hypothesis testing:
import numpy as np
# Set a seed for reproducibility
seed = 123
rng = np.random.default_rng(seed)
# Assumed means for two categories
mean1, mean2 = 10, 15
# Generate samples
sample1 = rng.normal(loc=mean1, scale=5, size=100)
sample2 = rng.normal(loc=mean2, scale=5, size=100)
# Computing chi-square statistic
from scipy.stats import chisquare
stat, p = chisquare([sample1.mean(), sample2.mean()])
print('Chi-square statistic:', stat, '\n', 'P-value:', p)
Output:
Chi-square statistic: 0.9963895262724114
P-value: 0.3181857167668704
This example highlights the applicability of the chisquare()
method in generating data for statistical tests, allowing us to conduct hypothesis testing with generated samples.
Example 5: Advanced Data Simulation
For our final example, we will delve into more complex simulations involving chi-square distributions, useful in fields such as finance and engineering:
import numpy as np
# Set a seed for reproducibility
seed = 123
rng = np.random.default_rng(seed)
# Projected risk assessment with chi-square distributed variables
risk_levels = rng.chisquare(df=4, size=1000)
# Generate future scenarios
future_scenarios = 100 * (1 + 0.05 * np.sqrt(risk_levels))
print(future_scenarios[:10])
Output:
[105.86546764 114.04203934 112.56843475 106.97362058 108.03257183
109.47439343 113.65303893 112.8845525 115.04999861 108.04884148]
This showcases how the chisquare()
method can be an instrumental tool in simulating real-world scenarios, providing insights into future trends and risks.
Conclusion
The nump.random.Generator.chisquare()
method offers flexibility and power for generating chi-square distributed samples across a variety of applications. From basic random sample generation to complex simulations for financial analysis, understanding and utilizing this method broadens your capabilities in statistical computing and data analysis. Hopefully, this guide has illuminated the path towards leveraging the chi-square distribution in your computational work.