Introduction
The numpy.rot90()
function is a powerful tool provided by the NumPy library for rotating arrays by 90 degrees in the plane specified by axes. This versatile function supports multidimensional arrays and provides an easy-to-use interface for array manipulation. Throughout this tutorial, we will cover six practical examples to illustrate the usage of numpy.rot90()
, ranging from basic to advanced applications. Let’s begin by setting up your environment.
Understanding numpy.rot90()
Syntax:
numpy.rot90(m, k=1, axes=(0, 1))
Parameters:
- m: array_like. The input array to be rotated.
- k: int, optional. The number of times the array is rotated by 90 degrees. A positive value rotates counterclockwise, while a negative value rotates clockwise. The default is
1
. - axes: tuple of 2 ints, optional. The two axes that define the plane of rotation. The default is
(0, 1)
, which rotates the first plane of the array.
Returns:
- rotated_array: ndarray. The rotated array.
Example 1: Basic Array Rotation
Let’s start with the most straightforward example. We will create a 2D array and rotate it once (90 degrees).
import numpy as np
original_array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
rotated_array = np.rot90(original_array)
print('Original array:')
print(original_array)
print('\nRotated array:')
print(rotated_array)
Output:
Original array:
[[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
Rotated array:
[[3, 6, 9],
[2, 5, 8],
[1, 4, 7]]
Example 2: Specifying the Number of Rotations
In this example, let’s rotate the same array but this time by 270 degrees (equivalent to rotating it three times by 90 degrees).
import numpy as np
original_array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
rotated_array_270 = np.rot90(original_array, 3)
print('270 degrees rotated array:')
print(rotated_array_270)
Output:
270 degrees rotated array:
[[7, 4, 1],
[8, 5, 2],
[9, 6, 3]]
Example 3: Rotating Along Specific Axes
NumPy’s rot90() allows you to specify along which axes the rotation should occur. This is particularly useful for multi-dimensional arrays. Here, we’ll rotate a 3D array.
import numpy as np
original_3d_array = np.arange(27).reshape(3, 3, 3)
rotated_3d_array = np.rot90(original_3d_array, axes=(1, 2))
print('Original 3D array:')
print(original_3d_array)
print('\nRotated 3D array along axes (1, 2):')
print(rotated_3d_array)
Output:
Original 3D array:
[[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]],
[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]]]
Rotated 3D array along axes (1, 2):
[[[ 2, 5, 8],
[ 1, 4, 7],
[ 0, 3, 6]],
[[11, 14, 17],
[10, 13, 16],
[ 9, 12, 15]],
[[20, 23, 26],
[19, 22, 25],
[18, 21, 24]]]
Example 4: Combining rot90() with Other NumPy Functions
To demonstrate the power of numpy.rot90() when used in combination with other NumPy functions, let’s perform an operation where we first rotate an array and then apply a flipping operation.
import numpy as np
original_array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
flipped_and_rotated = np.flipud(np.rot90(original_array))
print('Flipped and rotated array:')
print(flipped_and_rotated)
Output:
Flipped and rotated array:
[[1, 4, 7],
[2, 5, 8],
[3, 6, 9]]
Example 5: Rotating Images
Image data can be represented as multi-dimensional arrays, making numpy.rot90()
an excellent tool for image rotation. Here’s an example using an image represented as a 3D array (considering RGB channels).
Note: This example requires installing scikit-image
package (that provides sample images for practice):
pip install scikit-image
Example:
import numpy as np
from skimage import data
import matplotlib.pyplot as plt
astro = data.astronaut()
rotated_astro = np.rot90(astro)
plt.imshow(rotated_astro)
plt.title('Rotated Image')
plt.show()
Output:
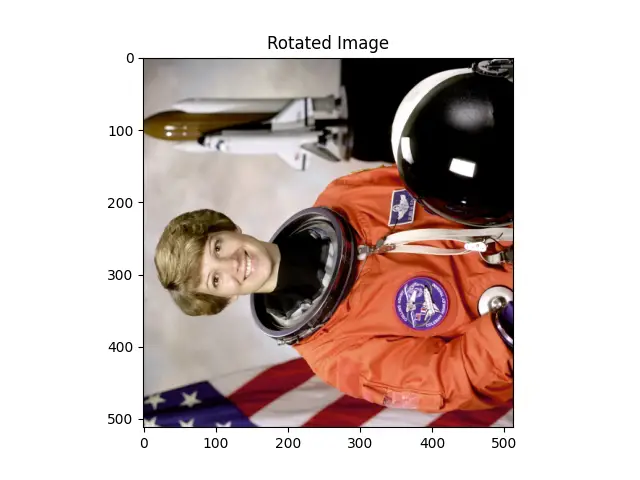
Example 6: Advanced Axis Manipulation
For our final example, we will explore more advanced axis manipulation by rotating a complex 4D array that could represent time-series data or sequences of images.
import numpy as np
original_4d_array = np.random.rand(10, 3, 3, 3)
rotated_4d_array = np.rot90(original_4d_array, axes=(2, 3))
print('Original 4D array:')
print(original_4d_array[0])
print('\nRotated 4D array:')
print(rotated_4d_array[0])
Conclusion
Through these examples, we showcased the versatility and power of numpy.rot90() in manipulating array orientations across various dimensions. Understanding how to apply this function effectively can enhance your data manipulation and analysis capabilities in Python.