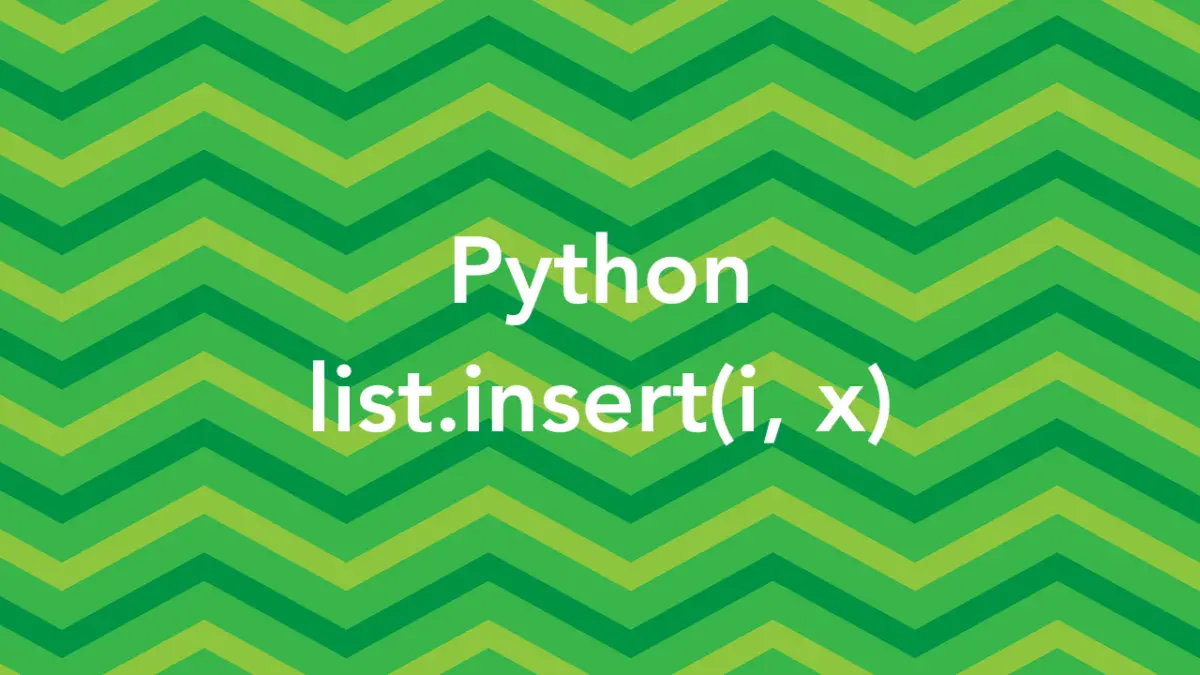
Overview
In Python, the list.insert()
method inserts an element at a given position in a list. Below is its syntax:
list.insert(i, x)
Parameters:
i
: The index of the element before which to insert, and can be any integer. If it is negative, it counts from the end of the list. If it is larger than the length of the list, it inserts the element at the end of the listx
: The element to insert. It can be any object, such as a string, a number, another list, etc. If it is an iterable, such as a list or a tuple, it will insert the whole iterable as a single element (you will understand this better in the examples to come).
The list.insert()
method does not return anything. It modifies the original list in place.
The performance of the list.insert()
method depends on the size of the list and the position where you insert the element. Its time complexity is O(n)
. That means it takes O(n)
time in the worst case, where n is the length of the list, because it has to shift all the elements after the insertion point to the right.
Now, it’s time for the interesting part: code and examples of using the list.insert()
method in practice.
Examples
Inserting a single element at a specific position in a list
The code:
# a list of video games
games = [
"Minecraft",
"Fortnite",
"CS:GO"
]
# Insert a game at index 2
games.insert(2, "League of Legends")
print(games)
Output:
['Minecraft', 'Fortnite', 'League of Legends', 'CS:GO']
Inserting an iterable as a single element in a list
This example demonstrates inserting a tuple into another list in Python:
my_list = [
"Sling Academy",
"Python",
"Programming"
]
my_list.insert(1, (2023, 2024, 2025))
print(my_list)
Output:
['Sling Academy', (2023, 2024, 2025), 'Python', 'Programming']
Inserting an element at the end or the beginning of a list
The code:
my_list = ["one", "two", "three"]
# Insert "zero" to the beginning of the list
my_list.insert(0, "zero")
# Insert "four" to the end of the list
my_list.insert(len(my_list), "four")
print(my_list)
Output:
['zero', 'one', 'two', 'three', 'four']