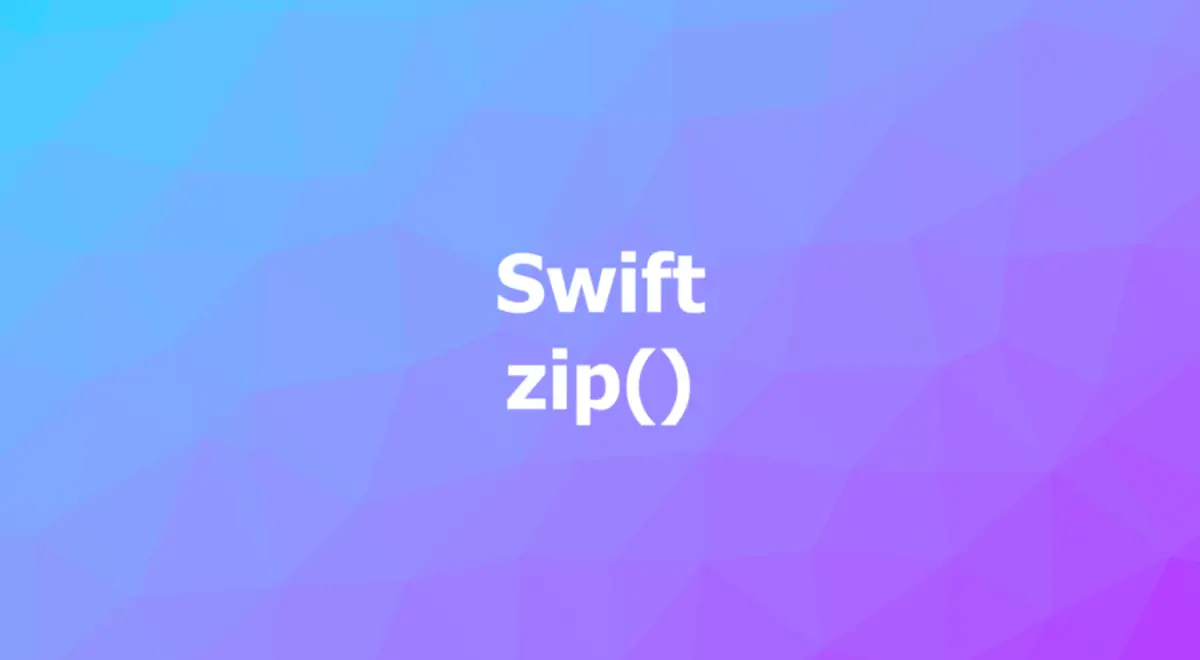
Overview
In Swift, the zip()
function is used to create a sequence of pairs built out of two underlying sequences, where the elements of each pair are the corresponding elements of each sequence. If the two input sequences have different lengths, it will use the shorter one as the limit.
Syntax:
func zip<Sequence1, Sequence2>(
_ sequence1: Sequence1,
_ sequence2: Sequence2
) -> Zip2Sequence<Sequence1, Sequence2>
where Sequence1 : Sequence, Sequence2 : Sequence
Where:
sequence1
: The first sequence or collection to zip. This can be any type that conforms to theSequence
protocol, such as an array, a range, a string, etc.sequence2
: The second sequence or collection to zip. This can be any type that conforms to theSequence
protocol, such as an array, a range, a string, etc.
The zip()
function returns a value of type Zip2Sequence
, which is a special type that conforms to the Sequence
protocol and stores both sequences internally. This type can be used in a for-in
loop or with other methods that work with sequences.
Examples
Learning by example is one of the best ways to get a deep understanding of the zip()
function.
Comparing two arrays of scores
A common use case of the zip()
function is to iterate over two sequences at the same time and perform some operation on their corresponding elements. For example, you are a teacher, and you have two arrays containing the scores of students on two recent tests. What you want is the average score of each student.
let scores1 = [80, 90, 75, 85]
let scores2 = [85, 95, 70, 80]
for (score1, score2) in zip(scores1, scores2) {
let averageScore = (score1 + score2) / 2
print("Average: \(averageScore)")
}
Output:
Average: 82
Average: 92
Average: 72
Average: 82
Creating a new sequence by combining two sequences
In this example, we have two arrays. The first array contains the names of some boys. The second array contains the names of some girls. What we will do is use the zip()
function to create an array of tuples where each tuple contains a couple of a boy and a girl.
let boys = ["Boy 1", "Boy 2", "Boy 3"]
let girls = ["Girl 1", "Girl 2", "Girl 3"]
let couples = Array(zip(boys, girls))
print(couples)
Output:
[("Boy 1", "Girl 1"), ("Boy 2", "Girl 2"), ("Boy 3", "Girl 3")]