When building backend applications with FastAPI, you may need to get the IP addresses of your users for several purposes, such as:
- Geolocation: With an IP address, you can determine the user’s location, which can be useful for providing location-specific content or services.
- Security: By logging IP addresses, you can detect and prevent unauthorized access to your application or website. You can also use IP addresses to identify potential security threats or suspicious activity.
- Analytics: Knowing the IP address of your users can help you track and analyze traffic to your application or website, including user behavior and preferences.
This quick article shows you a couple of different ways to get the IP address of a user with FastAPI.
Using Request Client Host
Example:
from fastapi import FastAPI, Request
app = FastAPI()
@app.get("/")
async def read_root(request: Request):
client_host = request.client.host
return {"client_host": client_host}
If your FastAPI project is running in localhost, the IP will be 127.0.0.1:
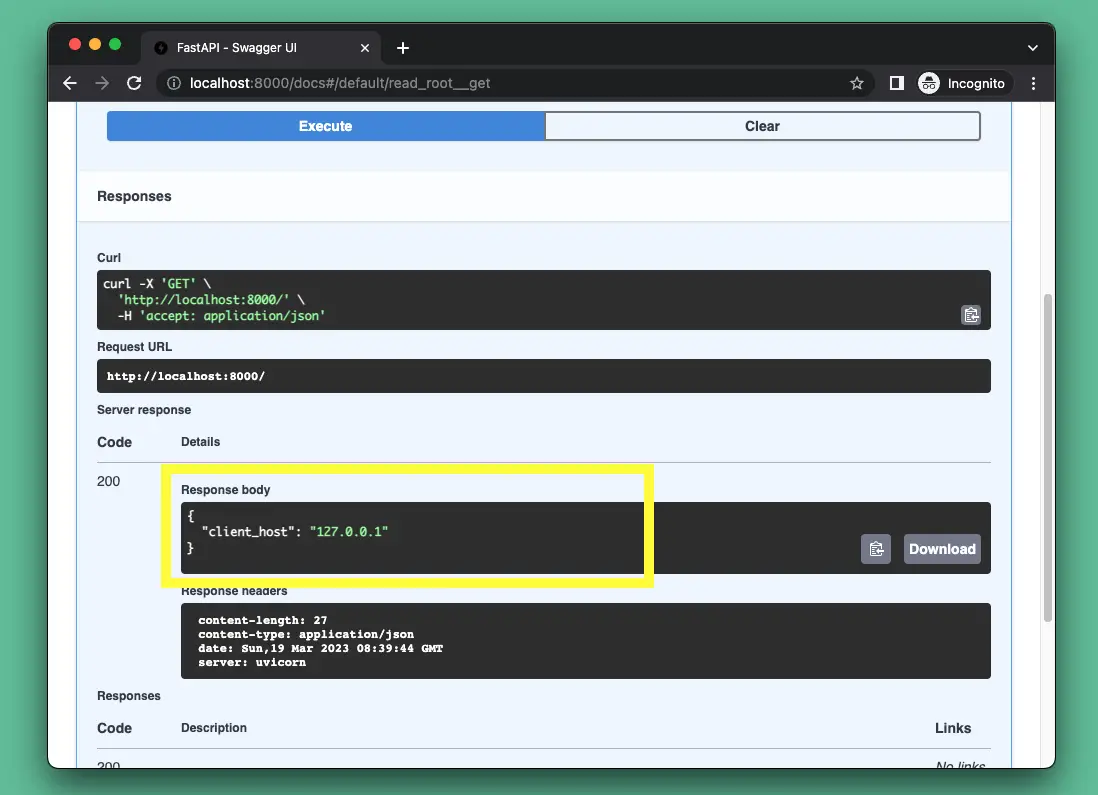
request.client will work well in the vast majority of cases unless you’re running behind a proxy. In that situation, you use uvicorn’s –proxy-headers flag to accept these incoming headers and make sure the proxy forwards them.
Using headers
You can use Header dependencies to access the X-Forwarded-For and X-Real-IP headers like so:
from fastapi import FastAPI, Depends, Header
app = FastAPI()
@app.get('/')
def home_route(
x_forwarded_for: str = Header(None, alias='X-Forwarded-For'),
x_real_ip: str = Header(None, alias='X-Real-IP')
):
return {"X-Forwarded-For": x_forwarded_for, "X-Real-Ip": x_real_ip}
The disadvantage of this approach is that these headers are not always reliable and can be spoofed by malicious clients. The result can also be null if the client is not sending the headers in the request.
Using information provided by Cloudflare
If your FastAPI application uses Cloudflare then you can easily get information about a user’s IP address, city, and country using the following headers: CF-Connecting-IP, CF-IPCity, and CF-IPCountry, respectively.
Example:
from fastapi import FastAPI, Request
app = FastAPI()
@app.get("/")
async def read_root(request: Request):
# Get user ip, city and country provided by Cloudflare
user_ip = request.headers.get('CF-Connecting-IP')
city = request.headers.get('CF-IPCity')
country = request.headers.get('CF-IPCountry')
This data is provided by Cloudflare for all plans, including the free one. Note that In some rare cases, information about a user’s city or country may be missing.