This concise and straight-to-the-point article walks you through different ways to make comments in JavaScript.
Line Comment
A line comment starts with two forward slashes // and ends at the end of the line. Line comments are used to add short explanations or temporarily disable a single line of code.
Example:
// Declare a variable named x and assigns it the value 100
const x = 100;
// The code below will not work because x is a constant
// console.log(x);
Block Comment
There might be cases where you want to comment out a section of code (such as an entire function or class) or just want to provide long explanations. This is a good time to use block comments.
Block comments start with /* and end with */. They can span multiple lines.
Example:
/*
This is a long comment
that spans multiple lines
*/
/*
const myFunction = () => {
console.log('Hello World');
};
*/
Docs Comment
Docs comments are a special type of comment used to generate documentation for code. They use a specific format that starts with /** and ends with */, and typically include information such as function parameters and return values.
Example:
/**
* This is a docs comment
* It includes information about a function
* @param {string} name - The name of the person to greet
* @returns {string} A greeting for the person
*/
const greet = (name) => {
return 'Hello, ' + name + '!';
};
Docs comments help IDEs (like VS Code) show useful suggesions when a developer hover their mouse over a function, a class, or something like that. The screenshot below clearly depicts what I mean:
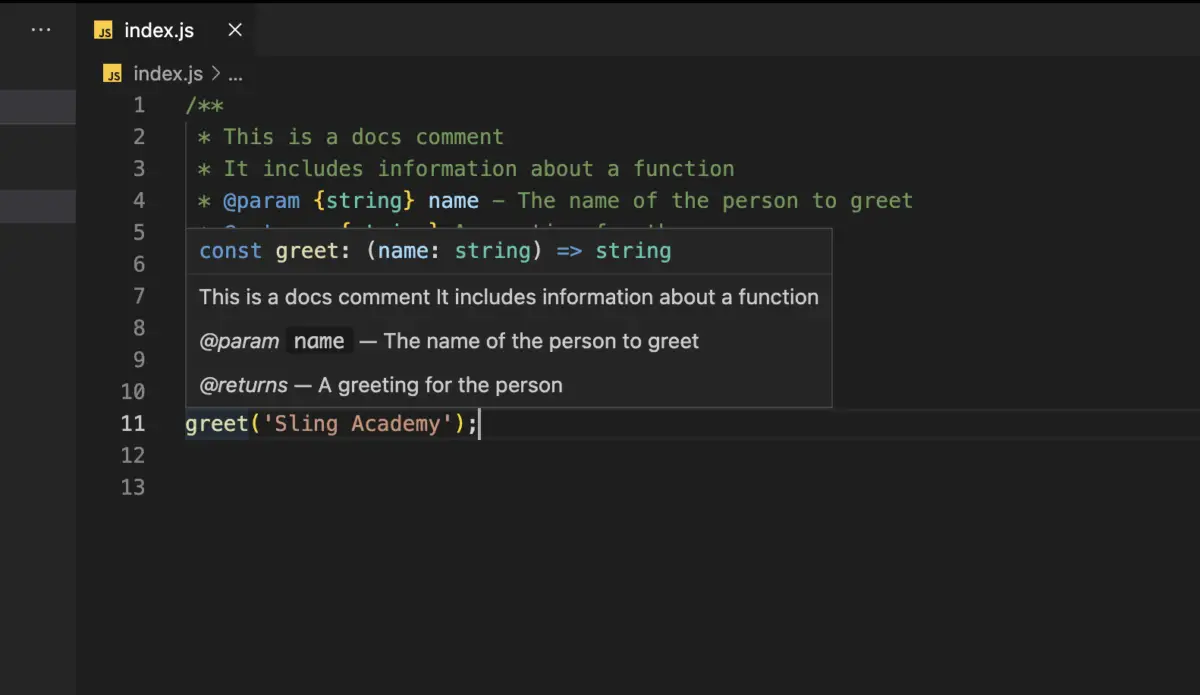
Using Shortcuts/Hotkeys
Most code editors and IDEs provide shortcuts to quickly add comments to your code. For example, in VS Code (Visual Studio Code), you can:
- Press Ctrl + / (Windows) or Command + / (macOS) to comment as well as uncomment a line of code (use your mouse to select that line)
- Press Shift + Alt + A (Windows) or Shift + Option + A (macOS) to add a block comment (use your mouse to select that block). If a block of code is commented out, you can uncomment it by using the same shortcut.
Demo:

That’s it. Happy coding!