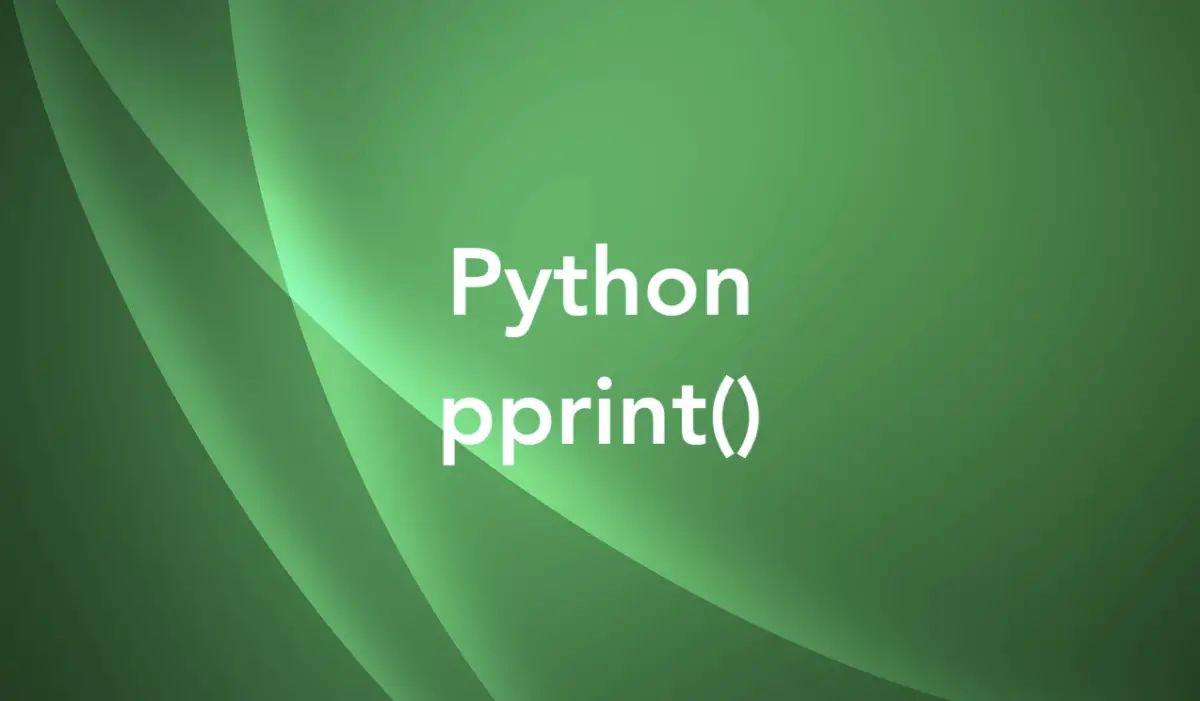
Overview
The pprint
module in Python provides a powerful pprint()
function whose name means “pretty print”. It is used to format and display complex data structures, such as dictionaries and lists, in a more readable and organized way. It is especially helpful when working with large and nested data structures.
Syntax:
pprint.pprint(object, stream=None, indent=1, width=80, depth=None, compact=False)
Where:
object
: This is the object or data structure that you want to pretty print.stream
: (Optional) This parameter specifies the output stream where the formatted output will be written. By default, it usessys.stdout
to print to the console.indent
: (Optional) It specifies the number of spaces used for indentation in the output. The default value is 1.width
: (Optional) This parameter determines the maximum width of the formatted output. If the output exceeds this width, it will be wrapped onto the next line. The default value is 80.depth
: (Optional) It sets the maximum depth of nested structures that will be printed. If the data structure exceeds this depth, it will be truncated and represented as ellipsis (…). The default value isNone
, which means the entire structure will be printed.compact
: (Optional) When set toTrue
, it removes unnecessary whitespace and indentation in the output, resulting in a more compact representation. The default value isFalse
.
The pprint()
function is part of the pprint
module, so don’t forget to import it before using it. You can use either import pprint
or from pprint import pprint
.
Examples
Some examples of utilizing the pprint()
function in practice.
Pretty print a list of dictionaries
The code:
from pprint import pprint
people = [
{
"name": "Mr. Turtle",
"age": 300,
"location": "Lake of Rot"
},
{
"name": "Ranni the Witch",
"age": 75,
"location": "The Forest"
},
{
"name": "Thanos",
"age": 1000,
"location": "Titan"
}
]
pprint(people)
Output:
[{'age': 300, 'location': 'Lake of Rot', 'name': 'Mr. Turtle'},
{'age': 75, 'location': 'The Forest', 'name': 'Ranni the Witch'},
{'age': 1000, 'location': 'Titan', 'name': 'Thanos'}]
Pretty print JSON
To use pprint()
with JSON data, you first need to parse the JSON string into a Python object using the json
module’s loads()
function. Once you have the Python object, you can pass it to pprint()
for beautiful printing.
Code example:
import pprint
import json
json_str = '{"name": "Mr. Wolf", "age": 35, "interests": ["music", "movies", "sports"], "children": [{"name": "Mary", "age": 5}, {"name": "John", "age": 7}]}'
data = json.loads(json_str)
pprint.pprint(data)
Output:
{'age': 35,
'children': [{'age': 5, 'name': 'Mary'}, {'age': 7, 'name': 'John'}],
'interests': ['music', 'movies', 'sports'],
'name': 'Mr. Wolf'}
Pretty print a class object
When it comes to a class object, pprint()
would not have direct access to the object’s attributes, and the output may not provide the desired level of detail and organization. Fortunately, we can overcome this by accessing the __dict__
attribute, which allows us to retrieve the object’s attributes and their values in a dictionary format.
Code example:
import pprint
class Person:
def __init__(self, name, age, address):
self.name = name
self.age = age
self.address = address
person = Person("Tazan", 50, "The Hidden Forest")
pprint.pprint(person.__dict__)
Output:
{'address': 'The Hidden Forest', 'age': 50, 'name': 'Tazan'}