This step-by-step guide shows you how to write your first Restful API with FastAPI: the Hello World project. Without any further ado, let’s get started.
Set Up Environment
Create a new directory to host the example project:
mkdir sling-academy
You can replace sling-academy with another name if you like.
Navigate into the newly created folder then initialize a Python virtual environment by executing the following command:
python3.11 -m venv
Active the virtual environment with this command:
source env/bin/activate
Note: If you aren’t familiar with Python virtual environment, see the detailed guide here.
Install Packages
Besides FastAPI, we need to use another package called uvicorn. Uvicorn is a web server implementation for Python that can help us launch our backend so that it can be accessed from web browsers or any HTTP client (Postman, Thunder Client, etc). Besides, uvicorn can also automatically reload the server when we change the code, making our working process faster and more efficient.
Within the activated environment from the previous step, run the following command:
pip install fastapi uvicorn
Write Code
In the project folder, create a new file named main.py. The project file structure now looks like this:
.
├── env
├── __pycache__
└── main.py
Add the following code to main.py:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def hello_world():
return {"success": True, "message": "Hello World"}
Code Explained
Firstly, we import FastAPI:
from fastapi import FastAPI
Then we Instantiate a FastAPI object:
app = FastAPI()
The next line is used to define a route:
@app.get("/")
Last but not least is the function that will be called when the route is accessed:
async def hello_world():
return {"success": True, "message": "Hello World"}
Run And Test
Start your API by running:
uvicorn main:app --reload
Where:
- main: The name of our entry file main.py without extension .py
- app: The app object in main.py
- –reload: This flag will help our server to automatically refresh when the code changes
Now you will see the following output in your terminal window:

In your web browser, go to http://localhost:8000 or http://127.0.0.1:8000. You would see this:

The response body is in JSON format.
If you access a route that doesn’t exist, the response will be:
{"detail":"Not Found"}
Screenshot:
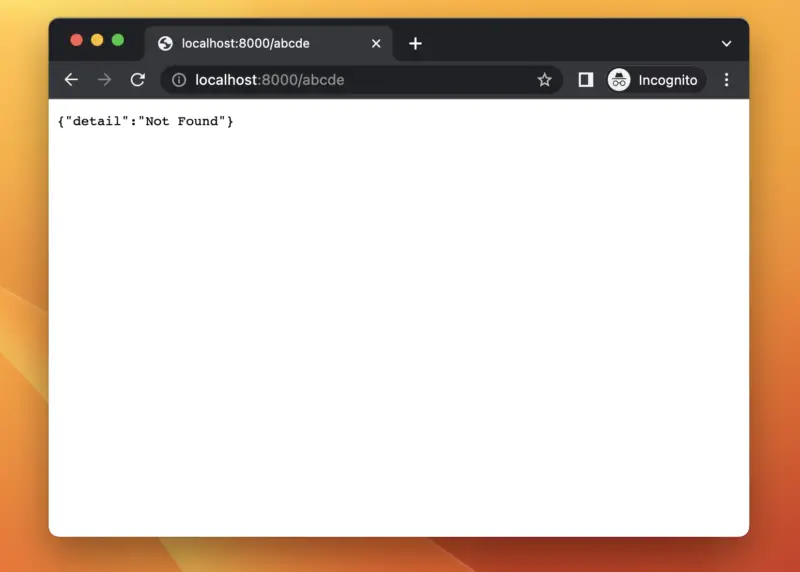
An awesome feature of FastAPI is the interactive documentation that is automatically generated. Go to http://localhost:8000/docs in your web browser and you will see all information about your routes:
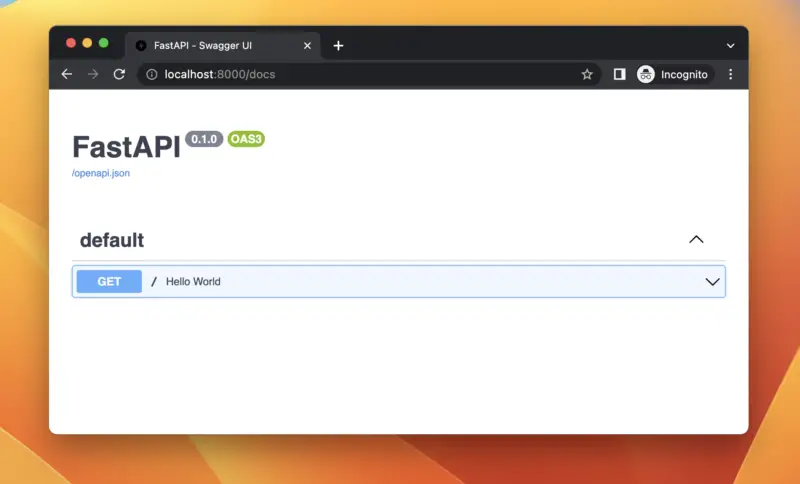
To stop the web server, just press Ctrl + C.
You can add other routes if you want:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def hello_world():
return {"success": True, "message": "Hello World"}
@app.get('/users')
async def get_users():
return {"success": True, "users": ["John", "Jane", "Sling Academy"]}
Congratulation! You’ve successfully created your first backend API with FastAPI. The amount of code is surprisingly small. If you have any questions, please leave a comment. Happy coding!