In SwiftUI, line separators are shown by default in List views. They help to visually distinguish each row and indicate the boundaries of each interaction area. However, not all List views need to have line separators. Sometimes, you may want to remove them for aesthetic reasons or to create a custom layout. This article will show you how to achieve that goal.
What is the point?
What you need to do is to use the listRowSeparator(_:) modifier on each row of your List and pass .hidden as the argument like this:
List {
ForEach(items) { item in
Text(item.name).listRowSeparator(.hidden)
}
}
Complete Example
Screenshot:
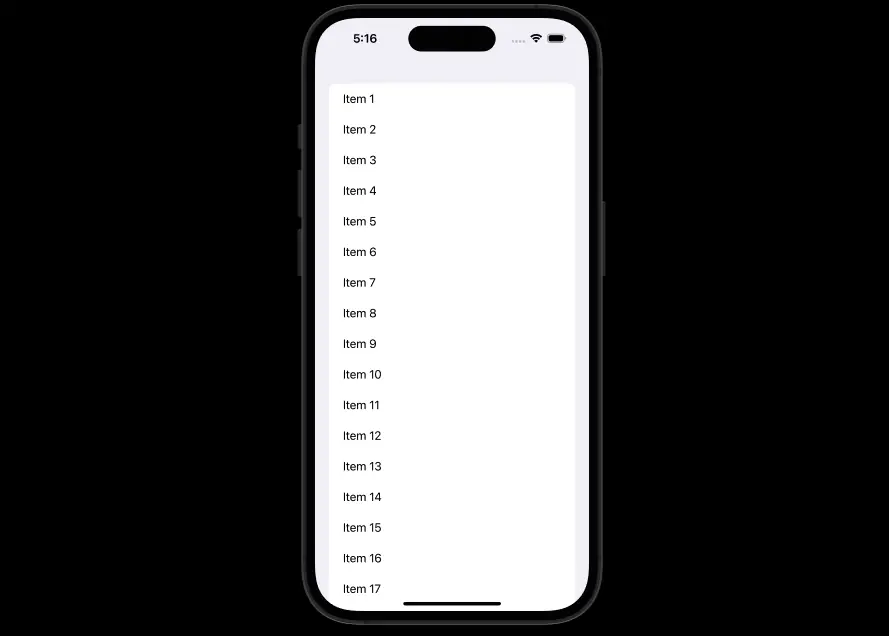
The full source code:
//
// ListNoSeparators.swift
// Examples
//
// Created by Sling Academy on 19/04/2023.
//
import SwiftUI
struct Item: Identifiable {
var id = UUID()
var name: String
}
struct ListNoSeparators: View {
// Generate 100 dummy items
let items = (1...100).map { i in
// Return a new item with the name "Item i"
Item(name: "Item \(i)")
}
var body: some View {
// implement the List
List {
ForEach(items) { item in
Text(item.name).listRowSeparator(.hidden)
}
}
}
}
struct ListNoSeparators_Previews: PreviewProvider {
static var previews: some View {
ListNoSeparators()
}
}
This concise tutorial ends here. Happy coding!