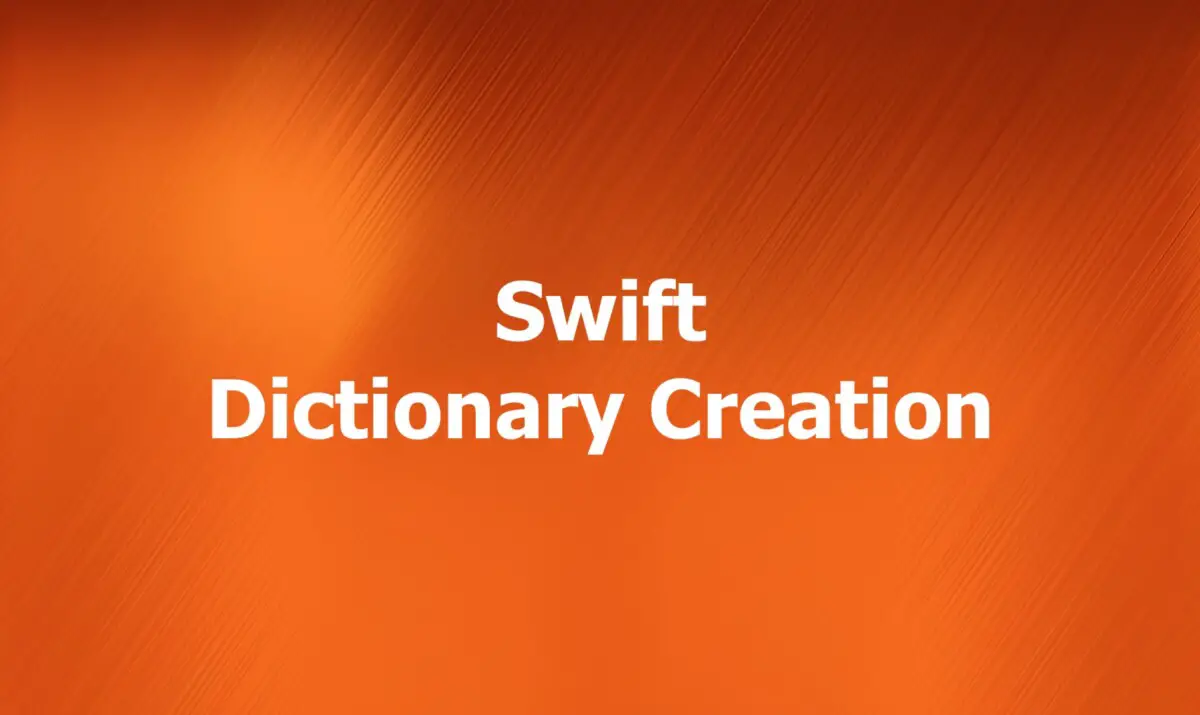
Introduction
One of the essential data structures in modern Swift programming is the dictionary. Dictionaries are unordered collections of key-value pairs, where each key maps to a specific value. They provide an efficient way to store and retrieve data, making them a fundamental part of many applications. In this article, we’ll explore several different approaches to creating dictionaries in Swift.
Using a dictionary literal
Non-empty dictionary
The simplest and most intuitive way to create a dictionary in Swift is by using a dictionary literal. A dictionary literal is a list of key-value pairs enclosed in square brackets and separated by colons.
Example:
var products = [
"notebook": 10.0,
"pen": 0.99,
"pencil": 0.5,
"eraser": 0.25,
"ruler": 1.0,
]
print(products)
Output:
["eraser": 0.25, "ruler": 1.0, "pen": 0.99, "pencil": 0.5, "notebook": 10.0]
In this example, we’ve created a dictionary called products
, which maps the names of some office equipment to their respective pricing.
Empty dictionary
In case you want to initialize an empty dictionary by using a dictionary literal, you need to provide the type information of the keys and values explicitly like so:
// create an empty dictionary
var emptyDict: [String: String] = [:]
// add a new key-value pair
emptyDict["first"] = "one"
Using the Dictionary initializer
Non-empty dictionary
You can create a dictionary by using the Dictionary
initializer when you have a sequence of key-value pairs, such as an array of tuples.
Example:
let pairs = [("a", 1), ("b", 2), ("c", 3)]
let dict = Dictionary(uniqueKeysWithValues: pairs)
print(dict) // ["a": 1, "c": 3, "b": 2]
This creates a dictionary named dict
with keys and values of type String
and Int
respectively. The initializer assumes that the keys are unique and throws a runtime error if there are duplicate keys.
If you have duplicate keys in your sequence and you want to combine the values for each key, you can use uniquingKeysWith
as shown below:
let pairs = [("a", 1), ("b", 2), ("c", 3), ("a", 4), ("b", 5)]
let dict = Dictionary(pairs, uniquingKeysWith: +)
print(dict) // ["a": 5, "c": 3, "b": 7]
The zip()
function can also be used to create a dictionary in Swift. This method is particularly useful when you have two separate arrays for keys and values.
Example:
let fruitNames = ["apple", "banana", "orange"]
let quantities = [5, 8, 10]
let fruits = Dictionary(
uniqueKeysWithValues: zip(fruitNames, quantities)
)
print(fruits) // ["orange": 10, "apple": 5, "banana": 8]
Empty dictionary
The syntax to create an empty dictionary by using the Dictionary
initializer is as follows:
var myDictionary = Dictionary<String, String>()
Creating a dictionary from an array of objects
In the following example, we will use the reduce(into: [:])
method to produce a dictionary from a given array of objects.:
struct Website {
let name: String
let url: String
}
let websiteObjects = [
Website(name: "Sling Academy", url: "https://www.slingacademy.com"),
Website(name: "Example", url: "https://www.example.com"),
Website(
name: "Sling Academy Public API",
url: "https://api.slingacademy.com"
),
]
let websites = websiteObjects.reduce(into: [:]) { dict, website in
dict[website.name] = website.url
}
print(websites)
Output:
[
"Example": "https://www.example.com",
"Sling Academy Public API": "https://api.slingacademy.com",
"Sling Academy": "https://www.slingacademy.com"
]