This short and straight-to-the-point article shows you a couple of different ways to comment out a single line or a block of code in Swift.
Table of Contents
Line Comment
A line comment begins with two forward slashes // and continues until the end of the line. Line comments are used to provide a brief explanation of the code on the same line.
Example:
// This code prints the sum of two numbers
let a = 10
let b = 20
print(a + b)
Block Comment
A block comment begins with a forward slash followed by an asterisk /* and ends with an asterisk followed by a forward slash */. Block comments are used to temporarily disable multiple lines of code at once or to show a long description.
Example:
/*
This function calculates the sum of two numbers.
To use it, call the function with two integer arguments.
*/
func sum(_ a: Int, _ b: Int) -> Int {
return a + b
}
// func subtract(_ a: Int, _ b: Int) -> Int {
// return a - b
// }
Docs Comment
A docs comment begins with three forward slashes /// and is used to generate documentation for the code. Docs comments include information about the purpose of the code, its inputs and outputs, and any special considerations.
Example:
/// This function calculates the sum of two numbers.
/// - Parameters:
/// - a: The first number to add.
/// - b: The second number to add.
/// - Returns: The sum of the two numbers.
func sum(_ a: Int, _ b: Int) -> Int {
return a + b
}
Docs comments help your IDE (Xcode, VS Code, etc.) show suggestions when you hover your mouse over a function or a class as shown below:
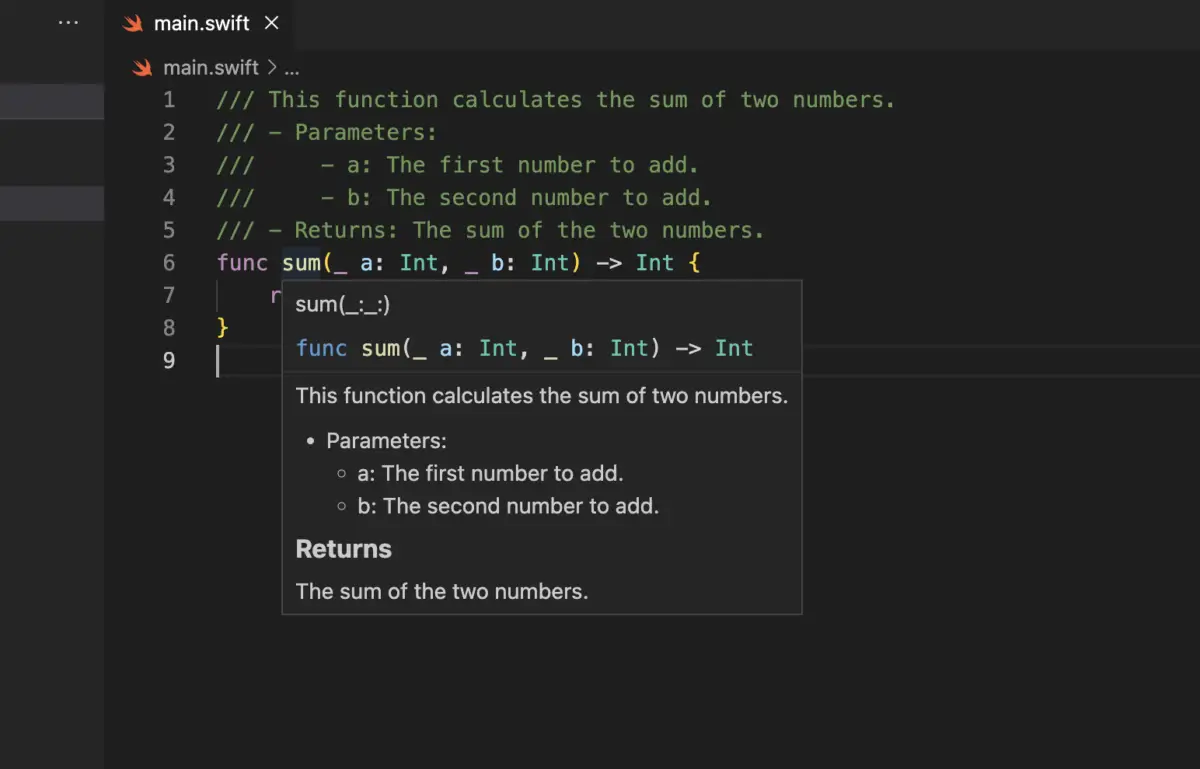
Using Shortcuts/Hotkeys
Modern IDEs like Xcode and VS Code (Visual Studio Code) support shortcuts for conveniently adding comments.
- To insert a line comment, use the shortcut Command + / on Mac or Control + / on Windows. To uncomment a line of code, just do the same
- To comment out a block of code, use your mouse to select (bold) it, then press Command + / on Mac or Control + / on Windows. To remove the comment and make this block of code working again (uncomment), you can use the same shortcuts.
A quick demo is worth more than a thousand of words:
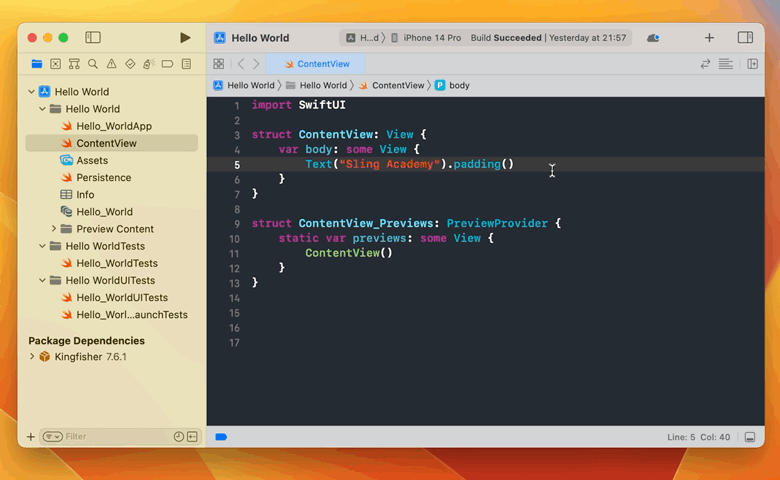
Hope this article is useful for you. Happy coding and have a nice day!