This concise, straight-to-the-point article will walk you through a couple of different solutions to fix a common error you might encounter when working with FastAPI.
The Problem
Here is the error message:
AttributeError: 'dict' object has no attribute 'encode'
The most likely reason for this error is that you used the Response
class from FastAPI to return a dictionary as the response data. The Response
class expects a string or bytes object as the input, and it tries to call the encode()
method on it. However, if you pass a dictionary instead, it will raise the AttributeError
.
The error message, AttributeError: ‘dict’ object has no attribute ‘encode’
, means that, behind the scene, the encode()
method is called on a dictionary object (which does not have such a method). The encode()
method is defined for string objects, and it converts them into bytes objects using a specified encoding.
The following code snippet looks pretty nice, but it will cause the mentioned issue if you make a GET request to the root route:
from fastapi import FastAPI, Response
app = FastAPI()
@app.get("/")
def root():
data = {
"message": "Welcome to Sling Academy!",
"a_random_number": 123
}
return Response(status_code=200, content=data)
Don’t worry. There are some solutions that can help you get rid of the problem.
Using the JSONResponse class
Instead of using the Response
class, you can use the JSONResponse
class to return dictionaries. This class will automatically serialize the dictionary into JSON format and set the appropriate headers.
Example:
from fastapi import FastAPI
from fastapi.responses import JSONResponse
app = FastAPI()
@app.get("/")
def root():
data = {"message": "Welcome to Sling Academy!", "a_random_number": 123}
return JSONResponse(status_code=200, content=data)
It works like a charm. You can test it with the built-in Swagger UI docs:
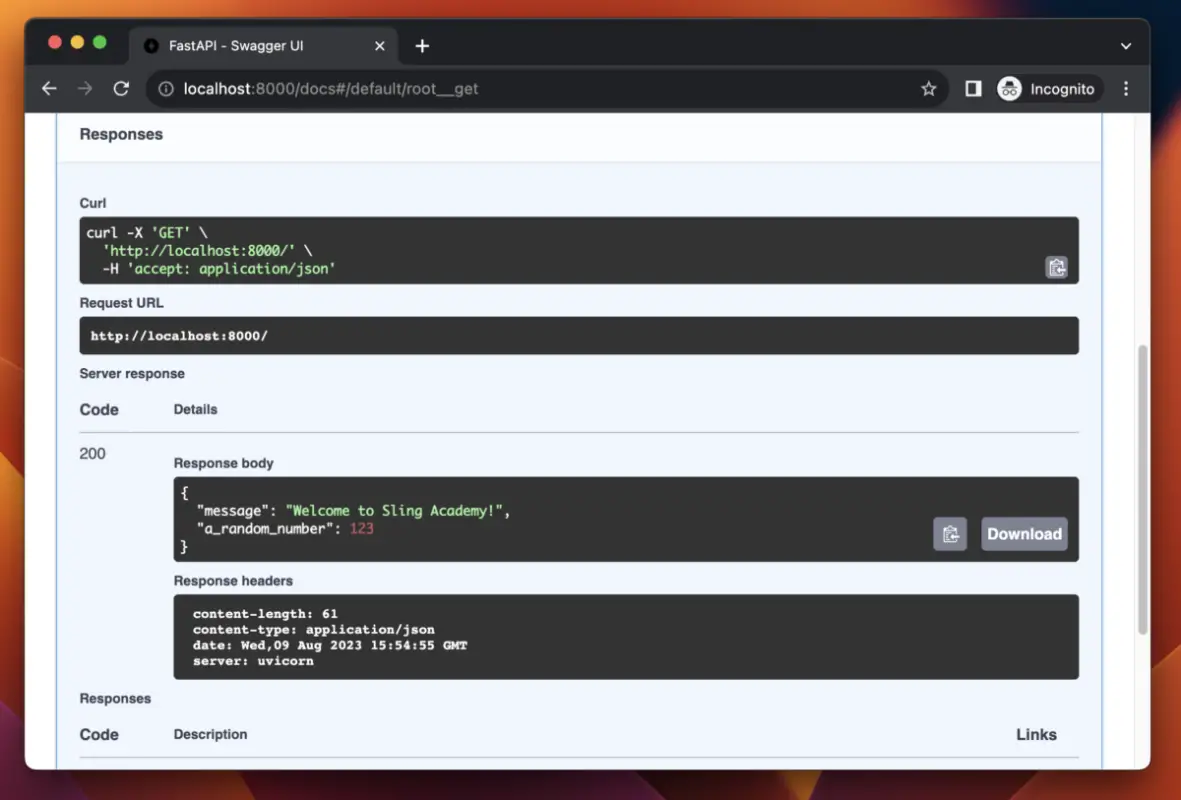
Convert the dictionary into a JSON string
If you insist on using the Response
class, you convert the dictionary into a JSON string using the json.dumps()
function. This will create a valid string that can be encoded using the encode()
method.
Example:
from fastapi import FastAPI, Response
import json
app = FastAPI()
@app.get("/")
def root():
data = {"message": "Welcome to Sling Academy!", "a_random_number": 123}
json_data = json.dumps(data)
return Response(status_code=200, content=json_data)
That’s it. Happy coding, make the bug go away, then enjoy your day!