This article walks you through a step-by-step guide to generating an XML Sitemap with FastAPI. Without any further ado, let’s get started.
What is an XML Sitemap?
An XML Sitemap is an XML file that contains a list of URLs on your website. It helps search engine crawlers index and rank your website’s pages. It also provides a convenient way for users to find the most important and latest pages on your website.
The structure of an XML Sitemap is quite simple. It consists of two parts: the root tag and the URLs. The root tag is the opening and closing tag that contain the XML sitemap information. The URLs are the actual URLs on your website that you want to be indexed by search engines. The XML sitemap also contains optional information about each URL, such as the date it was last modified or the priority of the URL.
Showcase: You can see a production-level XML sitemap at https://api.slingacademy.com/v1/examples/sitemap.xml
Returning XML Response in FastAPI
By default, FastAPI returns JSON data. However, you can make it returns XML response as needed. What you need to do is to import Response from fastapi and set media_type=”application/xml” like this:
return Response(content=your_content, media_type="application/xml")
For more clarity and completeness, see the final code at the end of this article.
Full Example
Generating an XML Sitemap with FastAPI is a quick and easy process. Just follow the steps listed below, and you’ll get the job done without any headaches.
Step 1: Add a Route that Serves the Sitemap
The code snippet below produces a route that returns XML content:
from fastapi import FastAPI, Response
app = FastAPI()
@app.get("/sitemap.xml")
async def get_sitemap():
my_sitemap = """<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">"""
my_sitemap += """</urlset>"""
return Response(content=my_sitemap, media_type="application/xml")
Run it with uvicorn like so:
uvicorn main:app --reload
Now go to http://localhost:8000/sitemap.xml with a web browser, and you will see a plain XML page like this:
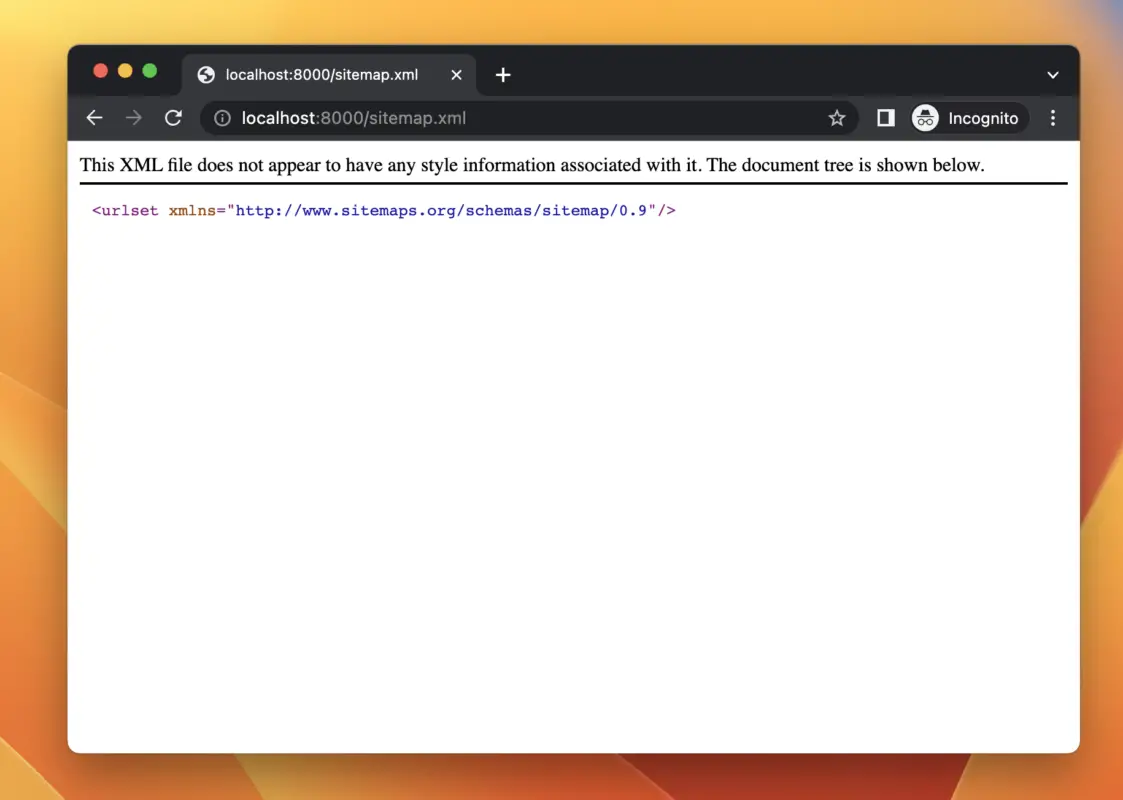
Even though it contains almost nothing, it has a valid XML schema.
Step 2: Adding URLs
For our XML sitemap to really make sense, it needs to contain a list of URLs. These URLs can be fetched from your database or somewhere else.
Continue to our example by modifying the code snippet in the previous section like this:
# main.py
from fastapi import FastAPI, Response
app = FastAPI()
@app.get("/sitemap.xml")
async def get_sitemap():
my_sitemap = """<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">"""
# Fetch your urls from database or somewhere else
# Then add to the urls array
urls = []
for i in range(100):
urls.append(f"""<url>
<loc>https://www.slingacademy.com/examples/article-{i}</loc>
<lastmod>2023-07-27T19:34:00+01:00</lastmod>
<changefreq>weekly</changefreq>
<priority>0.8</priority>
</url>""")
my_sitemap += "\n".join(urls)
my_sitemap += """</urlset>"""
return Response(content=my_sitemap, media_type="application/xml")
After saving the code and refreshing the browser, you’ll get an actual XML sitemap like this:
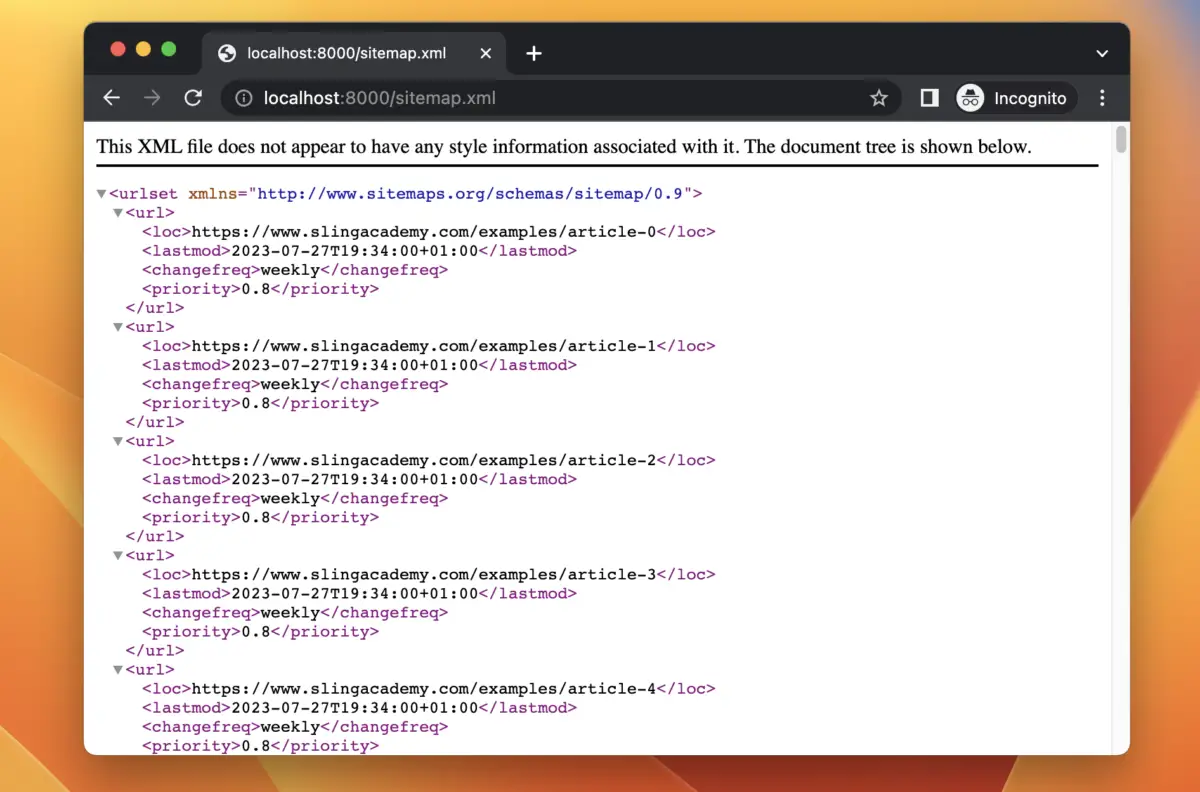
You can also see our online demo here.
Well, there’s one more thing that might help you. It’s a website that can have one or multiple sitemaps. Each sitemap should contain only 1000 URLs. If your site is large, add more sitemaps as necessary.
Conclusion
Congratulations! You’ve successfully created an XML sitemap with FastAPI. Try to modify the code, make some changes, add some things, and remove some things to see what happens next. That’s the best way to learn to program.
If you find a bug or something to improve in the code in the example above, let us know by leaving comments. Good luck and have a nice day.