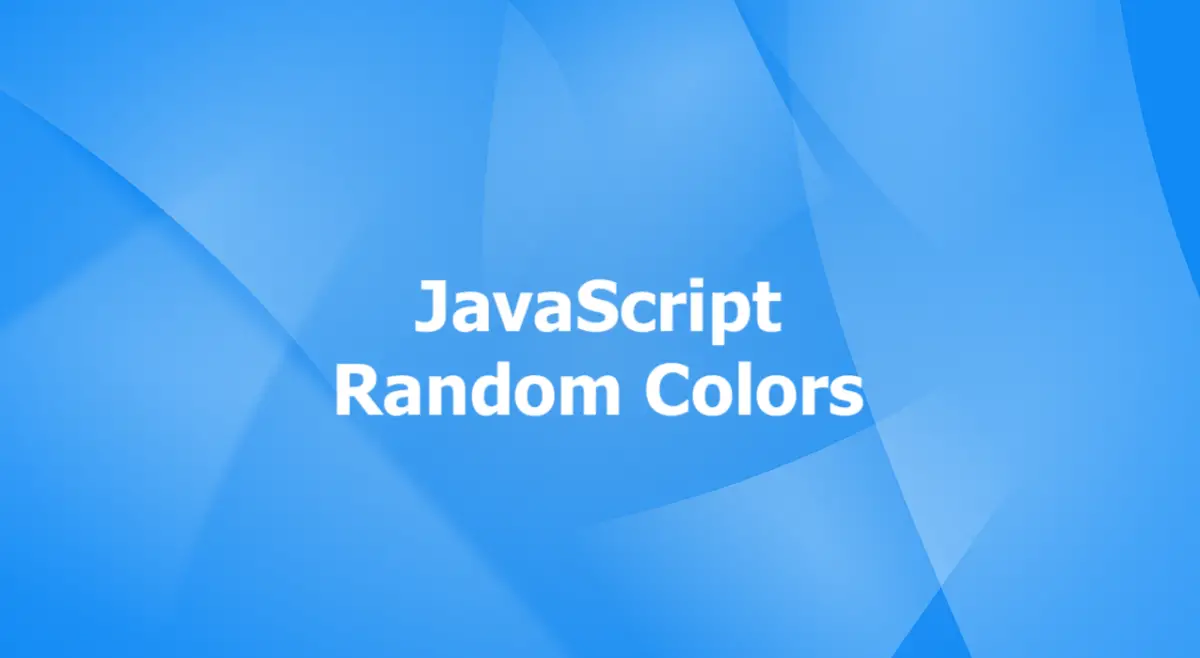
Introduction
When developing modern websites and web apps with JavaScript, there could be instances where you need to generate random colors, such as:
- You want to reduce boredom and increase the freshness of your websites
- To test or debug the appearance and functionality of web elements under different color schemes or contrasts.
This article will show you how to create random RGB colors, random hex (hexadecimal) colors, random HSL colors, and random HTML color names just by using vanilla JavaScript. No third-party libraries are necessary.
Generating random RGB color codes
An RGB color code is three numbers between 0 and 255 that represent the amount of red, green, and blue in a color: rgb(redValue, greenValue, blueValue). They are enclosed in parentheses and separated by commas.
To generate a random RGB color in JavaScript, you need to create three random integers between 0 and 255 using the Math.random() function. And then, you can concatenate them into a string with commas and parentheses.
Example:
// define a reusable function
const randomRgbColor = () => {
let r = Math.floor(Math.random() * 256); // Random between 0-255
let g = Math.floor(Math.random() * 256); // Random between 0-255
let b = Math.floor(Math.random() * 256); // Random between 0-255
return 'rgb(' + r + ',' + g + ',' + b + ')';
};
// Create some random colors
console.log(randomRgbColor());
console.log(randomRgbColor());
Output (note that the output is not consistent due to the randomness):
rgb(76,125,16)
rgb(99,208,133)
You can set a random background color for a div element like so:
const myDiv = document.querySelector("#my-div");
myDiv.style.backgroundColor = randomRgbColor();
Generating random hex color codes
A hex color code is a six-digit code that starts with a # and represents the amount of red, green, and blue in a color. Each digit can be from 0 to F (or 15 in decimal).
The solution to creating a random hex color code is to use the Math.random() function to generate random numbers between 0 and 15 and then convert them to hexadecimal using the toString(16) method. You can also use a loop or an array to concatenate the characters into a string.
Example:
const getRandomHexColor = () => {
// Define an array of hexadecimal digits
const hexChars = [
'0',
'1',
'2',
'3',
'4',
'5',
'6',
'7',
'8',
'9',
'A',
'B',
'C',
'D',
'E',
'F',
];
// Generate an array of six random indices from 0 to 15
const hexIndices = Array.from({ length: 6 }, () =>
Math.floor(Math.random() * 16)
);
// Map each index to its corresponding hex digit and join them into a string
const hexCode = hexIndices.map((i) => hexChars[i]).join('');
// Return the string with a "#" prefix
return `#${hexCode}`;
};
// Try it out
console.log(getRandomHexColor());
console.log(getRandomHexColor());
Output:
#9B5C4E
#75C55E
Your output might be different from mine. As always, the reason is the randomness.
Generating random HSL color codes
To generate random HSL color codes, you can create a function that returns a string of three numbers that represent the hue, saturation, and lightness of a color. The hue is a number between 0 and 360 degrees, while the saturation and lightness are percentages between 0 and 100. You can use the Math.random() function to generate random numbers within these ranges and then use template literals to format them into a string.
Example:
const getRandomHslColor = () => {
// Define an async function that returns a random number within a range
const getRandomNumber = (min, max) =>
Math.round(Math.random() * (max - min) + min);
// Destructure an object that contains three random numbers for hue, saturation and lightness
const { hue, saturation, lightness } = {
hue: getRandomNumber(0, 360),
saturation: getRandomNumber(0, 100),
lightness: getRandomNumber(0, 100),
};
// Return the string with hsl prefix
return `hsl(${hue}, ${saturation}%, ${lightness}%)`;
};
// test our function
console.log(getRandomHslColor());
console.log(getRandomHslColor());
My output:
hsl(338, 17%, 0%)
script.js:18 hsl(324, 8%, 43%)
Get a random color name from an array of color names
HTML color names like red, blue, orange, and orangered are intuitive and easy to remember than color codes. You can create an array containing a limited number of different color names and then randomly pick a color from that array. The benefit of this approach is that the selected color will only be among the ones you have preset and will not produce unwanted results.
Example:
const randomColors = (colorArray) => {
const randomIndex = Math.floor(Math.random() * colorArray.length);
return colorArray[randomIndex];
};
// only these colors are allowed
const colors = ['pink', 'orange', 'yellow', 'purple', 'green'];
// get a random color from the array
console.log(randomColors(colors));
The result could be one of the five predefined color names.